Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial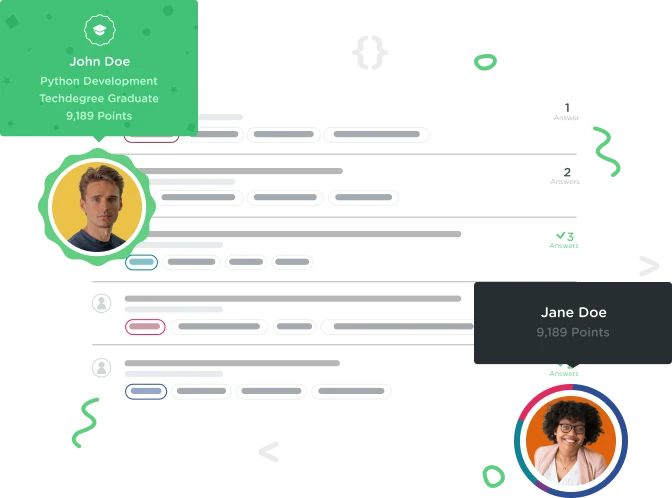
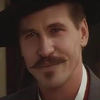
huckleberry
14,636 PointsTried something different and it's not working... can elements be created within an object literal?
Hello all you funky cold medina's out there...
Between the Appending & Removing Elements video and the one before it, Creating Elements, Chalkmeister makes the createNewTaskElement
function and in it, he does this.
var createNewTaskElement = function(taskString)
{
//create list item
var listItem = document.createElement("li");
//checkbox
var checkbox = document.createElement("input");
//label
var label = document.createElement("label");
//input (text)
var editInput = document.createElement("input");
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//Each element needs modifying
//all proper properties and attributes
//Each element need appending
listItem.appendChild(checkbox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
So, there I sit, looking over Andrew Chalkley 's code and I get to thinking and then I says to my myself... I says... "Hey Huck, that looks rather verbose and you are really trying to stick to nice and neat DRY code so why don't you group the variables up and ... oh, I know! It's all related to one thing so why don't you try sticking all that stuffs in an object and then use a for in
loop to append all that stuff??"
"Yeah!" I replied to myself with pride.
So then I reworked it so it looked like this...
var createNewTaskElement = function(taskString)
{
//create list item
var
listItem = document.createElement("li"), //list item
newTask = {
checkbox : document.createElement("input"), //checkbox
label : document.createElement("label"), //label
editInput : document.createElement("input"), //input (text)
editButton : document.createElement("button"),//button.edit
deleteButton : document.createElement("button")//button.delete
};
//Each element needs modifying
//all proper properties and attributes
//Each element need appending
for(element in newTask)
{
listItem.appendChild(element);
}
return listItem;
}
so this creates the listItem
first and then initializes an object literal with what I thought would be all the additional elements and then I thought the for in
loop would just run through that object and append all the elements.
The only problem is, it doesn't. It doesn't work and I get this...
Soooo ... wassup? Why can't I do this? It's definitely telling me that the parameter (element) I'm trying to give it as the thingy to append is in fact NOT a node so that tells me that the key values aren't being created. I'm obviously fairly new to working with the DOM and all its loveliness but, I dunno, it just seemed like something you could do. You can create key value pairs out of thin air just like you'd initialize a variable so why doesn't this work?
Is a method on the document object considered not a valid expression and therefore cannot be set as a value in an object?
Any help and insight as to why this won't work would be lovely and much appreciated.
Thanks all & cheers,
Huck -
1 Answer
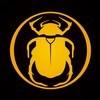
rydavim
18,814 PointsAs far as I know, there's no reason not to do it this way. Your for in
loop, however, is currently trying to append the key
rather than the value
. Since the key
is just a string
, you're getting that Node
error.
// Try running this to see what's going on:
for(element in newTask) {
console.log("The current element key: " + element);
console.log("The current element value: " + newTask[element]);
}
There are obviously other bits that need to be hooked up for everything to actually work, but hopefully that clears up your snag. Nice job looking for ways to make your code more concise. Happy coding!
huckleberry
14,636 Pointshuckleberry
14,636 Pointsahhhhh hahaha that's right! Thank you! I'd say DUH Huck! Buuuut tbh I haven't really mucked about and worked with objects and arrays as much as I should have after the initial lessons in order to really internalize some of the stuff to the point of just being able to mash it out without any thought like I should be able to.
I also need to cultivate more of a habit of debugging with the console in the manner that you did. Had I just tried to print out the results of the loop like you illustrated, even simply doing the first statement you did, it would've been apparent what the problem was.
Thanks for the comment on my refactoring there... I'm really trying to adhere to DRY and "elegant" code as it were yet still be fairly straightforward for human eyes.
Thanks a bunch dude!
Edit: Aww... someone else marked you as best answer. How did that even happen? Did they change permissions and allow others to do it?