Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial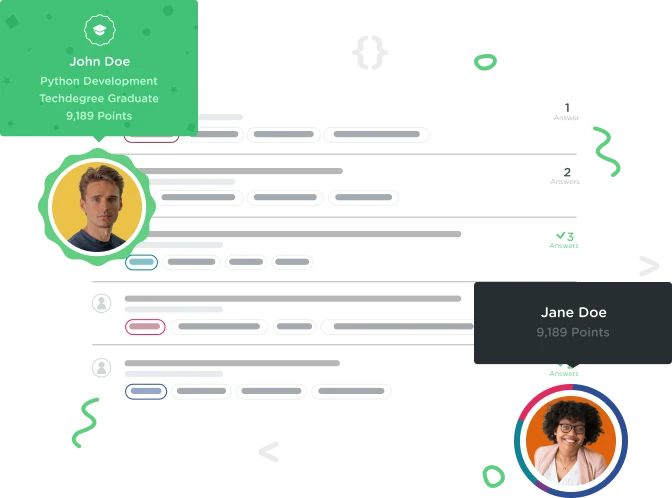

michael apprich
9,989 PointsTrouble coding a solution to duplicate names
Having trouble finding a solution to duplicate names. Anyone able to offer a hand and guide me in the right direction?
I'm not getting any console errors but the code doesn't seem to run past checking for an empty submission.
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
const li = createLI(text);
if (text === '') {
alert('Please Enter A Name');
} else {
const lis = ul.children
for (let i = 0; i < lis.length; i++) {
const registeredName = lis[i].firstElementChild.textContent;
if (text === registeredName) {
alert('That Name Has Already Been Added');
} else {
ul.appendChild(li);
}
}
}
});
4 Answers
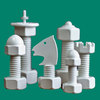
Steven Parker
229,744 PointsAdding to the list should not be done inside the loop. This prevents anything from being added when the list is still empty, plus it would add things multiple times otherwise. Instead, use the loop only to check for duplicates and return if any is found. Then, add the item after the loop:
for (let i = 0; i < lis.length; i++) {
const registeredName = lis[i].firstElementChild.textContent;
if (text === registeredName) {
alert('That Name Has Already Been Added');
return; // no need to check any more
}
}
ul.appendChild(li); // add only if loop finishes (without finding anything)

michael apprich
9,989 PointsWhere would this forum be without you, Steven? Thank you. I got a bit sloppy with my loops and completely overlooked the lack of return in the loop. I took the first if statement, added an else condition used it to append and put that after the for loop and it works how it should. Thank you, again! On to the next task!
here is the new code. might be useful to someone in the future:
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
const li = createLI(text);
const lis = ul.children;
for (let i = 0; i < lis.length; i++) {
const registeredName = lis[i].firstElementChild.textContent;
if (text === registeredName) {
alert('That Name Has Already Been Added');
return;
}
}
if (text === '') {
alert('Please Enter A Name');
} else {
ul.appendChild(li);
}
});
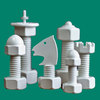
Steven Parker
229,744 PointsFunctionally, this would work just fine. But the original concept of checking for empty first has the advantage of saving the (admittedly small) CPU overhead of scanning the list when nothing was entered.

michael apprich
9,989 PointsSo would this be a more preferred and optimal way to do it:
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = input.value;
input.value = '';
const li = createLI(text);
const lis = ul.children;
if (text === '') {
alert('Please Enter A Name');
return;
}
for (let i = 0; i < lis.length; i++) {
const registeredName = lis[i].firstElementChild.textContent;
if (text === registeredName) {
alert('That Name Has Already Been Added');
return;
}
}
ul.appendChild(li);
});
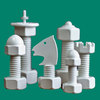
Steven Parker
229,744 PointsThe original "else" was fine, but the return is just as efficient.

michael apprich
9,989 PointsIt seemed that there was no real reason to keep the else so I just removed it.
Thank you again, Steven!