Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial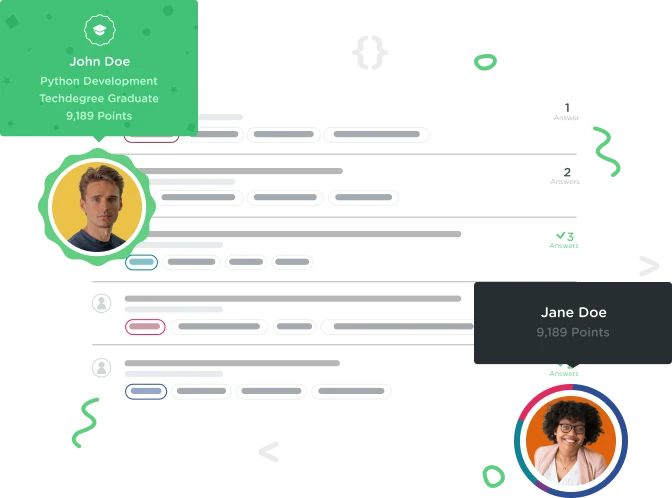

kyle hansen
2,132 Pointstotal loss for overloading a constructor
i am working on the shopping cart exercise and seem to have zero comprehension on the idea of overloading a constructor ? is there any resource that can explain the concept to me further, for i have watched the corresponding video several times and dont seem to be getting anything further from it
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
public int addItem(){
}
return addItem(quantity =+ 1);
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers
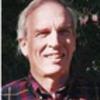
jcorum
71,830 PointsThis is the original addItem() method. It takes two parameters: item and quantity:
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
They want you to override it, create a version of it, so that it takes only 1 paramter: item, with the quantity understood to be 1.
To override means to write, in the same class, another method with the same name but a different method signature (number and type of parameters). One way you could do it (but not a DRY way) would be:
public void addItem(Product item) {
System.out.printf("Adding %d of %s to the cart.%n", 1, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
But this repeats the code in the overridden method. What they want you to do is call the overridden method in the overriding method:
public void addItem(Product item) {
addItem(item, 1);
}
Here none of the code in addItem(Product, int) is repeated.
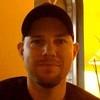
Jeremy Hill
29,567 PointsIn my own words an overloaded constructor is multiple constructors each with varying parameters to allow users to use only the arguments that are needed for their specific program.
For instance: if I was to use the program class Product but I only needed to use the constructor with String name and double price I could instantiate that class with just those two arguments. Later if someone else wanted use class Product with String name, double price and String Color they could instantiate the class with just those arguments, but if you only had one constructor with say three arguments but the user only needed two of them then the program wouldn't work without adding an arbitrary argument. Having multiple constructors (overloaded)this makes it more universal for lack of a better word. I hope this helps. You can message back if you have any more questions.
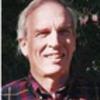
jcorum
71,830 PointsKyle, sorry, I forgot to add: addItem() isn't a constructor. It is a method.
Here's an example of a constructor, taken from the Product class:
public Product(String name) {
mName = name;
}
As Jeremy indicates, you can have multiple constructors in a class, and often for convenience. The bottom line is that each constructor must initialize all the class's instance variables, either with a default value or with a value passed in as an argument when the constructor is called.
So if Product had a instance variable mColor, then the above constructor would have to be changed either to accept a value for mColor:
public Product(String name, Color color) {
mName = name;
mColor = color;
}
or set a default for it in the constructor:
public Product(String name) {
mName = name;
mColor = new Color("red");
}
Client programs could change the color, if they didn't like red, using one of Product's setter methods: the one to set the color.
Or, set a default for the instance variable directly:
public class Product {
private String mName;
private Color mColor = new Color("red");
. . .