Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial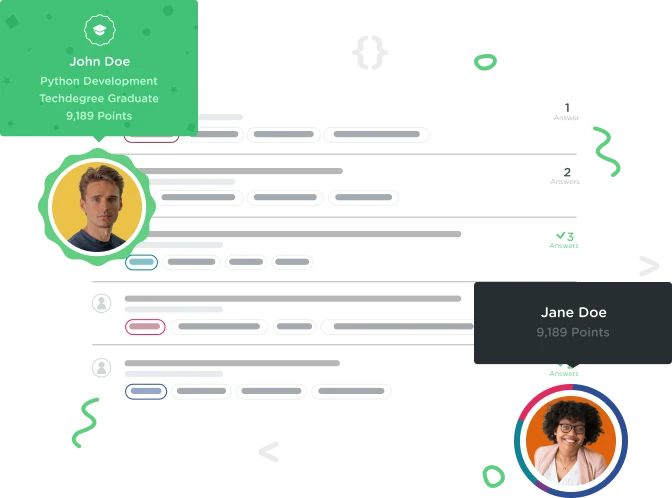

Adam Harms
9,826 Pointstraversing nested lists, need help in python
If I have a list now can I get all of the items out of the lists? The catch is for me, that it has lists within the lists and both integers and strings in the list. Thanks
python
test = [1, 2, [0, [3, 5, 'weather'], 1], 'hats', [0, 1]]
How can you pull out so it prints like this... (1, 2, 0, 3, 5, 'weather', 1, 'hats', 0, 1)
1 Answer
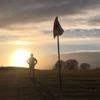
Stuart Wright
41,118 PointsThis seems like a good use for recursion. If you've never come across recursion before, it's a really interesting subject to read up on. The important thing to know for the purposes of this problem is that we will write a function that calls itself every time it comes across a nested list inside the list it's traversing. By appending non-list items when you come across them, and extending the outer list with the inner list when you come across a list, you'll end up with the result you're after. You can try it with an even deeper nested list than the one you've given in the example and this code will still work. It's not very easy to explain in a few lines, you might be better off just reading up on the subject if it's completely new to you:
http://openbookproject.net/thinkcs/python/english3e/recursion.html
Here's my solution - line 5 is where the magic happens, as the function calls itself to get to work on the inner list.
def combine(input_list):
output_list = []
for item in input_list:
if type(item) is list:
output_list.extend(combine(item))
else:
output_list.append(item)
return output_list
combined_list = combine(test_list)
print(combined_list)