Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial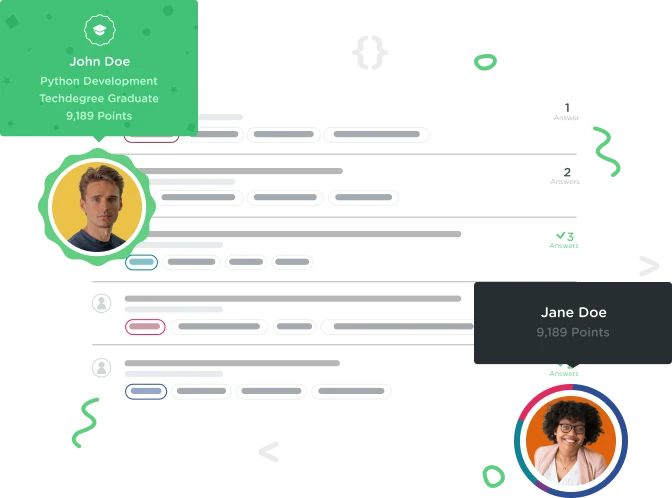

Robert Kendall
1,287 PointsTrouble setting up a getter method
Having trouble with this challenge- I understand FIREFLY is a string, not a variable, but not sure what parameters need to be passed to the getter.
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends Activity {
public Button mThrustButton;
public TextView mTypeLabel;
public EditText mPassengersField;
public Spaceship mSpaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
mThrustButton = (Button)findViewById(R.id.thrustButton);
mTypeLabel = (TextView)findViewById(R.id.typeTextView);
mPassengersField = (EditText)findViewById(R.id.passengersEditText);
// Add your code here!
mSpaceship = new Spaceship("FIREFLY");
public String getTextView() {
return mTypeLabel;
}
}
}
public class Spaceship {
private String mType;
private int mNumPassengers = 0;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public int getNumPassengers() {
return mNumPassengers;
}
public void setNumPassengers(int numPassengers) {
mNumPassengers = numPassengers;
}
public Spaceship() {
mType = "SHUTTLE";
}
public Spaceship(String type) {
mType = type;
}
}
1 Answer
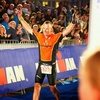
Steve Hunter
57,712 PointsHi Robert,
You're nearly there.
First, you've correctly assigned a new Spaceship
object and used the constructor to call it "FIREFLY".
Next, we want to set the TextView
property which is called mTypeLabel
. That is a member variable of the LandingActivity
. This variable holds the type of Spaceship, as its name suggests. This is where we use the "getter" method. A Spaceship has a getter to return the type of Spaceship. It is called getType()
and is a method of all Spaceship objects, like mSpaceship
that you just created. We can use the method to return the type by using dot notation: mSpaceship.getType()
will return a String; "FIREFLY" in this case.
To set the mTypeLabel
, we need to use a method of the TextView
class called setText()
. This is used with dot notation again. The method setText()
takes a string as a parameter; that is what the getType()
method returned, right?
This means we can chain all that together:
mTypeLabel.setText(mSpaceship.getType());
This sets the mTypeLabel
to the string returned by the Spaceship's getType()
method.
Make sense?
Steve.
Robert Kendall
1,287 PointsRobert Kendall
1,287 PointsThanks for the detailed explanation. I now understand it, but still would have a problem coming up with it on my own! Practice, practice, I guess.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHey, no problem.
Yes, more practice and familiarity and you'll be fine. I learned by just trying, messing up, asking questions and repeating that process!
Shout if you need help; happy to lend a hand where I can.
Steve.