Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial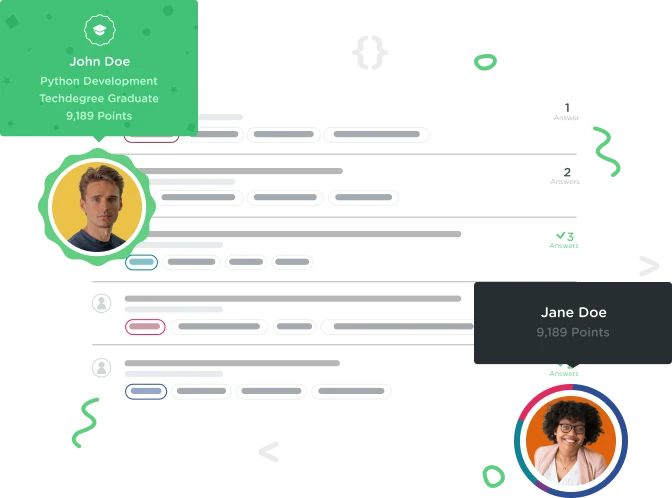

julius murray
919 PointsTried some stuff, require assistance
var ran1 = prompt("please type a number"); var ran2 = prompt("please type another"); if ( isNaN(ran1) || isNaN(ran2) ) { alert("please reload and type a number"); } else { function max(ran1, ran2) { if ( ran1 > ran2 ) { return ran1; } else { return ran2; } } function min(ran1, ran2) { if ( ran1 < ran2 ) { return ran1; } else { return ran2; } } } alert(Math.random( parseInt(max) - parseInt(min) + 1 ) + parseInt(min));
/* This function is technically correct, but is not doing what I want it to. I want to take in two numbers, sort through them and generate a random number between those two. */
1 Answer
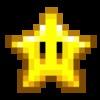
Tom Achki
3,680 PointsI assume you mean to set upperLimit to result of function max(), and lowerLimit to result of funtion min(). (Basically the lower number that user entered as lower limit and higher number that user entered as upper limit) Here is the code implementing the idea I just said.
There were multiple errors in your code.
- Random number generator formula was implemented incorrectly, so here is the formula: var randomNumber = Math.floor(Math.random() * (upperLimit - lowerLimit + 1) + lowerLimit);
- Instead of upper limit I placed max(ran1, ran2); function so that program gets higher number from the 2 numbers that user entered. Similarly, instead of lowerLimit, I placed min(ran1, ran2);
- Bunch of curly braces were placed incorrectly. So keep that in mind.
- I added parseInt in the isNaN parameter. I changed isNan(ran1) to isNaN(parseInt(ran1)) and did that for ran2 as well.
function max(ran1, ran2){
if (ran1 > ran2) {
return ran1;
} else {
return ran2;
}
}
function min(ran1, ran2) {
if (ran1 < ran2) {
return ran1;
} else {
return ran2;
}
}
var ran1 = prompt("please type a number");
var ran2 = prompt("please type another");
if (isNaN(parseInt(ran1)) || isNaN(parseInt(ran2))) {
alert("please reload and type a number");
} else {
alert(Math.floor(Math.random() * (max(ran1, ran2) - min(ran1, ran2) + 1) + min(ran1, ran2)));
}
I hope this helps. Carefully look through this code and make sure you understand it. Tell me if you have any questions and I will be happy to help you.