Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial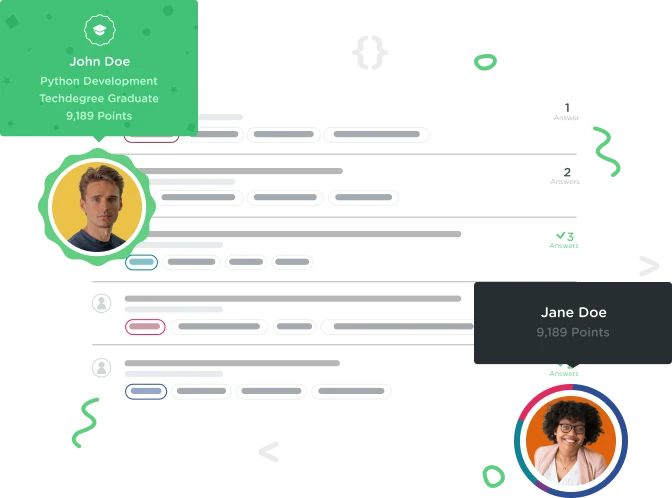

Tony Lawrence
3,056 PointsTrouble Finding the Average Tongue Length for Frogs For Loop
Hi everyone,
I'm having trouble trying to figure out how to get the challenge. So far I have this:
namespace Treehouse.CodeChallenges { class FrogStats {
public static double GetAverageTongueLength(Frog[] frogs)
{
double sum = 0;
for (int i = 0; i < frogs.Length; i++)
{
Frog sum = frogs[i];
}
return sum / frogs.Length;
}
}
}
I get this error message: FrogStats.cs(13,22): error CS0136: A local variable named sum' cannot be declared in this scope because it would give a different meaning to
sum', which is already used in a `parent or current' scope to denote something else
Compilation failed: 1 error(s), 0 warnings
The error is the same if I put the return line inside the for loop and if I remove 'Frog' before sum within the for loop. If I were to change the for loop to this:
double sum = 0;
for (int i = 0; i < frogs.Length; i++)
{
sum = frogs[i];
}
return sum / frogs.Length;
}
}
}
I get a error CS0029: Cannot implicitly convert type Treehouse.CodeChallenges.Frog' to
double'
I'm not sure what to do in order to correct this issue and give the challenge's answer. Thoughts?
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer

andren
28,558 PointsThe problem in your first example is that you are declaring the sum variable twice and the second time you declare it as a different data type, that is not allowed in C#.
In the second example the problem is that the frogs array is not an array of numbers, it is actually an array of frog objects, so frogs[i] will actually result in a singular Frog object, which is not something that can be stored in a double variable.
If you look at the file for the frog class which is available in the challenge workspace you will see that it has a property called TongueLength, this is where the tongue length of the frog is actually stored.
This means that you have to access it using dot notation like this: frogs[i].TongueLength
In addition to that there is a second problem in your loop which is the fact that you are setting sum equal to the frog object, rather than adding the frog object to it, this means that each time though the loop the sum variable is replaced by the contents of the current frog object rather than having the contents added to sum.
To fix that you have to use the += symbol (which adds the content on the right to the variable on the left) rather than the = symbol (which completely replaces the contents of the variable with the value on the right).
After applying those two fixes to your code you end up with this code:
double sum = 0;
for (int i = 0; i < frogs.Length; i++)
{
sum += frogs[i].TongueLength;
}
return sum / frogs.Length;
Which does what the challenge asks for.
Tony Lawrence
3,056 PointsTony Lawrence
3,056 PointsThank you. I got that answer about the error codes while looking up for means to solve the solution, but the examples given are confusing. I sometimes dislike having to ask these questions when it comes to these challenges, since I would rather figure out the problem I got myself stuck in. Thank you again for your help.