Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial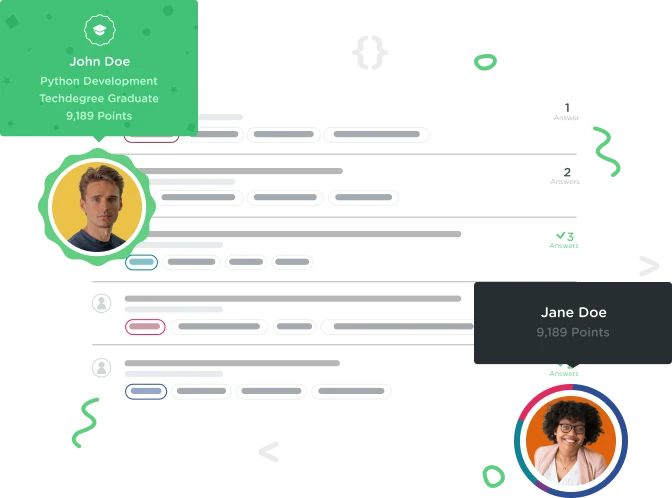

Pushkar Kadam
3,823 Points'treehouse' is giving me back 'trhuse' and how does try and except work?
I wrote a simple code to get an input from the user and remove the vowels. When I type 'apple', the output is 'ppl'. But, when I type, 'treehouse', it gives me 'trhuse'. My observation shows that if a character is repeated more than 3 times, the 3rd time is not removed. But, why wasn't 'u' removed from 'treehouse'. I tried three types of codes.
vowels = list('aeiou')
the_word = input("Enter a word. \n>")
word_list = list(the_word)
for letter in word_list:
if letter in vowels:
word_list.remove(letter)
print("".join(word_list))
Also, this code
the_word = input("Enter a word: \n>")
word_list = list(the_word)
for letter in word_list:
if letter == 'a':
word_list.remove('a')
continue
elif letter == 'e':
word_list.remove('e')
continue
elif letter == 'i':
word_list.remove('i')
continue
elif letter == 'o':
word_list.remove('o')
continue
elif letter == 'u':
word_list.remove('u')
continue
print("".join(word_list))
Both the above code gives me :'apple' gives back 'ppl' but, 'treehouse' gives 'trhuse' But, when I used the code with try and except, my problem was solved. So, how does try and except works in determining the elimination of all the vowels.
vowels = list('aeiou')
the_word = input("Enter a word. \n>")
word_list = list(the_word)
for letter in word_list:
for letter in vowels:
while True:
try:
word_list.remove(letter)
except:
break
print("".join(word_list))
The last code works and 'treehouse' gives me back 'trhs'
1 Answer
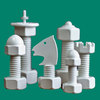
Steven Parker
229,732 Points
Never modify a list while it is being used for loop iteration.
It can cause items to be skipped and/or the loop may end early.
If you intend to modify a list in a loop, iterate using a copy of the list:
for letter in word_list[:]: # using splice to make a copy
john larson
16,594 Pointsjohn larson
16,594 PointsThis code works, but isn't it still iterating and modiying in the try block?