Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial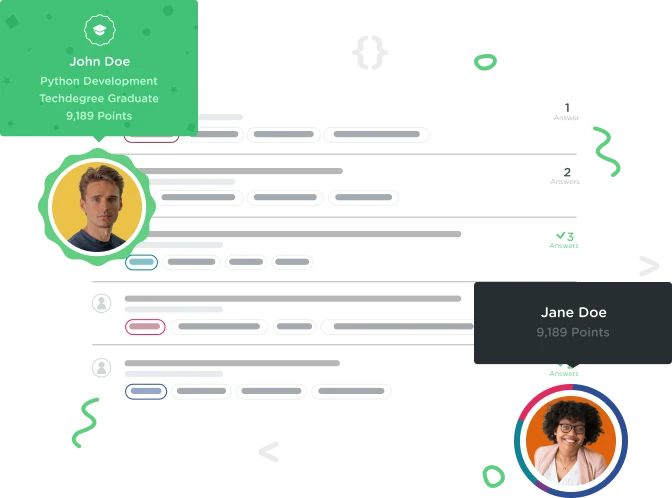

detlefbrune
1,061 PointsTowers cant be placed on path, without looping
In the video the instructor asks us to use what we've previously learned to solve the problem. At this point we haven't learned loops yet so I'd like to stick to the lesson and not use loops for this solution.
We need to check that the location of the tower is not on the path before placing it. And we should use the example of "OnMap" on the MapLocation class to achieve this. Here is what I've done so far (and what I need help with).
-
On the Path class I wrote a OnPath method and used the same X and Y parameters that I got from the map we've created on Main to check for it. I believe this would be correct as long as the map in main keeps those parameters, but if it changes then this would have to change as well. Is there a better solution for this?
public bool OnPath(Point point) { return point.X == 0 || point.X == 1 || point.X == 2 || point.X == 3 || point.X == 4 || point.X == 5 || point.X == 6 || point.X == 7 && point.Y == 2; }
On the Tower class I get no compiler errors but I would like to know if this fits the requirements. I'm not sure if it was correct to add a 'Path path' parameter on the Tower constructor and I don't know whether the "if (!path.OnPath(location))" is actually right because on OnMap in MapLocation it was "if (!map.OnMap(this))". When I used "(this)" here I would get compiler errors
class Tower { private readonly MapLocation _location; private readonly Path _path;
public Tower (MapLocation location, Path path)
{
_location = location;
_path = path;
if (!path.OnPath(_location))
{
throw new OutOfBoundsException("Towers can't placed on the path");
}
}
}
2 Answers
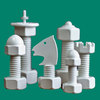
Steven Parker
231,275 PointsLoops make sense here.
I'm not sure why you would want to avoid loops, the video you have linked this question to is titled While Loops.
A much better solution is to use a loop to check your point against the actual points on the path. As you pointed out yourself, coding fixed values into the test is very "brittle" (easily broken by other code changes).
It makes sense to have a path parameter to the constructor for checking validity, but since it is only used that one time, you don't need to preserve it in a class variable.
The reason you can't use "OnMap(this)
" here is because "this" refers to the Tower object being constructed, and the OnMap method expects a Point or MapLocation value as a parameter (and that's why it works in the MapLocation constructor). But since the location is being passed in as a parameter, you could use "OnMap(location).
".
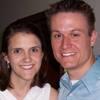
Shaun Barbour
Courses Plus Student 1,907 Pointshe's wanting to avoid loops, because the instructor asks us to pause the video and figure it out before he teaches us about loops.
detlefbrune
1,061 Pointsdetlefbrune
1,061 PointsThank you!