Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial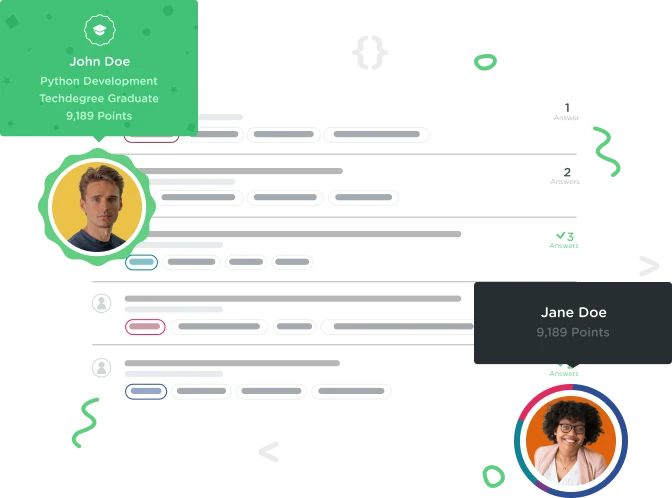
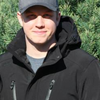
Tyler Chapman
20,470 PointsTrouble connecting weather app and retrieving JSON data?
I have tried testing my Stormy weather app on a device(connected via wifi not network) and the emulator in android studio. Neither way allows me to see the JSON data that Ben can see at the end of the video. When I load the app up in the emulator I do get the "Network is unavailable!" toast. If anyone has any ideas I'd love the advice.
public class MainActivity extends ActionBarActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "0480430260d54cff60991a1ab0cf22c2";
double latitude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecase.io/forcast/" + apiKey + "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(forecastUrl).build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
}
else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});}
else {
Toast.makeText(this, getString(R.string.network_unavalable_message), Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running!");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
return new CurrentWeather();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
public class AlertDialogFragment extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
Context context = getActivity();
AlertDialog.Builder builder = new AlertDialog.Builder(context)
.setTitle(context.getString(R.string.error_title))
.setMessage(context.getString(R.string.error_message))
.setPositiveButton(context.getString(R.string.error_ok_button_text), null);
AlertDialog dialog = builder.create();
return dialog;
}
}
public class CurrentWeather {
private String mIcon;
private long mTime;
private double mTemperature;
private double mHumidity;
private double mPrecipChance;
private String mSummary;
public String getIcon() {
return mIcon;
}
public void setIcon(String icon) {
mIcon = icon;
}
public long getTime() {
return mTime;
}
public void setTime(long time) {
mTime = time;
}
public double getTemperature() {
return mTemperature;
}
public void setTemperature(double temperature) {
mTemperature = temperature;
}
public double getHumidity() {
return mHumidity;
}
public void setHumidity(double humidity) {
mHumidity = humidity;
}
public double getPrecipChance() {
return mPrecipChance;
}
public void setPrecipChance(double precipChance) {
mPrecipChance = precipChance;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
}
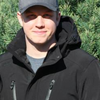
Tyler Chapman
20,470 PointsI only get the toast on the emulator. On my device I get through to the Internet in my other apps but my weather app doesn't seem to be connecting.
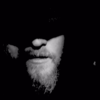
Micah Kline
17,831 PointsDo you see any data in Log-Cat. It would only toast if there was an error. Sounds like a perfect opportunity to set up break points in the code.
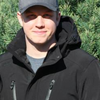
Tyler Chapman
20,470 PointsI do get the log message that the main ui is running but that is it, I will try out using some break points.
3 Answers
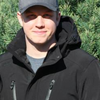
Tyler Chapman
20,470 PointsI feel pretty dumb but I had a typo in my forecastURL variable.
String forecastUrl = "https://api.forecase.io/forcast/" + apiKey + "/" + latitude + "," + longitude;
Once I corrected it I was able to connect. Thanks for all the help.
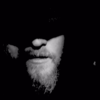
Micah Kline
17,831 PointsLook for the timezone in the log cat its the only thing I see you are saving at the moment
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
return new CurrentWeather();
}

James Simshaw
28,738 PointsHello,
You might want to check what your logcat filtering level. I'm going to guess its set at Debug since you are able to see the "Main UI code is running" message but none of the others. I would recommend setting it to Verbose since you are outputting some messages with the Log.v() method.
James Simshaw
28,738 PointsJames Simshaw
28,738 PointsHello,
On the device you tested, were you able to access the internet at all? The fact that you got the toast message leads me to believe that there's something wrong with your network connection.