Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial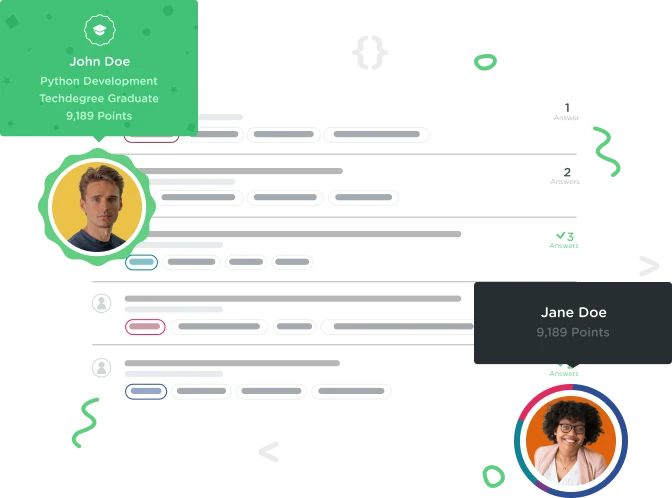

Arthur Podkowiak
3,633 PointsTrouble understanding Maps
When we were first introduced to Maps in the Java Data Structures course, we had this code:
Map<String, Integer> hashTagCounts = new HashMap<String, Integer>();
for (Treet treet : treets) {
for (String hashTag : treet.getHashTags()) {
Integer count = hashTagCounts.get(hashTag);
if (count == null) {
count = 0;
}
count++;
hashTagCounts.put(hashTag, count);
}
}
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
Map<String, List<Treet>> treetsByAuthor = new HashMap<String, List<Treet>>();
for (Treet treet : treets) {
List<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
if (authoredTreets == null) {
authoredTreets = new ArrayList<Treet>();
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
}
authoredTreets.add(treet);
}
System.out.printf("Treets by author: %s %n", treetsByAuthor);
System.out.printf("Treets by nickrp: %s %n", treetsByAuthor.get("nickrp"));
I'm having a little trouble with it and I will specify where exactly and why.
1) At the line
Integer count = hashTagCounts.get(hashTag);
if (count == null) {
count = 0;
}
count++;
I understand this is creating a counter just to count how many hash tags of the same kind are there. How come we create a new variable count
using Integer
? I know count will be an integer, and I briefly remember Craig stating when using generics we use the uppercase full wording of the primitive, but this isn't a generic as it isn't surrounded by <>
. Also, how is this even counting? It creates an integer object and then asks it to get the hash tag back from the Map being created. And thirdly with this set of code, how can we call the newly made hashTagCounts
object within itself?
2) I actually am starting to get it a little by rereading the code as I type this question, but I will continue. For the second part of the code referring to the Map treetsByAuthor
, this has helped me to see that List<Treet> authoredTreets
is creating a new object to be placed in that portion of the Map, where it will essentially make the value in the Map equal to the author of the Treet.
Now the "if" statement starts. It says that if there is no author found in a Treet, it creates a new list of Treet objects. Why do we have to create a new list? Don't we have our Map which is creating a list of Treets? This is getting confusing for me. It would also help if someone can walk through the rest of that code and exactly what it is doing as well.
Any help is MUCH appreciated! Just trying to understand this stuff better so I don't explode when I look at it. Thanks!
Also: how do I make my code not grey, but colored like it is in the Workspace?
2 Answers
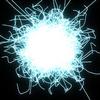
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey there Arthur,
What this code is doing is running through our getHashTags() method, which if we remember right returned every word that started with a Hashtag, with that being clear let's go through this step by step.
What we're first doing is we're setting the variable to getHashTags() by the key value, so, let's say we start this thing out and our hashes are simple #a #b #c #d #e #a #a #b #c
So let's run through our code.
it first starts count by pulling the first hash in getHashSet, which in our case is #a, so it it sets that to null at first.
Thankfully we gave it code that if count is null, set it to zero.
So, since it found #a by going through each of the strings, it sets the count to 0, then moves it to 1 the next time over.
It would do this the same for every thing in the string, moving right along to b,c,d,e, which would all have a value set to 1.
Pretty straight forward.
However it reaches #a again, Well it already has #a with a count of 1 because it found it before, well it's not null so it skips that portion of our code and just increments the count to 2, until at the end #a's count is 3, #b is 2, and #c is 2.
With all that being said, we set count to hashTagCounts.get(hashTag); because we need to set the count to how many times we can get the get the key from map, or the hashTag.
Moving onto the Treet. What we're basically doing in this is setting a List to contain each Treet by each author, we do have a map that lists the treets, yet we want to catagorize by author as well, so that we can see each unique Treet by that author, which is exactly what we are doing.
So what that statement is doing is much of the same as the previous one, it is going through each Treet that we have, and it creats a list for each Author if one hasn't been created yet, otherwise it just adds that specific Treet to that authors list.
That whole method is just a better way to organize out Treets, so that we could do exactly what Craig did and use treetsByAuthor.get("nickrp");
Since we gave Nick his own list of treets, this will return each Treet that Nick has tweeted.
I know that's a lot of information and feel free to shoot back if you're not sure.
For the simple part of your question, when writing code add
the name of the program after your 3 elipses in the opening, treehouse was giving me trouble in editing, so just assume the ... are
your three forward ticks below,
so it would be
...java
...
With of course the forward ticks instead of periods.
Sorry for throwing all the information, feel free to shoot back if you have more questions.
Thanks!

Arthur Podkowiak
3,633 PointsRob,
thank you SO much for talking through this with me. I think I'm finally starting to get it!!! So I now understand the who process, but what was really confusing me this whole time is that get(treet.getAuthor())
method. Correct me if I'm wrong, but within a map, when you say Map.get(key)
it returns the "value" part of the map, correct? Because it has been confusing me so much on what this function actually does as I've been reading it quite literally. I have read the following line completely wrong this whole time, I think:
List<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
This means that treetsByAuthor.get(treet.getAuthor())
searches your current Map for a value by the key, author, right? Let me know if I'm wrong because I definitely understand the entire process of what it's doing but I want to make sure I understand specifically that line of code because it is what has been confusing me the entire time.
Thanks, Arthur
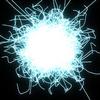
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsNo, you are exactly right Arthur,
that line of code is checking our map for the key, if the key never existed it creates the arrayList that is attached to that authors name, and adds the treet to the List<treet> object for that specific key or value.
It sounds like you pretty much got it!
Good job!
Arthur Podkowiak
3,633 PointsArthur Podkowiak
3,633 PointsHi Rob,
Thanks for the explanation, it's definitely helping! I still do have some questions though if that's alright.
For starters, let me try to explain what Map is and you can correct me, it will help me learn a little better. So
Map<String, List<Treet>>
means we are creating some sort of Map, where the String part is essentially the author's nam, and the List part is the list of Treets by that author. That's what the linetreetsByAuthor.put(treet.getAuthor(), authoredTreets);
does. It just adds those to the Map we've created.List<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
essentially is making an object for theList<Treet>
called "authoredTreets" and it is going through our Map and looking to see if we have a list created for the author that we're getting from our treets list. Here I don't understand how it's checking to see if the authors have a list or not. Because the author is the key, and the Treets that he wrote are the values. I'm having a lot of trouble understanding howList<Treet> authoredTreets = treetsByAuthor.get(treet.getAuthor());
sounds in literal terms, like pseudo code. Would you be able to spell it out for me? That may help. I understand what it's doing, but it's just hard for it to click because the end of the code is using the getAuthor method, which makes me think it's looking for the key and not the value of treet lists.Any thoughts on how you can help? I get the other stuff, like if there is no list created for that author already(since it returns null), then create a new list and add the treet into our map with the associated author. It just seems very backwards to me right now, I can't even place it into words lol.
Thanks!
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey again Arthur,
I know it certainly looks like confusing code it's check to see if the authors already have a treet list or not because we are telling it to, do so before it goes to try to add the list to authoredTreets. If that code was not there the program would never run and fail on every value that it met, because we're telling it that authroedTreets is basically returning the value stored in our map when it is passed in the key of the author
Think of it as sitting down by hand and trying to put these all in a binder that each represents a unique author. You'll first move to add that in a binder, luckily, you have someone watching from the sidelines that sees a name of an author pop up that you don't have a binder for, so he can run over to your desk and give you a binder real quick,
He's checking by the name being passed in and it's flashing on a screen, and he has a list of all the authors that you currently have a treet for, if he finds a new author he needs to run over and give you a new binder, quickly.
Which basically is what (if authoredTweets == null) is doing.
In our code we are going through each Tweet, and is having the get(treet.getAuthor())) passed to it, once this is passed the name from the get method, or the key from the map it can determine if a new binder is needed or not, because there was never a binder used previously as this is the first time you've come across that author.
Another good way to think about it is say you're a freight company and you have multiple orders on the back of your truck, you need to bring those orders off your truck, and put them on a shipping pallet You would get the identifying property of each package by looking at the address (this is the key value, and what out method is doing, it is acquiring the key value and seeing if we have a put to put it), this is the first package you have going to that location, you would have someone who you could singal to, that could get a walkie talkie and have another pallet brought to the floor (This is the method communicating back that there's no place for this to go, or our authoredTreets == null function) With these two operating together, your eyes getting the key value of the address, and the authored treets == null fuction is exactly doing. With the help of getting the key component of that particular box (you used your eyes our code used the getauthor method) and you both were able to signal (either with humans by basic hand gesturing and short wave communication, or a computers more advanced 1's and 0's) you were able to have a place to put your box. Of course our way is a lot more primitive than computers, but that's why they're so popular.
That's a real world example I could think of that would relate to our code.
Thanks I'm not sure if this helped or not, but as always, shoot back if it doesn't. and I'll try to think of a better way to explain it.