Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial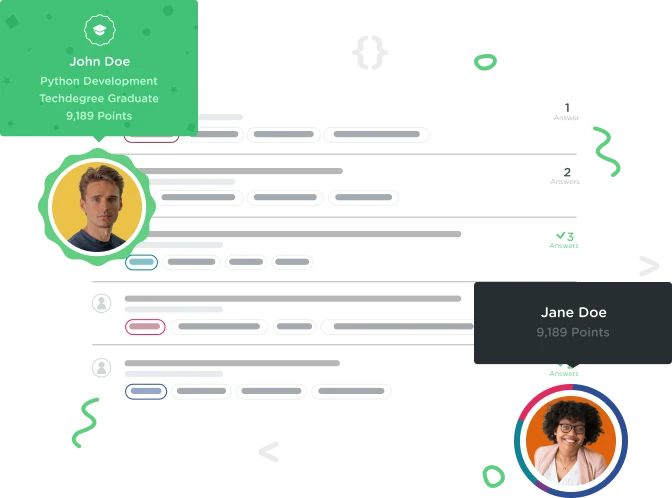

chrisshadrach
11,058 PointsTrigger Button Click on Enter
In short, I have a input field and a button. At the moment, someone can enter a number and click the button and everything works as I would expect (it takes their salary, breaks down the tax etc and displays it in a table). I am trying, with very little success, to have the same thing happen when someone hits enter instead of pressing the button. I had to set my button type to "button" in order to get everything to work and fear this is what is causing me issues. If I don't have it set that way, the data flashes in my table and doesn't stay, hence settling with "button" as the type. Any feedback would be appreciated.
The current HTML is as follows:
<form id="form">
<input type="number" id="salary" class="input" placeholder="Income before tax.." name="salary">
<button type="button" id="calculate">£ Calculate</button>
</form>
And the current JS:
//click button to call function
document.getElementById("calculate").onclick = function() {
calculateBreakdown();
}
//function runs when button is clicked
function calculateBreakdown() {
grossIncome = document.getElementById("salary").value;
// ... then a few other things happen ...
}
The code I found online that seems to work for everyone but me is as follows:
//press enter to call function
var input = document.getElementById("salary");
input.addEventListener("keyup", function(event) {
if (event.keyCode === 13) {
event.preventDefault();
document.getElementById("calculate").click();
}
});
Mod Edit: Fixed code formatting and added language syntax highlighting.
3 Answers

KRIS NIKOLAISEN
54,967 PointsStrange. It works with two inputs but not one. If you press Enter in the second box then gi=what was entered
will be logged. Here is the code:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<body>
<form id="form">
<input type="number" id="salary1" placeholder="Income before tax.." name="salary1">
<input type="number" id="salary" class="input" placeholder="Income before tax.." name="salary">
<button type="button" id="calculate">£ Calculate</button>
</form>
<script src="app.js"></script>
</body>
</html>
//click button to call function
document.getElementById("calculate").onclick = function() {
calculateBreakdown();
}
//function runs when button is clicked
function calculateBreakdown() {
grossIncome = document.getElementById("salary").value;
console.log("gi= " + grossIncome)
}
var input = document.getElementById("salary");
input.addEventListener("keyup", function(event) {
if (event.keyCode === 13) {
event.preventDefault();
document.getElementById("calculate").click();
}
});
With only one input I can see a flash in the console but it clears for some reason.
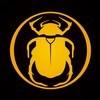
rydavim
18,813 PointsGiven what KRIS NIKOLAISEN discovered, my guess is it has something to do with default form submission behavior if there's only a single element? This is just a theory - but if you only have one input and you hit enter, the form assumes you're done. But if you have more than one element you might not be done, so it holds off on submission?
Given that what you're trying to prevent is the form submit, you could try binding the event to the form itself.
var form = document.getElementById("form"); // bind to form instead of input
form.addEventListener("keyup", function(event) {
if (event.keyCode == 13) {
event.preventDefault();
calculateBreakdown();
}
});
That worked for me, but obviously you'd need to tweak it if you have more than one element. Although it sounds like perhaps in that case, your original solution would work anyway.

chrisshadrach
11,058 PointsTry as I might, I just couldn't get it to work - sure I am missing something painfully obvious. For now, I am going to (maybe naively) assume it has something to do with the default form submission behaviours. In the end I got it to work by changing the tag from <form> to either a <div> or <section> and used the "keyup" method you both described. This worked a charm, although probably not the best way to do it. I didn't want to append form-data into the URL, which is what the form was doing on enter with "keyup".
I wish I knew why, but for now I will take the small victory and go back to learning. Appreciate both your help on this.