Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial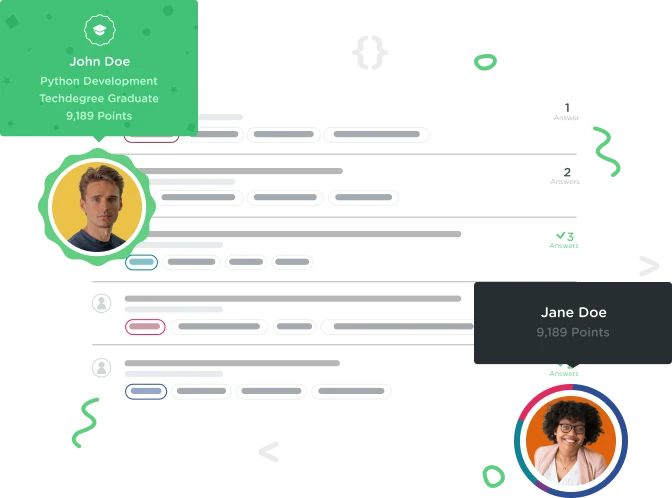
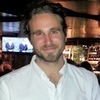
Justin McAuley
6,643 PointsTough lecture to follow conceptually.. any other resources?
For some reason, this lecture (Anonymous Functions) seemed relatively (compared to the rest of the lectures) unclear and difficult to understand.
Does anyone have any other resources, inside or outside of treehouse, that might be helpful in conveying this concept a little more clearly? Or perhaps someone can offer their own perspective/insight on understanding the conceptual core of anonymous functions.
3 Answers
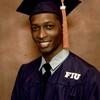
Dane Parchment
Treehouse Moderator 11,077 PointsI can probably help you out some.
Anonymous functions are not that difficult to conceptualize once you understand them, at its most basic an anonymous function is simply a function without a name.
//This is a regular function
function sayHi() {
alert("Hello! What's up?");
}
//This is an anonymous function
var sayBye = function() {
alert("Alright then! See ya later!");
}
//Both are called similarly
sayHi();
sayBye();
The two main ways you will see anonymous functions utilized in JavaScript is via closures or as an argument for another function.
//========================================================================
// An example of a closure
// The surrounding braces are that of a wrapper
// for the anonymous function
// The ending curly braces are that of the function
// itself being called, this will include any
// parameters that you added (if any) and will ensure that the
// function is run when the interpreter //reaches it.
//========================================================================
(function() {
alert("This was said by a closure!!!");
}()); //
// An example of an anonymous function
// being used as an argument.
// Basically the syntax is setting up a timeOut and
// instead of giving
// two simple parameters we make one parameter
// an anonymous function that alerts a message
setTimeout(function() {
alert('hello');
}, 1000);
Hopefully that helps you understand it a bit more, if you still don't get it, let me know and I will try to explain it again in better detail (Most of the sites I know, the explanation will be quite complex).
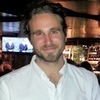
Justin McAuley
6,643 PointsIn case anyone else comes across this looking for help with javascript closures:
http://stackoverflow.com/questions/111102/how-do-javascript-closures-work
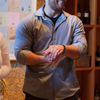
Jonathan Ankiewicz
17,901 PointsI completely agree, super easy to get lost.
Justin McAuley
6,643 PointsJustin McAuley
6,643 PointsThanks @Dane Parchment. This is helpful.
I understand using an anonymous function to pass as an argument. But the closure use case is a bit cloudy for me. Mainly, what's the purpose of the parentheses right after the closing curly bracket of the anonymous function. i.e.
( /this parenthesis to the left */ function() { alert("This was said by a closure!!!"); }( ) / and these parentheses to the left */ );
Are they just for syntax purposes? So simply adding two parenthesis after a function (i.e." } ( ) " ) calls the anonymous function to execute??
Dane Parchment
Treehouse Moderator 11,077 PointsDane Parchment
Treehouse Moderator 11,077 PointsYes, basically writing those two parentheses is the same as doing:
When you add those two parentheses (along with any parameters) it allows the function to run automatically instead of having to be called through an event.
Remember you can't really call a unnamed function, that is what the two parentheses at the end of the anonymous function are for..hope this helped!
Justin McAuley
6,643 PointsJustin McAuley
6,643 PointsAhhhh.. just had that 'aha' moment with your last sentence there. This makes perfect sense. Thanks a lot, man.