Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial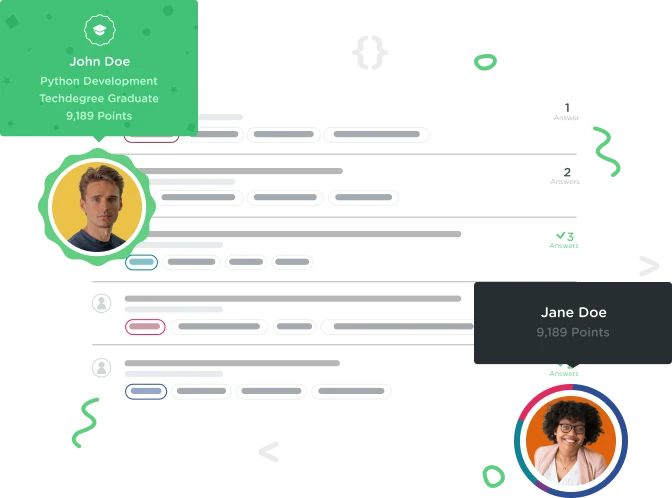
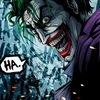
fredyrosales
10,572 PointstotalPoints
HI! Great Course btw i really find it fun! I just need a little help with this line of code.
var totalPoints = props.players.reduce(function(total, player) {
return total + player.score;
}, 0);
If anyone can iterate it a bit more i would be very grateful! Thanks
3 Answers
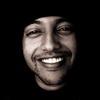
miikis
44,957 PointsHey Fredy,
That reduce method belongs to the JS Array object. It's main purpose is to take a list of things and "reduce" them to one thing. One of it's primary use-cases is to perform a summation on a list of values, as is the case here.
Quick example of summing a list of consecutive integers:
const consecutiveIntegers = [1, 2, 3, 4, 5, 6]
const result = consecutiveIntegers.reduce(function (prev, current, index, array) {
return prev + current
}, 0 /* this zero here is optional; if we didn't put it there, it would default to zero anyway*/)
// console.log(result) would log 21
You presumably have an Array somewhere called players. Players probably looks something like this:
const players = [{
name: 'dude',
points: 5000
}, {
name: 'dudette',
points: 1000
}, {
name: 'dude-bro',
points: 2321
}, {
name: 'broseph',
points: 2323
}]
Say you wanted to find the total points of all players. Traditionally, you'd need a for-loop, or a while-loop, or Array#forEach to do this; but, there's another way of life — Array#reduce. Array#reduce takes two parameters: a function and an optional starting value that defaults to 0. Inside of the first parameter — the function — you'll write the algorithm for how exactly you want to reduce your values. If you have a bunch of numbers — like consecutiveIntegers — above, it's simple; you can just add them. But what about your players array? That contains a bunch of object literals. As you are probably aware, it doesn't make any sense to add two object literals together (you'd get some string interpolation of [object Object] returned to you). Besides, we just want the points to be summed. So, we do exactly that; we sum the points property of each object.
const totalPoints = players.reduce(function (prev, current, index, array) {
// REMEMBER: prev and current here correspond to the previous and current object in the players list
return prev.points + current.points // this will return a number — 10,644, to be exact
}, 0)

Iain Simmons
Treehouse Moderator 32,305 PointsMikis Woodwinter is almost correct, except the prev
parameter in the reduce
function will already contain the reduced value of whatever you're doing in the function, in this case, a number containing the total points.
It won't be an object with a points
property.
So you'd want:
const totalPoints = players.reduce(function (prev, current, index, array) {
// REMEMBER: prev and current here correspond to the previous and current object in the players list
return prev + current.points // this will return a number — 10,644, to be exact
}, 0)
In any case, another way to look at reduce
is to think of it as being like a function called on each iteration of a loop over the array, where you're changing some variable holding your 'total' and then returning it once you've finished looping.
So the longer, less elegant way of writing the reduce
might look something like this:
function sumPlayerPoints(players) {
var total = 0,
i;
for (i = 0; i < players.length; i++) {
total = total + players[i].score;
}
return total;
}
var totalPoints = sumPlayerPoints(players);
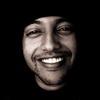
miikis
44,957 PointsOh shit. Yeah, you're right. Don't know what I was thinking. Good catch :)
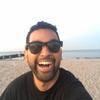
Anthony Albertorio
22,624 PointsSee this video for a good explanation from Fun Fun Function: https://www.youtube.com/watch?v=Wl98eZpkp-c
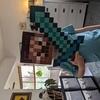
Gabbie Metheny
33,778 PointsMattias is the best, that video was how I learned reduce, too!