Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial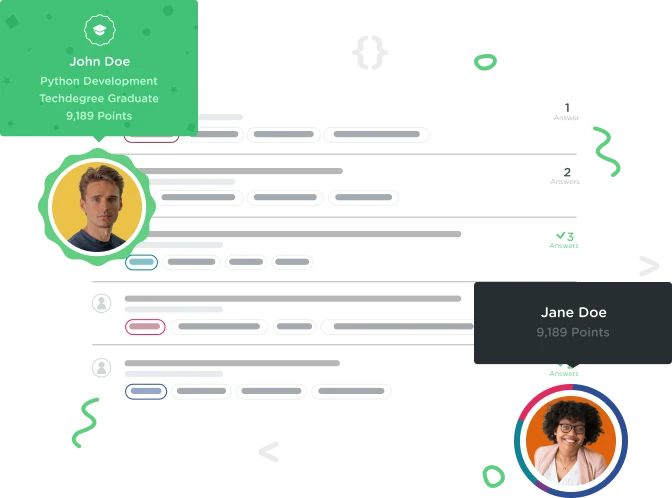

Juan Huttemann
7,464 Pointstodo_list.rb: " `method did not return the correct index." but I test my code and it works https://repl.it/EGOY
Sometimes I just hate this platform of tth :(
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
@todo_items.push(TodoItem.new(name))
end
def find_index(name)
if @todo_items.include? name
@todo_items.each_with_index do |item, index|
if item == name
return index
end
end
else
return nil
end
end
end
1 Answer
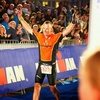
Steve Hunter
57,712 PointsHi Juan,
My solution to this isn't pretty - there must be a better way of doing it!
However, I took an each
loop to iterate through the todo_items
array. The loop escapes if the item.name
is the same as the parameter passed in - I escape the loop by returning the current value of index
. At each iteration, index
is incremented. If the loop finishes without the return
call then there was no match, so I return nil
.
def find_index(name)
index = 0
found = false
todo_items.each do |item|
if item.name == name
found = true
return index
end
index += 1
end
return nil
end
Your solution is similar, but you haven't compared item.name
to name
. The item
is an instance of the TodoItem
class - it won't (shouldn't) compare directly with the name
string, perhaps? Your code sets up todo_items
to be an array of strings. In the challenge it is an array which is a member variable of the TodoList
class which is populated by instances of the TodoItem
class. It is an array of TodoItem
instances, not strings.
I hope that helps,
Steve.