Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial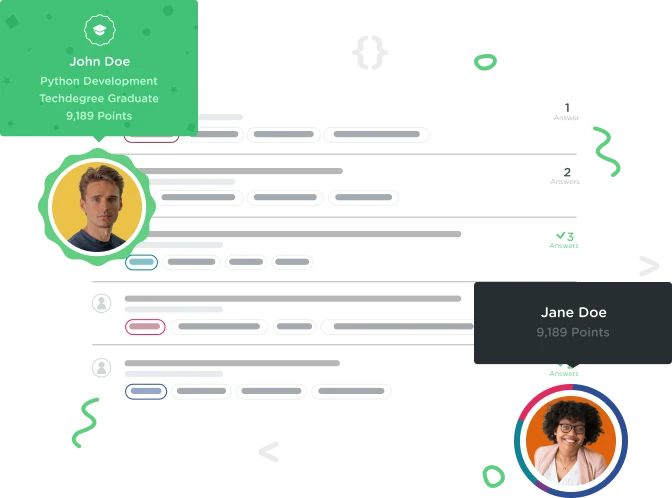
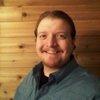
Joel Wood
6,327 PointsTreets - ClassCastException Error
I receive the following compiler error when running the code (code posted separately). Please advise, and thanks for the help:
Exception in thread "main" java.lang.ClassCastException: java.lang.String cannot be cast to [Lcom.teamtreehouse.Treet;
at com.teamtreehouse.Treets.load(Treets.java:25)
at Example.main(Example.java:34)
3 Answers
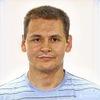
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsThe problem is very simple actually, but hard to find.
You are NOT saving 'treets' array but saving string "treets". Look at the lecture once again and change the following line:
oos.writeObject("treets"); // just some String
TO
oos.writeObject(treets); // actual array passed as argument
So what happened is that you saved String "treets" but not the array. That is why when you call load
program cannot cast String
to Treets[]
.
So uncomment your saving code in Example
.
Change Treets.save
method like I said.
Re-save treets.
Type cat treets.ser
to make sure you see Treets and not Strings ( check with lecture)
And the you can comment "save" part in Example
and use just "load" part
Does it make sense?
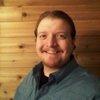
Joel Wood
6,327 PointsWow! This was very informative. You helped me fix the problem, and I learned something new.
Thank you, Alexander and Tomas, for all the help!
-Joel
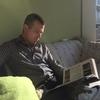
Tomas Schlepers
18,039 PointsThis exception means that two types are not castable. Think of it as apples and peares (IF spelled correct!). You cannot convert an apple into a pear.
In this case, you are trying to print out your object as a whole, and after that the seperate words. When trying to print your object as a String, you need to call the toString() method on it, like this :
System.out.printf("This is a new Treet: %s %n", treet.toString());
Hope this helps!
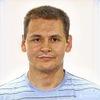
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsBoth statements will have same result
System.out.println(object.toString());
and
System.out.println(object);
Because PrintStream.println
has an overload, so it will call toString
method for you.
Check here for more:
http://stackoverflow.com/questions/16570937/tostring-method-within-system-out-println-a-double-call
What is more important.
If you write:
System.out.println(object.toString());
It will throw NullPointerException
Whereas
System.out.println(object);
will print null
without exception, which is number one of the reason at least for me not to use toString()
explicitly.
Joel Wood
6,327 PointsJoel Wood
6,327 Points