Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial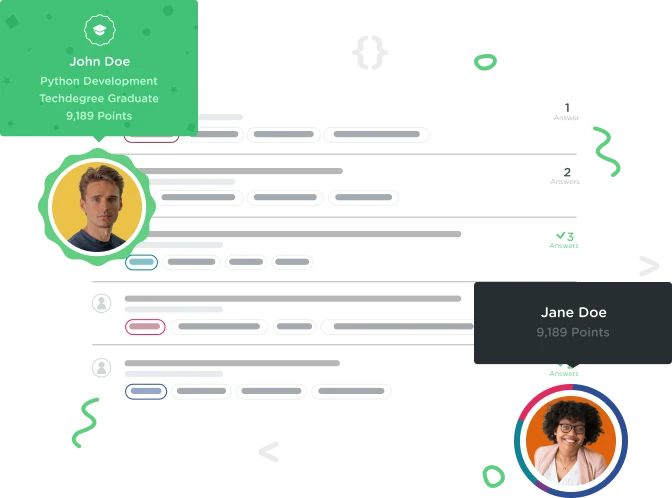
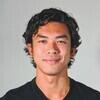
fediesonlandicho
17,818 PointsTraversing an array that contains dictionaries in Swift
I am trying to fish out information from this array that has items that are dictionaries. So for example:
{
array item 0 = {
key = value;
key = value;
key = value;
}
array item 1 = {
key = value;
key = value;
key = value;
}
array item 2 = {
key = value;
key = value;
key = value;
}
}
So I guess I'm trying to iterate each array item and print out a key value pair within each array's dictionary. Can anyone point me in the right direction?
4 Answers
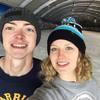
Dominic Bryan
14,452 PointsWhat I suggest in the case you wish to for example print the second item of each dictionary in the array is a simple for loop:
var item = [
[1 : "Tom", 2 : "Dave"], [1 : "Green", 2 : "Blue"]
]
for var i = 0; i < item.count; i++
{
var newItem = item[i]
println(newItem[2])
}
Effectively the same code just with a for loop at the end to iterate over the dictionaries.
Hope this was of help Thanks Dom
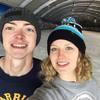
Dominic Bryan
14,452 PointsIt works for the layout you have used in your question, I just made a couple of arrays and dictionaries :)
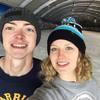
Dominic Bryan
14,452 PointsThinking about it I can't quite figure out how to get it directly so something like item[0["Key"]], but I have another solution that is potentially easier to read anyway. try calling the array and the index in that array with the dictionary you need, then setting this to a variable, then call the new variable with the key for the value you want. Here is an example:
//This is the array of dictionaries
var item = [
[1 : "Tom", 2 : "Dave"], [1 : "Green", 2 : "Blue"]
]
//This would return [1 : "Tom", 2 : "Dave"] as you are taking the array item, and asking whats in index 0
//Then setting it to a variable
var lookForDic = item[0]
//This then take the new variable you created and you ask for the key 2
//This returns/prints "Dave"
lookForDic[2]
Hope this is a little bit helpful, I know it's not what you wanted but it's pretty close right :D
Give it the green tick if you like the answer (it helps me out a lot) and just ask if you need anything else
Thanks Dom
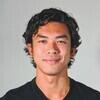
fediesonlandicho
17,818 PointsThats pretty close. I wanted to iterate each array item and call the specific values. For example I would want the second value from each array, printing to the console: "Dave", "Blue"
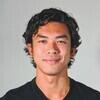
fediesonlandicho
17,818 PointsThanks Dom.
I am able to run your example code just fine in a playground.
The iterations work, but I am unable to get the println(newItem[2]) to work for my data. Swift gives me an error: 'AnyObject?' does not have a member named 'subscript'
I think it has to do with how my data is structured or how the optionals are manipulated.
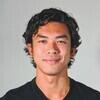
fediesonlandicho
17,818 PointsI think I just literally figured it out after my last post. I changed Dom's code to:
var newItem: NSDictionary = item[i] as NSDictionary!
println(newItem[2])
and now I am able to call the key/value pairs. They are in the console as:
Optional (value)
Optional (value)
Optional (value)
Optional (value)
and its exactly what I needed to move forward.
So can anyone elaborate on what exactly is going on when I added NSDictionary! to Dom's code?
Dominic Bryan
14,452 PointsDominic Bryan
14,452 PointsI am a little confused reading the code example, does each "item" equal a different array or what, it seems like you are calling 4 arrays which hold key:value pairs which doesn't make sense. Could you display in a code field?