Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial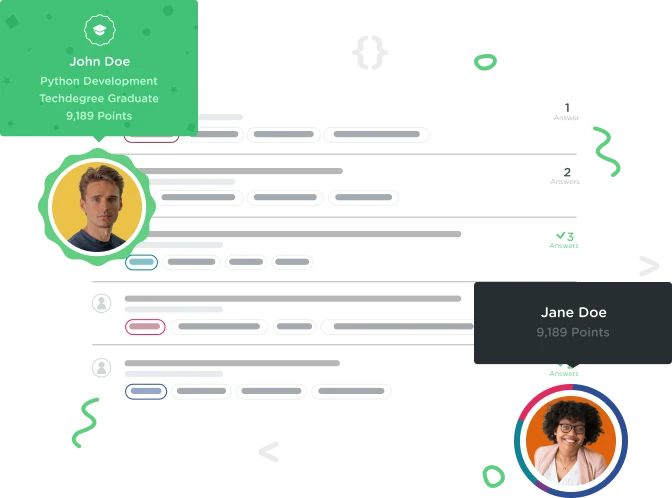
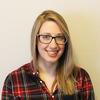
Ashley Boucher
Treehouse TeacherToken Properties Brainstorming Discussion
Share your notes, thoughts, and answers to brainstorming questions for Token Properties Brainstorming.
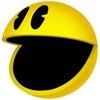
Richard Eldridge
8,229 PointsI guess it would be similar to the player differentiation. You could give it a color property and an id that corresponds to the player properties. On screen, the color property will allow the user to keep track of whose token is who's, and the id will keep track of which token corresponds to which player. If a token is on the board, then it has been played. Right? If the spot on the board is full, then there's a token in it. It's not really important to track individual tokens, I think, but rather, which spots are left on the board. Each player goes in turn until one player wins or the board is full and it's a draw. Nobody runs out of tokens.
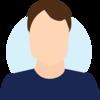
Mike Hatch
14,940 PointsI failed to come up with anything on this one. I typed out the basic structure:
class Token {
constructor() {
// this. = ??
// this. = ??
// this. = ??
}
}
.... and then drew a blank.

Félix Guérin
17,910 PointsProperties I think the Token class should have:
- color (derived from the Player color);
- player (associate the token to particular player with the player ID);
- played (boolean value);
- token id (to differentiate each token).

Piero Meza
2,285 Pointsclass Token{ constructor(id,color,player,status, position) } I think those can be the properties for constructor
17 Answers
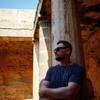
mersadajan
21,306 PointsI see no reason why the token should have anything more than a color property. The token is placed by the player so the method is in the player class. Its position will be determined by the board and it doesn't really need an ID. There are two players and both have a color that clearly determines who this token belongs to.

Sheila Anguiano
Full Stack JavaScript Techdegree Graduate 35,239 PointsI had similar ideas..
Properties: color, player, and array of tokens connected to the player
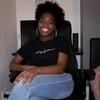
Kaleshe Alleyne-Vassel
8,466 PointsStruggled to think of what properties the token should have. I just attached the player to it where it can access the colour which is the only thing I think it important in this case.
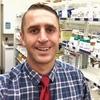
Kurt Pessa
16,763 PointsTwo interesting thoughts I had was:
- We could pass
player
object into Token constructor to have access toplayer.color
- Also, it felt like each time we instantiated a new token, we should push in on to that player's token array with
player.token.push(this)
?
Questions:
-
Type of Coordinate System - (x,y) vs id-based coordinate system
- For the board, I really wanted to use an (x,y) coordinate system for the board, but I've seen chess, snake, and other grid based games translated into 1-D with each position having a unique ID versus and (x,y) coordinate.
- Here's an example of a 1-d coordinate system.
-
row
ory
: A floor division would give which row of the board you're on -
col
orx
: A modulus would give which column. - For
(2,3)
and 7x7 grid,position = 17
I think bcMath.floor(17/7) = 2
forrow
and17%7 = 3
forcol
-
- Here's an example of a 2-d or
x,y
coordinate system. I've also seen a few options:- Using an
Object
-{ x : 2, y : 3 }
- Using an
Array
-[2,3]
- Using an
String
-"2,3"
with comma-separated value
- Using an
- Here's an example of a 1-d coordinate system.
- Still interested to see which direction this project takes!
- For the board, I really wanted to use an (x,y) coordinate system for the board, but I've seen chess, snake, and other grid based games translated into 1-D with each position having a unique ID versus and (x,y) coordinate.
-
ID - Do we really need an
id
for each token?- Wondering about when you would use the id for each token! Interested to see what way the project goes!
Class implementation
class Token {
constructor(id, player, played = false, coordinates={}) {
this.id = id;
this.color = player.color; // pass in player object, use player's color property to assign token's color property
this.coordinates = coordinates
player.tokens.push(this) // push token to player's token array each time a token is instantiated?
}
}
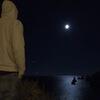
tapio
Full Stack JavaScript Techdegree Graduate 25,832 Pointsclass Token {
constructor(id, color, used=false){
this.id = id;
this.color;
this.used = used;
}}
Question: How can I make sure the ID matches the player ID? Maybe with Jeffrey's approach?

Rupertson Espinosa
4,128 PointsI believe that the ID of each token object created would be unique as they are created (like token 1, token 2, token 3, ... ). And the "color" attribute of Token is what matches with the "color" attribute of Player to determine which token belongs to which player.
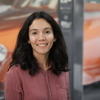
Berlin Galvan
7,145 PointsWe could use the following properties: color, an id set to which player id used it, and a boolean value to determine if it has been played.

James Nguyen
Courses Plus Student 6,841 Pointsclass token{ constructor(id, color, isPlay=false){ this.id= id; this.color= color; this.isPlay= isPlay }
}
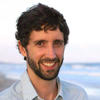
Brenden Seidel
16,890 Pointsclass Token {
constructor(
coordinates,
id,
player,
played = false
) {
this.coordinates = coordinates;
this.id = id;
this.player = player.id;
this.color = player.color;
this.played = played;
}
}

Mikel Cati
8,045 Points//REPRESENT THE TOKENS
class Tokens {
constructor(color, position) {
this.color = color;
this.position = position;
this._played = false;
this.id = null;
}
}
Based on the OOP course i thought maybe i could use a setter and getter to update if the token is played and by who.
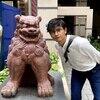
Carlos Chavez
5,691 Points/**
* Each token can be linked to the individual player id
* The color of each token corresponds to the color of the player
* the token class can have the following properties:
* - status (used/not used)
* - id
* - color
* - position
* knowing if a token has been used in the game can be done by comparing its position to the empty/filled spaces in the game board.
*/

James Croxford
12,723 PointsMy initial thoughts
class Token {
constructor (tokenColour, tokenID, playerID, boardLocation, usedToken = false) {
this.colour = tokenColour;
this.tokenID = tokenID;
this.assignedTo = playerID;
this.boardLocation = boardLocation;
this.usedToken = usedToken;
}
}
Utilise the playerID from the Player class as parameter to determine who the token is assigned to.

Joseph Bertino
Full Stack JavaScript Techdegree Student 14,652 Pointsclass Token {
constructor (playerid, color) {
this.playerid = playerid;
this.color = color;
}
}
How will you differentiate between token objects? How can you use the Player ID to create a token identifier?
- I wouldn't. I would just generate a new token every time a Player is about to take a turn. The player takes their turn, the token would then occupy a space on the game board, and that's it.
How will you keep track of which player an individual token belongs to?
- By its playerid, which be used to automatically determine the token's color
How will you know if a token has been played or not?
- If it exists, it's been played. I don't believe in creating a cache of Tokens for each player and tracking how many tokens were played so far.

Kylie Soderblom
3,890 PointsI'm wondering if all is needed is that the token has the color of the player who is active. set token (this.player.color){ this._tokenColor = this.player.color; } get token() { return this._tokenColor; } Nope - scratch that. I'm not quite there.
If the position of the token is to be handled in the board class, as well as the number of tokens available then what else does the token do? Ashley does create an array for tokens in her Player class. So, that would lead me to believe that I need to have a unique identifier for each token. the player chooses the column into which to drop the token, but the board determines how far down that token falls. (If there are already tokens in that column). So -
class Token{
constructor (TokenID, player.color, TokenColumn) {
this.TokenID = TokenID;
this.TokenColor = player.color;
this.TokenColumn = TokenColumn; // the board probably takes care of positions.
}
}
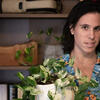
Chris Adams
Front End Web Development Techdegree Graduate 29,423 PointsMy thinking for the Token class object:
- It needs to be associated with a player or owner.
- It needs to be associated with a specific colour.
- It needs to be associated with a specific position on the board.
- It needs to have a status identifying whether the token has been used or not.
class Token {
constructor(ownerID, colour, position = null, tokenUsed = false) {
this.ownerID = ownerID; // to reference the ID of the player that owns that token
this.colour = colour; // to reference the colour of the token piece
this.position = position; // to reference the cell position on the board;
this.tokenUsed = tokenUsed; // to reference whether the token has been used or not.
}
}
I've set the tokenUsed status to false per the example provided for the Player class. Position is set to null to ensure it's not already placed anywhere, but not sure if that is necessary!

Fahim Ahmed Xec
Full Stack JavaScript Techdegree Graduate 24,565 PointsFor token class, below are the constructor properties
- color
- number
- player id
- play

thedren kemp
4,218 Pointsclass Token { constructor(color, amount, leftOver, ){ this.color = color // color of the token this.amount = amount // how many token a p/ayer should have this.leftOver = leftOver // how many token is left well the player is playing } }

Ethan Jarvey Ocampo
11,922 PointsHmm... I am not sure, but I think it should inherit the color property from the Player class. We can associate the player's id to the token as a property, so we could know which token belongs to whom. A token could have a Boolean value to check whether it has been played or not.
Jeffrey Sevinga
8,497 PointsJeffrey Sevinga
8,497 PointsMy take on the Token Class