Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial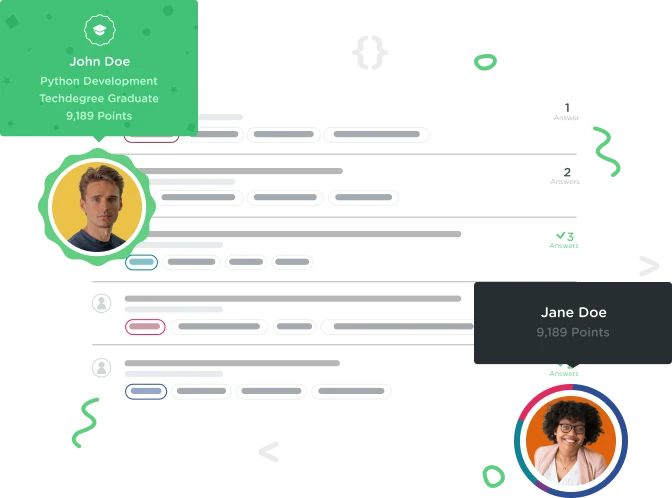
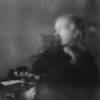
R W
19,894 Points"Too Much Recursion"
I've been following along in the React Basics course and when I finished the code for this video I started getting the error "Too Much Recursion" and the page never loads. I've examined my code and the code from the video several times and I can't find what it is I'm doing incorrectly.
var PLAYERS = [
{
name: "Player 1",
score: 33,
id: 1
},
{
name: "Player 2",
score: 22,
id: 2
},
{
name: "Player 3",
score: 45,
id: 3
}
];
function Header(props) {
return (
<div className="header">
<h1>{props.title}</h1>
</div>
);
}
Header.propTypes = {
title: React.PropTypes.string.isRequired
};
function Counter(props) {
return (
<div className="counter">
<button className="counter-action decrement"> -- </button>
<Counter score={props.score} />
<button className="counter-action increment"> ++ </button>
</div>
);
}
Counter.propTypes = {
score: React.PropTypes.number.isRequired
};
function Player(props) {
return (
<div className="player">
<div className="player-name">{props.name}</div>
<div className="player-score">
<Counter score={props.score} />
</div>
</div>
);
}
Player.propTypes = {
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired
};
function Application(props) {
return (
<div className="scoreboard">
<Header title={props.title} />
<div className="players">
{props.players.map(function(player) {
return (
<Player name={player.name} score={player.score} key={player.id} />
);
})}
</div>
</div>
);
}
Application.propTypes = {
title: React.PropTypes.string,
players: React.PropTypes.arrayOf(
React.PropTypes.shape({
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired,
id: React.PropTypes.number.isRequired
})
).isRequired
};
Application.defaultProps = {
title: "Scoreboard"
};
ReactDOM.render(
<Application players={PLAYERS} />,
document.getElementById("container")
);```
1 Answer

Aaron Price
5,974 PointsThis component calls itself, which creates a recursive loop because every time it calls itself, it calls itself again, and so on, until the machine running the code runs out of RAM and you get a stack overflow.
Recursion is actually pretty cool when you know how to use it, but I suspect it's not what you're aiming to do.
function Counter(props) {
return (
<div className="counter">
<button className="counter-action decrement"> -- </button>
<Counter score={props.score} />
<button className="counter-action increment"> ++ </button>
</div>
);
}
Sam Wight
64 PointsSam Wight
64 PointsAdding to this, if you have time, you should look at some of ES6's features, specifically generators and
yield
.yield
basically allows you to do recursion as much as you want while returning values as the loop goes along. Really cool stuff.R W
19,894 PointsR W
19,894 PointsYeah, recursion is definitely not what I was aiming for here haha. Is it the Counter calling itself? I'm looking through my code but it's hard to spot which component is causing the recursion.
Sam Wight
64 PointsSam Wight
64 PointsLook at the code in the return function for the counter function. You called
<Counter>
in there, which would mean the function would be calling itself, since React components are functions.