Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial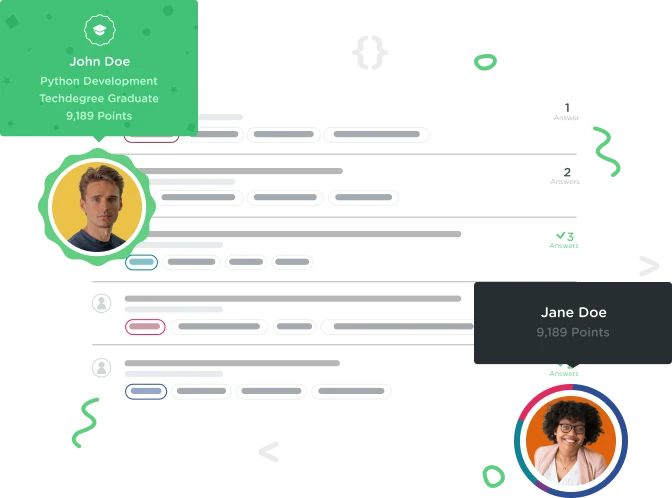

Ben King
Courses Plus Student 4,350 PointsTotlally confused...
Trouble with task 2 giving arguments to functions. I find it easy to follow illustrated "goToCoffeeShop" examples, but take away the illustrations and I find myself in a confused state. Please can you explain the problem with my code? Thank you.
Ben
function returnValue (amount) {
var echo = "this is interesting";
}
var echo = returnValue;
returnValue( echo + amount);
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
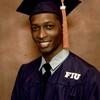
Dane Parchment
Treehouse Moderator 11,077 PointsHello Ben! I am going to help you solve your dilemma!
First though, let's take a look at the problems in your code:
function returnValue (amount) {
var echo = "this is interesting";
}
var echo = returnValue;
returnValue( echo + amount);
Though you have generally structured the function properly, this is not the proper way to return a value from a function, nor is it the proper way to call a returned value from a function. First let's look at your syntax for the function with parameters and a return. You will have to do something like this:
function returnValue (amount) {
return amount;
}
As you can see in the bottom example the function is actually returning a value, in programming/software engineering we sometimes refer to functions that have a return value as a getter because it gets a value for us to use. However, by itself this getter function will not actually use the data that it gets. In order to use or store a value that a getter function will retrieve for us, we need to store it to a variable like this:
function returnValue (amount) {
return amount;
}
var echo = returnValue("This is the value of the parameter here!");
As you can see we store the value of the variable echo as the returned value of the function returnValue (that was a mouthful). The above example will provide a correct answer to your solution, however, I believe that you need to refresh your mind on the concept/structure of a function, the parameters of a function, and return values. I will give you a quick course below:
Functions
//Below is the syntax of a function
function function_name(list_of_parameters) {
//body of a function
return return_value; //An optional return value
}
A function will allow you to perform a box of code with just one line, anywhere in your program, if you see large chunks of repeated code it is probably best to turn it into a function for easier use/readability/staying DRY (do no repeat yourself). A function can include a list of parameters that you can describe as just variables that provide information for your function to use. For example, the below function will print out your name and age, provided by the user as parameters, to the console.
function displayUserInfo(name, age) {
console.log(name);
console.log(age);
}
displayUserInfo("Dane", 20);
When I call the function (as I did on the last line of code) I will get the values:
Dane
20
written to the console.
Whatever, happens in a function for the most part generally stays in a function, and as such we sometimes need to provide a way for a function to store it's value for later use, we can do this with returns, that will not only exit a function at the return call, but also send the value that it is returning into a temporary memory location that can be later sent t o a variable for permanent storage. For example, the function below, will store the area of a square, given the length and with as parameters.
function areaOfSquare(length, width) {
return length * width;
}
var area1 = areaOfSquare(3, 2);
var area2 = areaOfSquare(10, 5);
If we were to console.log the 2 variables that hold the value of the return, we will get (6, and 50).
Hopefully this answers your question and more. If you need any more help understanding feel free to ask. Though I do recommend re-watching the videos about functions.
Ben King
Courses Plus Student 4,350 PointsBen King
Courses Plus Student 4,350 PointsThank you Dane, I am kind of a newbie to programing. It will take me a while to get it. I will study your words carefully tomorrow. Really grateful for such a concise reply. Thanks again
Ben