Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial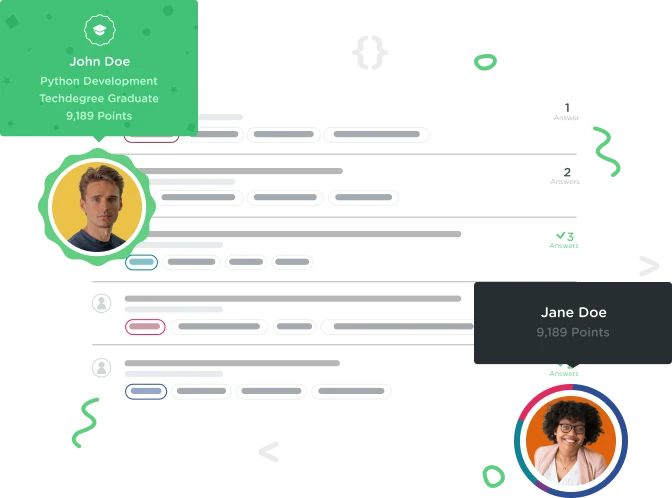

Abdulrahman Al Ani
PHP Development Techdegree Graduate 17,607 PointsTracking an action with jQuery
Hello everyone,
I really hope I can get some help here. This is my very first project and I'm trying to build a very basic shopping cart (it won't be linked to a DB or backend language, just something to practice what I learn on tree house with jQuery).
What I'm doing is I have "add to cart" button when it's clicked it will add the content of the product to the cart and the button will be disabled because I only want the user to add the product to the cart once.
Please see my code here
('.add-cart').on( "click", function() {
/// this will add the product info to the cart table
$('table').append( '<tr>'
+ '<td><img src="' + $( this ).prev().prev().prev().attr('src') + '" alt="" height="50px" width="50px"></td>'
+ '<td>' + $( this ).prev().prev().text() + '</td>'
+ '<td>' + $( this ).prev().text() + '</td>'
+ '<td class="item-remove"><img src="assets/error.svg" height="20px"></td>'
+ '</tr>' );
/// this will disable the button so they can only add the product to the cart once
$(this).prop('disabled', true);
});
And I have this code giving the user the ability to delete the product from the cart
$(document).on( "click", ".item-remove", function() {
/// this will remove the product from the cart
$( this ).parent().remove();
});
The issue I'm facing is once that "add to cart" button is disabled on the first click event it remains disabled even after the user deletes the product from the cart.
Is there somehow I can create a tracker that checks to see if the product has been removed from the cart to activate the button again?
Now, I understand this is probably not the best way to achieve the functionality of a basic shopping cart, but like I said this literally my first coding project.
I normally search google for finding a solution, but I really don't know what to search for here, So even if you can't provide the full answer if you can just direct me to what I should be searching for that would be so much appreciated.
I also have a Codepen for the code for better visibility: https://codepen.io/alani95/pen/qBBKEMP
Thank you so much in advance.
2 Answers
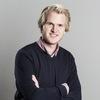
Halvard Bastiansen
Data Analysis Techdegree Student 14,886 PointsGreat job with your first project! It was a cool challenge :)
You can add $(".add-cart").prop('disabled', false); to your click handler for the item-remove button.
But that will enable both add to cart buttons since you use the same class name for both buttons. Meaning you can add multiple items of the same product.
So the best way would be to specify what products you are adding and which product you are removing. One way to do that would be to name your add to cart buttons "add-cart-product1" and "add-cart-product2" in your HTML file. And then update your event listeners to target each of them individually.
Something like this:
// Event listener for product 1
$('.add-cart-product1').on( "click", function() {
$('table').append( '<tr>'
+ '<td><img src="' + $( this ).prev().prev().prev().attr('src') + '" alt="" height="50px" width="50px"></td>'
+ '<td>' + $( this ).prev().prev().text() + '</td>'
+ '<td>' + $( this ).prev().text() + '</td>'
// NB: Renaming the remove button
+ '<td class="item-remove-1"><img src="http://box3082.temp.domains/~aalanibl/error.svg" height="20px"></td>'
+ '</tr>' );
$(this).prop('disabled', true);
});
// Event listener for product 2
$('.add-cart-product2').on( "click", function() {
$('table').append( '<tr>'
+ '<td><img src="' + $( this ).prev().prev().prev().attr('src') + '" alt="" height="50px" width="50px"></td>'
+ '<td>' + $( this ).prev().prev().text() + '</td>'
+ '<td>' + $( this ).prev().text() + '</td>'
// NB: Renaming the remove button
+ '<td class="item-remove-2"><img src="http://box3082.temp.domains/~aalanibl/error.svg" height="20px"></td>'
+ '</tr>' );
$(this).prop('disabled', true);
});
// Remove button for product 1
$(document).on( "click", ".item-remove-1", function() {
$( this ).parent().remove();
$(".add-cart-product2").prop('disabled', false);
});
// Remove button for product 2
$(document).on( "click", ".item-remove-2", function() {
$( this ).parent().remove();
$(".add-cart-product2").prop('disabled', false);
});
Its not really DRY programming, but it will get you there :)

Abdulrahman Al Ani
PHP Development Techdegree Graduate 17,607 PointsYea that seems like a good option, and I agree with you it's not the most DRY code, but this will do for now.
Thank you for your help