Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial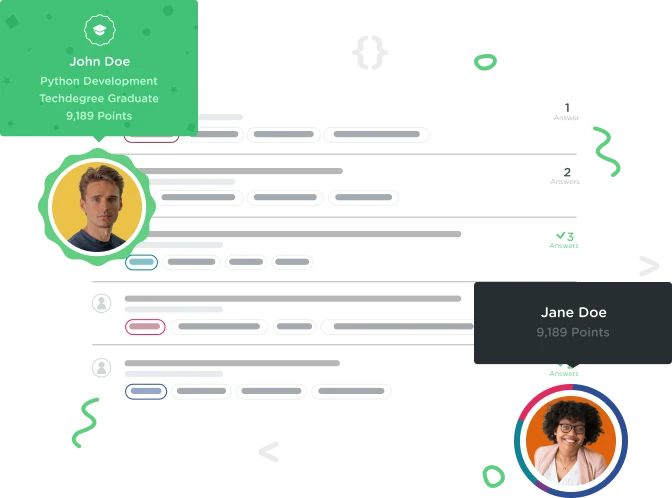
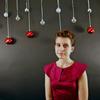
teddy waggy
3,486 PointsTrouble generating HTML in JS
Hi! Okay. So. I'm building a simple calendar to practice JS, and I'm having a lot of trouble getting it to display properly. Specifically, I cannot get the table to take on a colspan attribute. Instead it will just display my days of the week in rows on top of each other. I've tried using the .setAttribute() method a few different ways, I've tried putting it in every piece of HTML it might go in, and I've tried putting it in the separate HTML file as well. No cigar. Here's the code I have so far for this. If anyone has any ideas or suggestions, that would be great. I also just read that using the .createElement or TextNode and .appendChild methods are preferable to the .innerHTML that I'm using. Could that have anything to do with it?
//Create your day, month and month-length arrays, and a new date
//with some variables to latch onto pieces of that date
var daysArray = ['Monday', 'Tuesday', 'Wednesday', 'Thursday',
'Friday', 'Saturday', 'Sunday'];
var monthsArray = ['January', 'February', 'March', 'April', 'May',
'June', 'July', 'August', 'September',
'October', 'November', 'December'];
var monthLengthArray = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31];
var currentDate = new Date();
var currentDay = currentDate.getDay();
var currentMonth = currentDate.getMonth();
var currentYear = currentDate.getFullYear();
//Set a variable to grab onto your calendar table
var calendar = document.getElementById("calendar");
//Build your calendar table
calendar.innerHTML = '<tr><th>';
calendar.innerHTML += monthsArray[currentMonth] + ' ' + currentYear;
calendar.innerHTML += '</th></tr><tr>';
//Make a row showing the days of the week
for (var i = 0; i < daysArray.length; i++) {
calendar.innerHTML += '<td>' + daysArray[i] + '</td>';
}
calendar.innerHTML += '</tr>';
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Teddy,
I think your problem is that you're repeatedly setting the innerHTML
property with incomplete html as you're trying to build up all of the html. Each time, the browser then tries to fix this incomplete html by closing off tags for you. I had pasted your js in a codepen and noticed that the browser had created multiple tbody
tags around each row for example.
You should define a new variable, something like var html;
and then build up your html string while storing it in that variable. Then at the end you can set the innerHTML
property one time to the value you have in your variable.
So replace all calendar.innerHTML
with html
and then at the end you could add calendar.innerHTML = html;
By making this change you should at least start getting the html output that you're expecting.
I realize this is primarily a javascript exercise for you but I would recommend you make some changes to your html. The month/year title would probably be more suitable in a caption
element for the table rather than as a table heading. I've also seen it in a heading element above the table.
The days of the week can then be the table headings. Currently you have them in td's which doesn't distinguish them from the later td's you'll have with the days of the month.
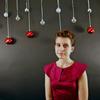
teddy waggy
3,486 PointsThank you, this is extremely helpful! And any input, HTML or JavaScript, is great, I want to be the queen dungeon master overlord of both. Thanks for both!

Jason Anello
Courses Plus Student 94,610 PointsAnd css too, right? :)
I thought I would give you some sample html for how you could markup your calendar. This way you can kind of use it as a reference and you can compare the output from your javascript and see how it compares to this sample. Of course, this is just one way to markup a calendar and opinions are going to vary on this.
<table>
<caption>April 2014</caption>
<thead>
<tr>
<th>Sunday</th>
<th>Monday</th>
<th>Tuesday</th>
<th>Wednesday</th>
<th>Thursday</th>
<th>Friday</th>
<th>Saturday</th>
</tr>
</thead>
<tbody>
<tr>
<td></td>
<td></td>
<td>1</td>
<td>2</td>
<td>3</td>
<td>4</td>
<td>5</td>
</tr>
<!-- The rest of the weeks here -->
</tbody>
</table>
I've found that at least firefox will add tbody tags for you if it doesn't find them and it may place them around all your rows including your day of the week headings. So I feel it's better to put your thead, tbody, and tfoot (if applicable) in yourself instead of relying on the browser's best guess.