Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial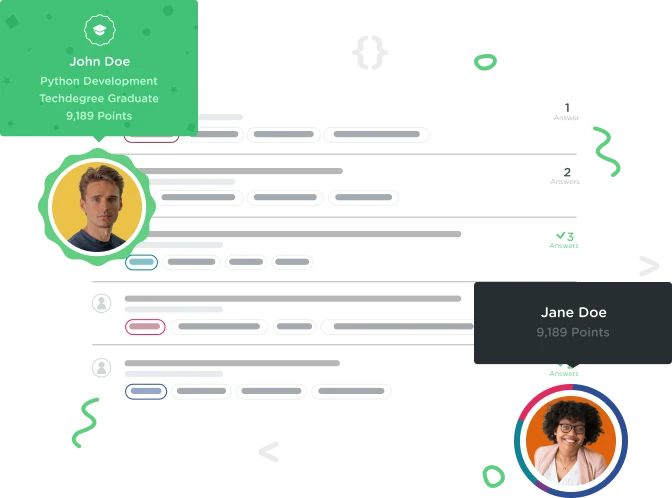

Nascent Noob
1,062 PointsTowers on path Help
I am having trouble with the challenge to write validation for a tower not being on a path. I've reviewed where others have asked for help but still can't find a solution or understanding of the intent.
At the moment I know the Tower constructor will need to take in a MapLocation for the tower's proposed location, a Path to check against and it's best to use a loop. However, I don't understand how a Tower can be on a MapLocation without being on a Path.
Trying to make something I copied the OnMap method to Path.cs, renamed it to OnPath and changed the max check to be less than Length of path as for a tower to be on Path the first step off the path is Length of the path.
public bool OnPath(MapLocation location)
{
return location.X >= 0 && location.X < Length &&
location.Y >= 0 && location.Y < Length;
}
Then in Tower.cs I have
class Tower
{
private readonly MapLocation _location;
public Tower(MapLocation location, Path path)
{
_location = location;
if(path.OnPath(location))
{
throw new OutOfBoundsException("Tower is on a path!");
}
}
}
Problem is I don't understand what the goal is therefore guessing at how or why is difficult. Doesn't a Tower have to be on a path for it to be on the map? How do I know a solution I implement is correct and won't be incompatible with design pattern used by lecturer later on?
Could someone please provide some starter steps to nudge me in the right direction? I've spent a couple hours reviewing other answers, trying different things and rewriting this question so sorry if it was incoherent.
Side Note: I think a diagram showing how the classes interact or specifically the components involved in the model validation method would provide clarification for students trying to create their own validation method.
1 Answer
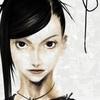
S Hedron
17,123 PointsHi --
I made a quick image for reference here.
It's important to remember that a map and a path are both collections of points. The map is the collection of all points (all squares in the image) for a given level and the path (red squares) is the specific collection of points that the enemies move on.
Your OnPath checks always return true because it's checking the entire span of the map (0-0 through 3-2). Looking at the grid image example, we only need to return true if it's in 0-1, 1-1, 2-1, or 3-1. All other points on the map would be legal. Because a path is a collection of points, all you need to do is check your proposed location point against each of the points in the current path.
Hopefully that helps!