Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial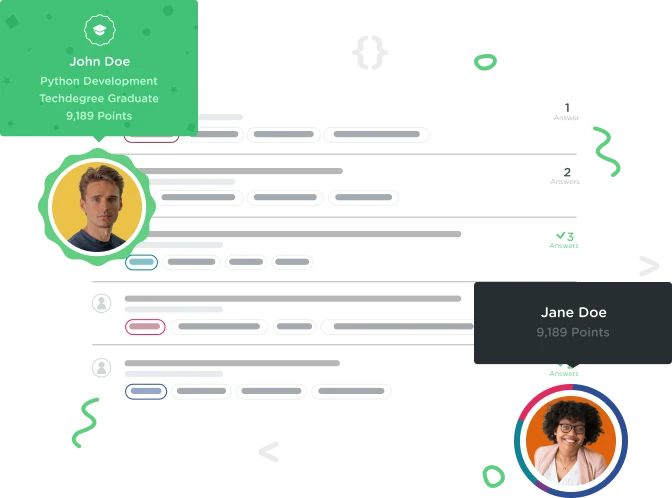

Anthony Nguyen
3,214 PointsTrouble understanding how to complete this quiz
I'm having trouble how to get the code to list the first 10 multiples of 6. Should the code I have entered already list the first 10? Thanks for any help.
Sincerely, A newbie swift student
// Enter your code below
var results: [Int] = []
for results in 1...10{
print(results * 6)
}
for multiplier in results {
print(results)
}
2 Answers
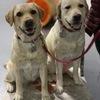
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Anthony,
This line:
var results: [Int] = []
Declares the results
variable. Its type is being explicitly declared as an array of Ints. You might be used to declaring your variables like this:
var my_variable = [1, 2, 3]
And letting the compiler infer the type for you (which is the recommended practice by Apple). However, when declaring an empty array, it's impossible for the compiler to infer the type inside the array so you are required to explicitly declare the type.
On the right side of the assignment operator, the square brackets are just an empty array.
So, when we start your for loop, we have an empty Int array called results
sitting there, waiting for values to be added to it.
Now let's look at your for loop:
for multiplier in 1...10 {
(multiplier * 6)
}
Like the challenge asks, you are iterating through the range 1 to 10 and using a temporary constant called multiplier
.
Then, also like the challenge asks, you are calculating the value of multiplier
times 6. But then you do nothing with this value. The challenge says:
Once you have a value, append it to the results array.
Let's look back at my previous answer to see how to append a value to an array:
If you have forgotten the syntax for appending an element to an array, here is what the official documentation says:
Adding and Removing Elements
...
To add single elements to the end of an array, use the append(_:) method.
...
students.append("Maxime")
In Apple's example, they are appending a string "Maxime"
to an array called students
. Since the value you want to append is multiplier * 6
you should replace "Maxime"
with multiplier * 6
. Your array is called results
so you should replace students
with results
.
Hope that's clear.
Cheers
Alex
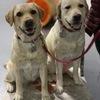
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Anthony,
For part 1 of the challenge you were tasked with defining one for in
loop with the following characteristics:
- iterate over a range that goes from 1 to 10;
- name the temporary constant
multiplier
- leave the body of the loop empty
You've correctly defined a range that goes from 1 to 10 (inclusive).
You've also correctly named a temporary constant in one of your loops multiplier
.
However, you've created two loops, when only one was asked for. You weren't asked to create a loop with a temporary constant called results
nor were you asked to create a loop with a range of results
.
If you reduce your code to just the parts that were asked for, you will have the correct foundation for going into part 2 of the challenge.
When you get to part 2 of the challenge you will be entering the code into the one (and only one) for loop:
Inside the body of the loop,
The code that you enter inside the loop will perform one calculation: multiply the value in the temporary variable (named multiplier
) by 6:
we're going to use the multiplier to get the multiple of 6
Then you need to append that value to the array called results
.
Once you have a value, append it to the results array.
If you have forgotten the syntax for appending an element to an array, here is what the official documentation says:
Adding and Removing Elements
...
To add single elements to the end of an array, use the append(_:) method.
...
students.append("Maxime")
Note that the challenge instructions are only asking you to modify the contents of the supplied results
array. You are not being asked to print anything.
Hope this clears things up for you.
Cheers
Alex

Anthony Nguyen
3,214 PointsThank you Alex. This clears up a lot for me as I was going into the second step while my first answer was not entirely correct. This is what I have now:
var results: [Int] = []
for multiplier in 1...10 { (multiplier * 6) }
Now, I'm trying to understand how to modify the results array with a value. When I look at the results array, I see [Int] = []. Which doesn't make any sense to me. I am obviously missing something and I can't wrap my finger around it. Thanks for the help.
Anthony Nguyen
3,214 PointsAnthony Nguyen
3,214 PointsAlex, thanks so much for your guidance. You've explained it perfectly and helped me understand this concept. Thanks again.
ewa boer
648 Pointsewa boer
648 PointsI did it and it doesn't work results.append(multiplier * 6) is that wrong? Cheers Ewa