Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial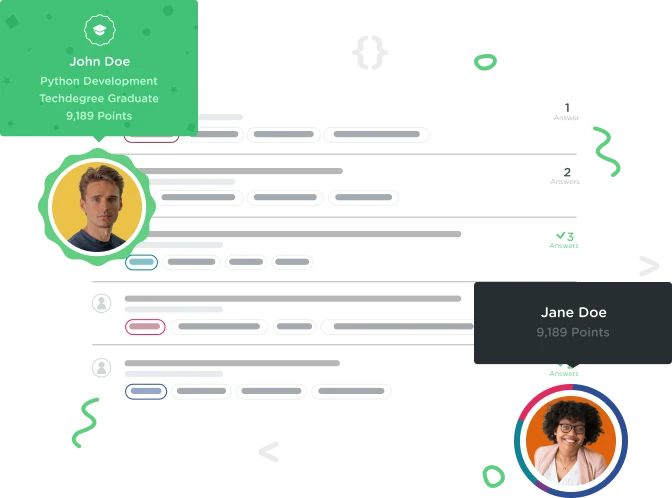

Edmundo Ruiz
3,488 Pointstotally lost
Add a method to the object literal called countWords(). countWords() should return the number of individual words in the string property.
I have bent this several ways and cant seem t find a solution.
const myString = {
string: "Programming with Treehouse is fun!" ,
countTheWords: function(){
this.string.countWords();
}
}
countWords.(myString.string);
2 Answers

Cooper Runstein
11,850 PointsYou need to create a method called countWords that exists for the myString Object. You kinda got that part, but you need to change the name countTheWords to simply countWords.
countWords needs to do two things:
It needs to access value of the string that the property 'string' of myString contains. In other words, we need to be able to get the "Programming with Treehouse is fun!", or whatever else that property might become.
We need to find a way to get the number of words exist in that string, and return an integer representing this.
To tackle the first step, we need to use the this keyword.
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/this
- https://teamtreehouse.com/library/understanding-this-in-javascript
- https://www.w3schools.com/js/js_this.asp
This gives us access to the internal properties of the myString class from within. It looks like this:
const myString = {
string: "Programming with Treehouse is fun!",
countWords: function(){
return this.string
}
}
Now if we run myString.countWords
we'll get back the value of 'string', that's a good first step.
Now that we know we have access to the string property, we need to count the words in it. This can be divided into two further steps:
- Figure out how to split the string into "words"
- Count and return the number of words
Step one can be done a few ways, but I prefer the string.split()
method javascript comes with.
Checkout https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/split
Quickly, this method works as follows:
"I am a big string, make me into words".split(' ') //Split on empty spaces (this is what makes a word) =>
//['I', 'am', 'a', 'big', 'string,', ... 'words']
Next we can access the length of the array we created by splitting the string using the built in length method: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/length
['I', 'am', 'a', 'big', 'string,', ... 'words'].length //get the length
//9
Once we return that, our method is complete. All together, that looks like this:
const myString = {
string: "Programming with Treehouse is fun!",
countWords: function(){
const wordArray = this.string.split(' ');
return wordArray.length;
}
}
Hope that helps you, and anyone else that's struggling with this question!

Mohamad Khalili
8,271 PointsThis did it for me.
const myString = {
string: "Programming with Treehouse is fun!",
countWords: function(){
return this.string.split(' ').length;
}
}