Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial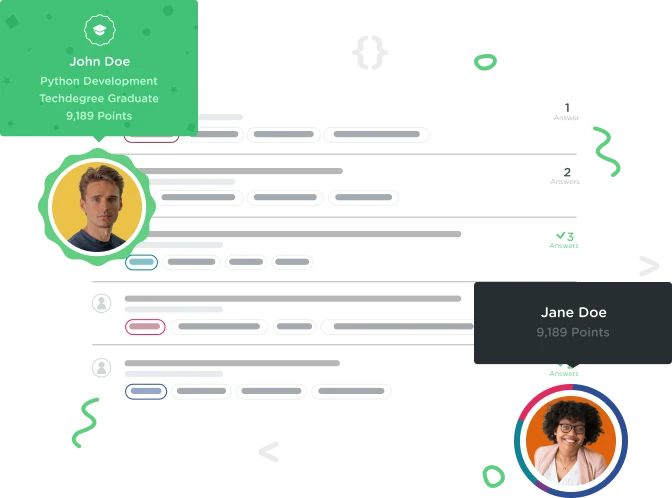

Thomas Katalenas
11,033 Pointstrouble returning
func greeting(person:String) -> String{
let language = "English"
let greeting = "Hello \(person)"
let tup = (greeting, language)
print(tup)
return greeting
}
greeting("tom")
I get the basic idea and this returns, ("Hello Tom", "English")
but I cant return (greeting, language) or I get this error
tmp/3wXV2YS6fa.m:8:12: error: cannot convert return expression of type '(String, String)' to return type 'String'
func greeting(person: String) -> String {
let language = "English"
let greeting = "Hello \(person)"
let tup = (greeting, language)
print(tup)
return greeting
}
4 Answers
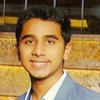
Anish Walawalkar
8,534 PointsYou're almost there. You just need to change your function definition to show that it is now going to return a tuple not a string. I also did the remaining part of the challenge:
so in code:
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
var result = greeting("Tom")
println(result.language)

Thomas Katalenas
11,033 Pointsoh I was wondering about that lol
func greeting(person: String) -> (String,String) {
let greeting = "Hello \(person)"
let language = "English"
let tup = (greeting, language)
print(tup)
return tup
}
like that?
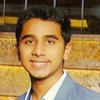
Anish Walawalkar
8,534 PointsYeah thats perfect, just remove the print. also just a just a quick note:
func greeting(person: String) -> (greeting: String, language: String) {
let greeting = "Hello \(person)"
let language = "English"
let tup = (greeting, language)
print(tup)
return tup
}
if you name your return values in the return tuple, like I have you can access them like this:
var result = greeting("Tom")
print(result.greeting)
print(result.language)
However if you do not name them, then you will have to access them like this:
var result = greeting("Tom")
print(result.0) //to print the greeting
print(result.1) //to print the language
Hope that helps

Thomas Katalenas
11,033 Pointsis that faster, greeting("Tom").1
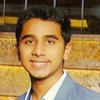
Anish Walawalkar
8,534 PointsNo, its takes the same time to execute (as far as I can tell)

Thomas Katalenas
11,033 Pointsfor i in 0..2{ //or stream of 1's and 0's
let a: int = i
var m = greeting("John").a
print(m)
}
Anish Walawalkar
8,534 PointsAnish Walawalkar
8,534 Pointsdon't know why the -> isn't showing up properly lol