Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial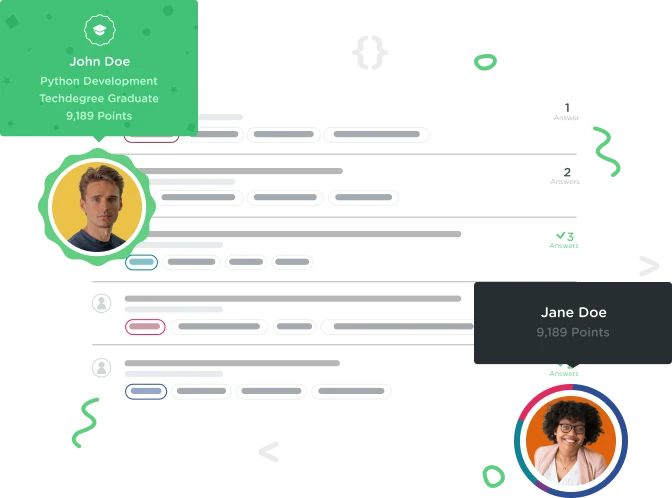

Brian Maimone
Courses Plus Student 1,644 PointsTrouble understanding how nested for loop and counting work. Appreciate help.
Looking at the creation of the Map interface and the nested for loops: Line 33, the second for statement, isn't treet.getHashTags() an ArrayList of words with hash tag prefixes. Each treet calls the getHashTags() method which returns the List of words with hash tags contained in the treet?
Don't understand how next line works, 34. Does hashTagCounts.get(hashTag) pull out each word with a hashtag in front? How does variable count take on a null value? Is Integer automatically switching to an object wrapper type?
Why check if count == null if getHashTags() above is a list of words with the hashtag prefix?
public class Example {
public static void main(String[] args) { Treet[] treets = Treets.load(); System.out.printf("There are %d treets. %n", treets.length); Set<String> allHashTags = new HashSet<String>(); Set<String> allMentions = new TreeSet<String>(); for (Treet treet : treets) { allHashTags.addAll(treet.getHashTags()); allMentions.addAll(treet.getMentions()); } System.out.printf("Hash tags: %s %n", allHashTags); System.out.printf("Mentions: %s %n", allMentions);
//Make key be hashtag, value be count so we can see what most popular hashtags were
Map<String, Integer> hashTagCounts = new HashMap<String, Integer>();// key type is String, value is Integer, Note values must be of type object
//Uppercase Integer will toggle to type int automatically - autoboxing
for (Treet treet : treets) { //for each treet in treets
for (String hashTag : treet.getHashTags()) {//loop through each hashtag, .getHashTags,of a specific treet
Integer count = hashTagCounts.get(hashTag);//see if it exists
if (count == null) {
count = 0;
}
count++;
hashTagCounts.put(hashTag, count);
}
}
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
Map<String, List<Treet>> treetsByAuthor = new HashMap<String, List<Treet>>();
for (Treet treet : treets) {
List<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
if (authoredTreets == null) {
authoredTreets = new ArrayList<Treet>();
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
}
authoredTreets.add(treet);
}
System.out.printf("Treets by author: %s %n ", treetsByAuthor);
System.out.printf("Treets by nickrp: %s %n", treetsByAuthor.get("nickrp"));
// Treet originalTreet = treets[0];//pullout first one, there in sorting order // System.out.println("Hashtags:"); //for (String hashtag : originalTreet.getHashTags()) { // System.out.println(hashtag);
}
}
package com.teamtreehouse;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
public class Treet implements Comparable, Serializable { private static final long serialVersionUID = 7146681148113043748L; private String mAuthor; private String mDescription; private Date mCreationDate;
public Treet(String author, String description, Date creationDate) { mAuthor = author; mDescription = description; mCreationDate = creationDate; } @Override public String toString() { return String.format("Treet: \"%s\" by %s on %s", mDescription, mAuthor, mCreationDate); } @Override public int compareTo(Object obj) { Treet other = (Treet) obj; if (equals(other)) { return 0; } int dateCmp = mCreationDate.compareTo(other.mCreationDate); if (dateCmp == 0) { return mDescription.compareTo(other.mDescription); } return dateCmp; } public String getAuthor() { return mAuthor; } public String getDescription() { return mDescription; } public Date getCreationDate() { return mCreationDate; } public List<String> getWords() { String[] words = mDescription.toLowerCase().split("[^\w#@']+"); return Arrays.asList(words); } public List<String> getHashTags() {//return a list of strings calling it gethashtags return getWordsPrefixedWith("#"); } public List<String> getMentions() {//make a list of mentions, return a list of strings return getWordsPrefixedWith("@"); } private List<String> getWordsPrefixedWith(String prefix) {//always want return list interface,return a list of strings List<String> results = new ArrayList<String>(); //make a new list called results, its a new arraylist for (String word : getWords()) {// loop through array, check each word in the treet,to see if it has hashtag if (word.startsWith(prefix)) {//startsWith is special method of strings results.add(word); } } return results; } }
2 Answers
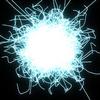
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsGood afternoon Brian,
Basically what we are doing in that code is checking to see each HashTag word in our treets. So that we can determine which one is the most often used.
We use the code
Integer count = hashTagCounts.get(hashTag);//see if it exists
if (count == null) {
count = 0;
Because in the event that we have never come across that word as a hashTag before, we need to create a new count for it, as we can't increase the count if it hasn't origionally been created.
Next each time we across the word we implement the count by one. and update the map with the hashtag phrase, and count.
Thanks, I hope this helps. Shoot back if it doesn't.

Shariq Shaikh
13,945 PointsI get it now, thanks.
Shariq Shaikh
13,945 PointsShariq Shaikh
13,945 PointsWhat type of object is hashTagCounts.get(hashTag) returning? Does is just return a object of type Object and in this case, casts the object to type Integer?
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey There Shariq, the name is confusing but it's actually just returning the interger value that we put as the value's into our map below.
Map<String, Integer> hashTagCounts = new HashMap<String, Integer>();
What we're doing is going through each item in the map, if we've come across the hash tag before, we just that reference to the map as hash tag, and increase the count.
But in the event that it's not a word, we create a new key in the map, and set the value to 1.
So essentially all that it is doing is checking in our map to see first, if the hash tag was ever used, and second how many times it was used.
Thanks, hope this helps.