Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial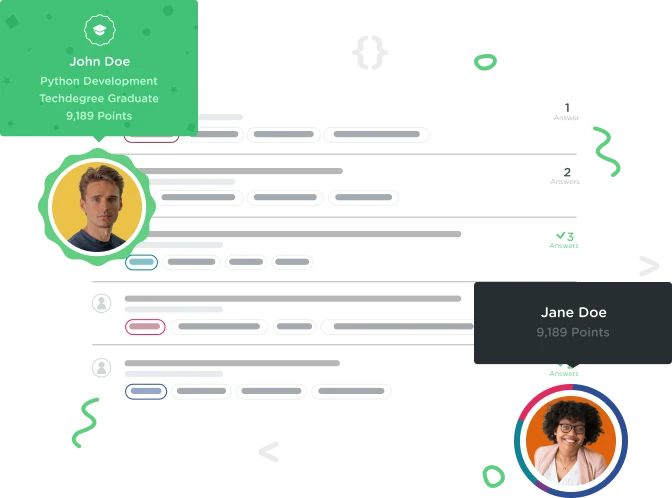
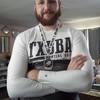
Zach Rebstock
4,632 PointstoString() not working on exercise practice
I am trying to set the passengersField.setText by calling spaceship.getNumPassengers().toString() inside of the setText.
And I get this error:
./LandingActivity.java:28: error: int cannot be dereferenced passengersField.setText(spaceship.getNumPassengers().toString()); ^ 1 error
public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_landing);
thrustButton = (Button)findViewById(R.id.thrustButton);
typeLabel = (TextView)findViewById(R.id.typeTextView);
passengersField = (EditText)findViewById(R.id.passengersEditText);
spaceship = new Spaceship("FIREFLY");
typeLabel.setText(spaceship.getShipType());
passengersField.setText(spaceship.getNumPassengers().toString());
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends AppCompatActivity {
public Button thrustButton;
public TextView typeLabel;
public EditText passengersField;
public Spaceship spaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
thrustButton = (Button)findViewById(R.id.thrustButton);
typeLabel = (TextView)findViewById(R.id.typeTextView);
passengersField = (EditText)findViewById(R.id.passengersEditText);
spaceship = new Spaceship("FIREFLY");
typeLabel.setText(spaceship.getShipType());
passengersField.setText(spaceship.getNumPassengers().toString());
}
}
public class Spaceship {
private String shipType;
private int numPassengers = 0;
public String getShipType() {
return shipType;
}
public void setShipType(String shipType) {
this.shipType = shipType;
}
public int getNumPassengers() {
return numPassengers;
}
public void setNumPassengers(int numPassengers) {
this.numPassengers = numPassengers;
}
public Spaceship() {
shipType = "SHUTTLE";
}
public Spaceship(String shipType) {
this.shipType = shipType;
}
}
1 Answer
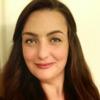
Jennifer Nordell
Treehouse TeacherHi there! The toString
is a method on the Integer class which accepts an integer as an argument. Your toString
is not accepting anything and is chained onto the end after the getNumPassengers
. But the number returned by the the method to get the number of passengers is what should be sent in to toString
. If you want to use toString
, you could do it like this:
passengersField.setText(Integer.toString(spaceship.getNumPassengers()));
This goes out and gets the number of the passengers and then passes it into the toString
method which then returns a string. The string then is passed into the setText
.
Alternatively, and a little shorter, you could opt to use Java's type inference:
passengersField.setText(spaceship.getNumPassengers() + "");
By concatenating an empty string onto the end, Java forces the other part to be a string automatically.
Hope this helps!