Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial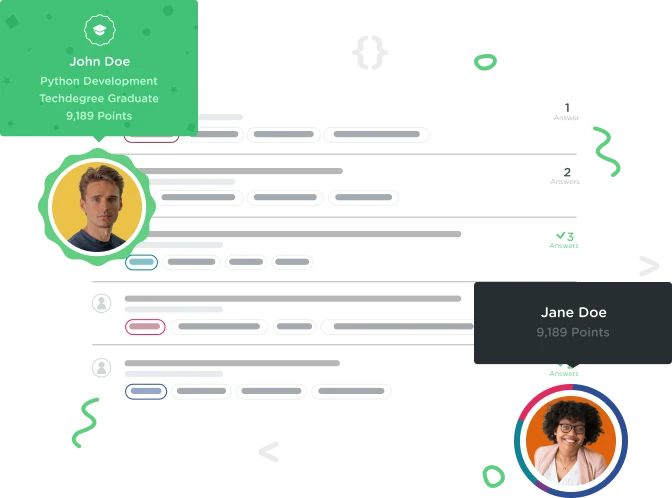
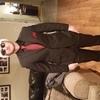
Daniel Hulin
1,852 PointsTrouble getting return.
What part of the code is missing? I belive my trouble is understanding what "method" I should be using?
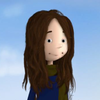
Maximiliane Quel
Courses Plus Student 55,489 PointsWe can't really see your code. Can you please copy paste what you tried?
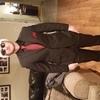
Daniel Hulin
1,852 PointsI apoligise. I thought I had posted my answer. Thanks everyone for the help.
3 Answers
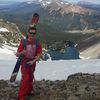
Ethan Weeks
15,352 PointsIn this is challenge you could have written it with two return methods like this:
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50
return "safe"
else
return "unsafe"
end
end
or with just one return method like this:
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && car_speed <= 50
result = "safe"
else
result = "unsafe"
end
return result
end
I personally like to keep the return statement to a minimum by assign each option to a variable and returning the variable. Both ways are right! I hope that helps!
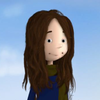
Maximiliane Quel
Courses Plus Student 55,489 PointsHi,
if you are still having trouble passing the challenge, please post your code, so we can identify what exactly is wrong with it.
@Ethan: You do not have to use the return keyword as ruby automatically returns the last line that gets run. Either the car speed is in between the boundries or it is not. Whichever case it is only one of the strings is returned. So you do not need to return anything outside of the if else block.
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && 50 >= car_speed
"safe"
else
"unsafe"
end
end

andi mitre
Treehouse Guest TeacherI personally would recommend to use the return statement at is good practice and very commonly used among other languages. Thus, I would go with @Ethan's first answer but any of the ones provided are correct.
Cheers
def check_speed(car_speed)
# write your code here
if car_speed >= 40 && 50 >= car_speed
return "safe"
else
return "unsafe"
end
end
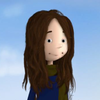
Maximiliane Quel
Courses Plus Student 55,489 Pointsyou are right that an explicit return makes it more visible that we are returning something. :0)
I was merely referring to Ethan's wish to keep the return statements to a minimum. in which case leaving the return out in the first place would probably be the way to go. with such a short statement it would probably be safe to do so don't you think?
I still feel we haven't answered the original question though ...
andi mitre
Treehouse Guest Teacherandi mitre
Treehouse Guest TeacherDaniel you haven't posted any code