Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial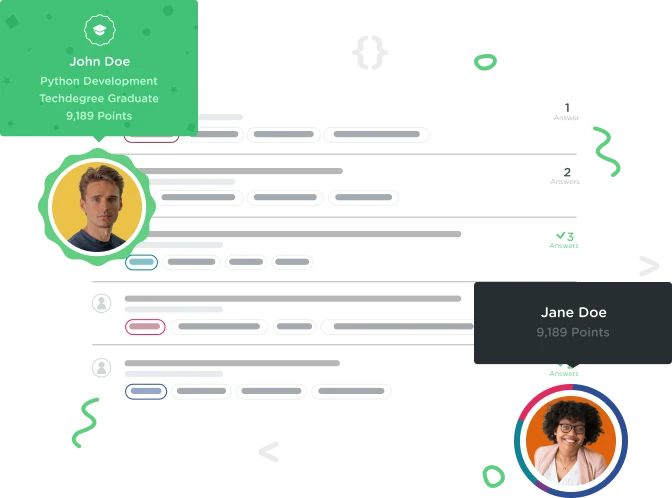
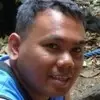
Dennis Amiel Domingo
17,813 PointsToggle sorting options with select and check boxes
Hi guys!
You can make sorting easier using a select box and a check box. Here's the code:
<?php
include 'list.php';
$filter = array();
$status = '';
$checkThis = '';
$selectPrio = '';
$selectDue = '';
$selectTitle = '';
$field = '';
if(isset($_POST['field'])) {
$field = $_POST['field'];
if ($field == null) {
echo 'Please select an option.';
} else {
switch ($field) {
case 'priority':
$selectPrio = 'selected';
break;
case 'due':
$selectDue = 'selected';
break;
case 'title':
$selectTitle = 'selected';
break;
}
}
}
if(isset($_POST['status'])) {
$status = 'all';
$checkThis = 'checked';
}
echo '<form action="index.php" method="post">';
echo '<p>Sort by</p>';
echo '<select name="field">';
echo '<option value="priority"' . "$selectPrio" . '>Priority</option>';
echo '<option value="due"' . "$selectDue" . '>Due Date</option>';
echo '<option value="title"' . "$selectTitle" . '>Title</option>';
echo '</select>';
echo '<br>';
echo '<input type="checkbox" name="status" value="Yes"' . "$checkThis" . '>Show completed tasks';
echo '<br>';
echo '<br>';
echo '<input type="submit" value="Submit">';
echo '</form>';
foreach ($list as $originalKey => $item) {
if ($status == 'all' || $item['complete'] == $status) {
if (isset($field) && isset($item[$field])) {
$filter[$originalKey] = $item[$field];
} else {
$filter[$originalKey] = $item['priority']+12;
}
}
}
asort($filter);
echo '<br>';
echo '<table>';
echo '<tr>';
echo '<th>Title</th>';
echo '<th>Priority</th>';
echo '<th>Due Date</th>';
echo '<th>Complete</th>';
echo '</tr>';
foreach($filter as $id => $value){
echo '<tr>';
echo '<td>' . $list[$id]['title'] . "</td>\n";
echo '<td>' . $list[$id]['priority'] . "</td>\n";
echo '<td>' . $list[$id]['due'] . "</td>\n";
echo '<td>';
if ($list[$id]['complete']){
echo 'Yes';
} else {
echo 'No';
}
echo "</td>\n";
echo '</tr>';
}
echo '</table>';
?>
1 Answer

Julian Helmholz
3,242 Pointsnice but propably too much for most students that do not know html well.
Jeremy Antoine
15,785 PointsJeremy Antoine
15,785 PointsThat's brilliant! Thank you for that!