Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial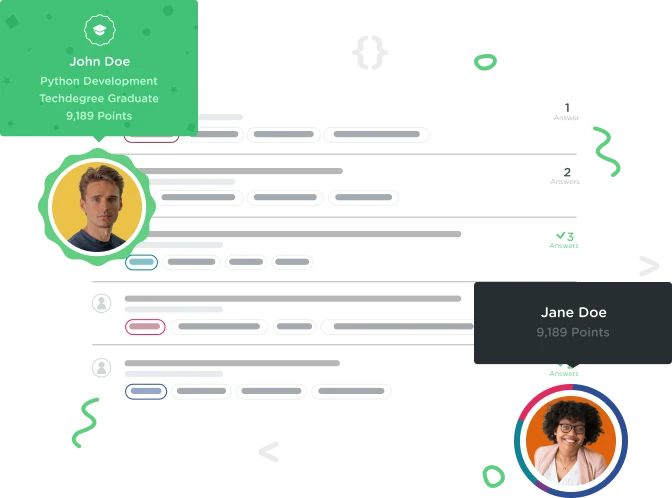
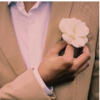
Muaaz Matwadia
Full Stack JavaScript Techdegree Graduate 19,327 PointsTransverse
How do I do this challenge?
var removeMe = document.querySelector('.remove_me');
var parent = ;
<!DOCTYPE html>
<html>
<head>
<title>Parent Traversal</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<ul>
<li>Hello</li>
<li>Hi</li>
<li class="remove_me">Good bye!</li>
<li>Howdy</li>
</ul>
<script src="app.js"></script>
</body>
</html>
2 Answers
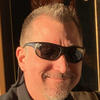
Peter Vann
36,427 PointsThis passes:
var removeMe = document.querySelector('.remove_me');
var parent = removeMe.parentNode;
for more info, check out: https://www.w3schools.com/js/js_htmldom_navigation.asp
and
https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model
That's task 1 of 2.
For task 2 of 2 you have to remove the parent's child element that you started with, therefore this passes:
var removeMe = document.querySelector('.remove_me');
var parent = removeMe.parentNode;
parent.removeChild(removeMe);
I hope this helps. Happy coding!
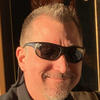
Peter Vann
36,427 PointsBy the way, don't feel bad/discouraged. I find DOM transversal syntax clunky and less-than-intuitive (and therefore, kind of confusing). I frequently have to do a LOT of trial-and-error before I can target and manipulate the right element.
It could use an update/overhaul/revision, in my opinion.
Again, I hope that helps. Happy coding!