Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial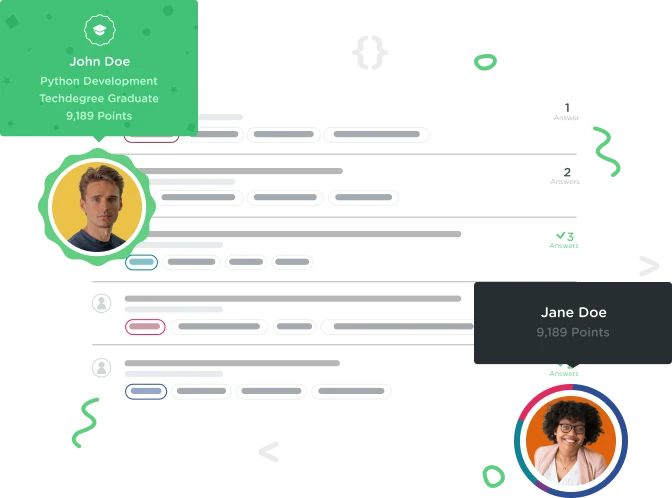

Caleb Austin
1,408 PointsTrouble in this objective
I am struggling with figuring out how to successfully approach this objective. Any help would be much appreciated. Thanks!
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
public void drive() {
lapsDriven == 0;
lapsDriven++;
}
}
}
1 Answer
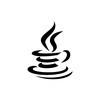
John Anselmo
Courses Plus Student 2,281 PointsI noticed you finished task 1/3 based on you already having your drive() method.
For task 2/3 : Great! Now let's write a simple drive method. It should be public and not return anything. We'll start out basic, calling the drive method will make the GoKart drive a single lap.
In your newly created drive method, increment the new lapsDriven
variable by 1. Use the incrementing shorthand to increase lapsDriven
.
What you want to do is take away the lapsDriven == 0;
that you have in your drive()
method because whenever you call drive()
it resets the lapsDriven
variable to 0, and you don't want that. So just having:
public void drive(){
lapsDriven++;
}
is fine for task 2/3.
Now for task 3/3: Each lap around the track takes exactly 1 energy bar from the battery.
For this final task, in your drive method, decrement the battery's status that we maintain in the private field barCount.
Take your newly fixed code from task 2/3 and add barCount--;
to the drive()
method because the task wants you to subtract 1 from barCount
whenever you complete a lap. So your drive method should now look like this:
public void drive(){
lapsDriven++;
barCount--;
}
annapoff
13,552 Pointsannapoff
13,552 Pointsi'm following along here! My 1st task passes, then when i enter this data:
public void drive(){ lapsDriven++; }
it gives me a fail: "Oops! It looks like Task 1 is no longer passing."
https://teamtreehouse.com/library/java-objects-2/harnessing-the-power-of-objects/increment-and-decrement