Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial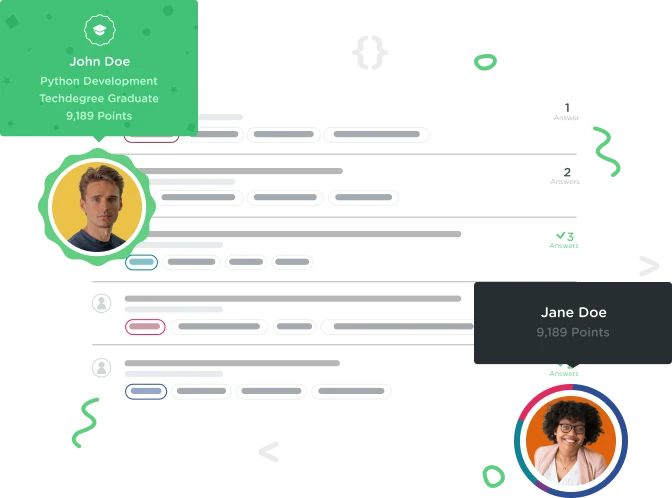
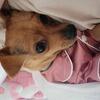
shiny peanut
5,113 PointsTrouble throwing exceptions
Am I trying to do more than it is asking for? Every time I try to finish this course, I get stuck. I really don't get it and this course is moving too fast for me to fully get each new concept back to back. If you can, please really break this problem down because I do not understand it at all.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
}
int newAmount = barCount + laps;
if (newAmount < 0) {
throw new IllegalArgumentException("Not enough bars!");
}
lapsDriven += laps;
barCount -= laps;
}
}
4 Answers
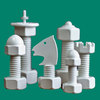
Steven Parker
230,274 PointsYou're actually pretty close! You have a few duplicated lines and a math error:
public void drive(int laps) {
// lapsDriven += laps; <- these lines are copies of the ones below
// barCount -= laps; <- I have commented them out here but
//} <- they can be deleted
int newAmount = barCount + laps; // SUBTRACT the laps
// barCount - laps <- instead of adding them
if (newAmount < 0) {
throw new IllegalArgumentException("Not enough bars!");
}
lapsDriven += laps;
barCount -= laps;
}

Seth Kroger
56,413 PointsThe code you wrote you should work. It's just outside the closing brace of the drive method so it's considered a syntax error. If you remove the first 3 line lines it should be fine.
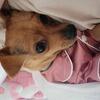
shiny peanut
5,113 PointsI assume by "the first three lines," you mean lapsDriven += laps; barCount -= laps; and int newAmount = barCount + laps;? I removed them and it still doesn't work and I still don't get what is happening with the code regardless.
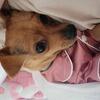
shiny peanut
5,113 PointsThank you! That was super helpful