Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial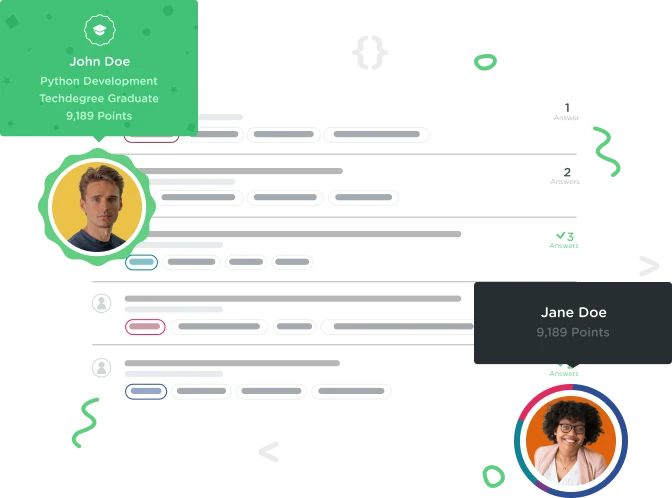

Zack Ball
4,958 PointsToo Many Redirects
I'm stuck with an issue of too many redirects. Two others have posted on this issue one solved it himself and the other person from 2 months ago never got an answer. The person who solved it going over his code my code seems to match his. I've even gone so far as to literally delete all my code and try said Mod's code. Nothing changed. Still getting redirects.
catalog.php
<?php
include("inc/functions.php");
$pageTitle = "Full Catalog";
$section = null;
$items_per_page = 8;
if (isset($_GET["cat"])) {
if ($_GET["cat"] == "books") {
$pageTitle = "Books";
$section = "books";
} else if ($_GET["cat"] == "movies") {
$pageTitle = "Movies";
$section = "movies";
} else if ($_GET["cat"] == "music") {
$pageTitle = "Music";
$section = "music";
}
}
if (isset($_GET["pg"])) {
$current_page = filter_input(INPUT_GET,"pg",FILTER_SANITIZE_NUMBER_INT);
}
if (empty($current_page)) {
$current_page = 1;
}
$total_items = get_catalog_count($section);
$total_pages = ceil($total_items / $items_per_page);
//limit results in redirect
$limit_results = "";
if (!empty($section)) {
$limit_results = "cat=" . $section . "&";
}
//redirect too-large page numbers to the last page
if ($current_page > $total_pages) {
header("location:catalog.php?" . $limit_results . "pg=" . $total_pages);
}
//redirect too-small page numbers to the first page
if ($current_page < 1) {
header("location:catalog.php?" . $limit_results . "pg=1");
}
//determine the offset (number of items to skip) for the current page
//for example on pg 3 with 8 items per page, the offset would be 16
$offset = ($current_page - 1) * $items_per_page;
if (empty($section)) {
$catalog = full_catalog_array($items_per_page,$offset);
} else {
$catalog = category_catalog_array($section,$items_per_page,$offset);
}
include("inc/header.php"); ?>
<div class="section catalog page">
<div class="wrapper">
<h1><?php
if ($section != null) {
echo "<a href='catalog.php'>Full Catalog</a> > ";
}
echo $pageTitle; ?></h1>
<ul class="items">
<?php
foreach ($catalog as $item) {
echo get_item_html($item);
}
?>
</ul>
</div>
</div>
<?php include("inc/footer.php"); ?>
functions.php
<?php
function get_catalog_count($category = null) {
$category = strtolower($category);
include("connection.php");
try {
$sql = "SELECT COUNT(media_id) FROM Media";
if (!empty($category)) {
$results = $db->prepare(
$sql
. " WHERE LOWER(cateogry) = ?"
);
$results->bindParam(1,$category,PDO::PARAM_STR);
} else {
$results = $db->prepare($sql);
}
$results->execute();
} catch (Exception $e) {
echo "bad query";
}
$count = $results->fetchColumn(0);
return $count;
}
function full_catalog_array($limit = null, $offset = 0) {
include("connection.php");
try {
$sql = "SELECT media_id, title, category, img
FROM Media
ORDER BY
REPLACE(
REPLACE(
REPLACE(title,'The ',''),
'An ', ''),
'A ', '')";
if (is_integer($limit)) {
$results = $db->prepare($sql . " LIMIT ? OFFSET ?");
$results->bindParam(1,$limit,PDO::PARAM_INT);
$results->bindParam(2,$offset,PDO::PARAM_INT);
} else {
$results = $db->prepare($sql);
}
$results->execute();
} catch (Exception $e) {
echo "Unable to retrieve results";
exit;
}
$catalog = ($results->fetchAll(PDO::FETCH_ASSOC));
return $catalog;
}
function category_catalog_array($category, $limit = null, $offset = 0) {
include("connection.php");
$category = strtolower($category);
try {
$sql = "SELECT media_id, title, category, img
FROM Media
WHERE LOWER(category) = ?
ORDER BY
REPLACE(
REPLACE(
REPLACE(title,'The ',''),
'An ', ''),
'A ', '')";
if (is_integer($limit)) {
$results = $db->prepare($sql . " LIMIT ? OFFSET ?");
$results->bindParam(1,$category,PDO::PARAM_STR);
$results->bindParam(2,$limit,PDO::PARAM_INT);
$results->bindParam(3,$offset,PDO::PARAM_INT);
} else {
$results = $db->prepare($sql);
$results->bindParam(1,$category,PDO::PARAM_STR);
}
$results->execute();
} catch (Exception $e) {
echo "Unable to retrieve results";
exit;
}
$catalog = ($results->fetchAll(PDO::FETCH_ASSOC));
return $catalog;
}
function random_catalog_array() {
include("connection.php");
try {
$results = $db->query(
"SELECT media_id, title, category, img
FROM Media
ORDER BY RANDOM()
LIMIT 4"
);
} catch (Exception $e) {
echo "Unable to retrieve results";
exit;
}
$catalog = ($results->fetchAll(PDO::FETCH_ASSOC));
return $catalog;
}
function single_item_array($id) {
include("connection.php");
try {
$results = $db->prepare(
"SELECT title, category, img, format, year, genre, publisher, isbn
FROM Media
JOIN Genres ON Media.genre_id = Genres.genre_id
LEFT OUTER JOIN Books ON Media.media_id = Books.media_id
WHERE Media.media_id = ?"
);
$results->bindParam(1,$id, PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
echo "Unable to retrieve results";
exit;
}
$item = ($results->fetch());
if (empty($item)) return $item;
try {
$results = $db->prepare(
"SELECT fullname, role
FROM Media_People
JOIN People ON Media_People.people_id = People.people_id
WHERE Media_People.media_id = ?"
);
$results->bindParam(1,$id, PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
echo "Unable to retrieve results";
exit;
}
while($row = $results->fetch(PDO::FETCH_ASSOC)) {
$item[$row["role"]][] = $row["fullname"];
}
return $item;
}
function get_item_html($item) {
$output = "<li><a href='details.php?id="
. $item["media_id"] . "'><img src='"
. $item["img"] . "' alt='"
. $item["title"] . "' />"
. "<p>View Details</p>"
. "</a></li>";
return $output;
}
function array_category($catalog,$category) {
$output = array();
foreach ($catalog as $id => $item) {
if ($category == null OR strtolower($category) == strtolower($item["category"])) {
$sort = $item["title"];
$sort = ltrim($sort,"The ");
$sort = ltrim($sort,"A ");
$sort = ltrim($sort,"An ");
$output[$id] = $sort;
}
}
asort($output);
return array_keys($output);
}
2 Answers

Denny Schouten
8,246 PointsHi, i think you miss spelled category here it's in the get_catalog_count function, hopefully this will fix your code!
$sql
. " WHERE LOWER(cateogry) = ?"
);
PS. It might help you to fix your code if you echo the Exception $e variable.
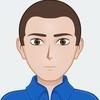
Marc Busby
35,550 PointsAs Denny suggested, you have a spelling mistake in your SQL. Too many redirect errors is normally something simple, like typing - instead of = etc.