Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial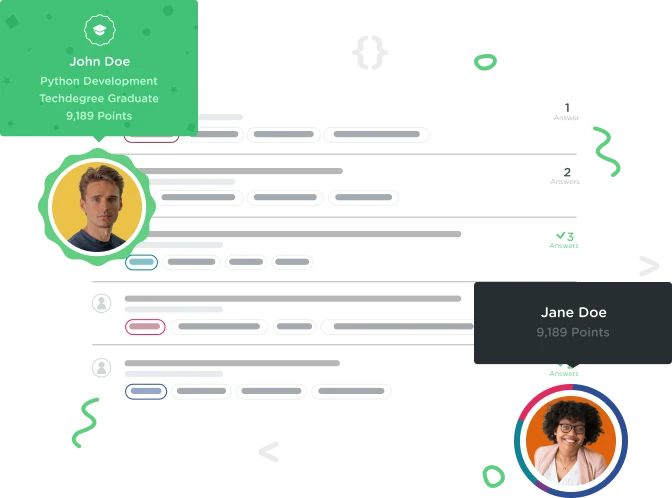

Tsvetelin Buhlev
1,086 PointsTrouble when using "except ValueError as err:" Console displays "invalid literal for int() with base 10: 'blue'."
Near the end of the video, the lecturer has us use an "as err". I would appreciate help on where I went wrong.
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >=1:
name = input("What is your name? ")
print("There are {} tickets remaining, {}".format(tickets_remaining, name))
tickets_wanted = (input("How many tickets would you like to purchase? "))
try:
tickets_wanted = int(tickets_wanted)
if tickets_wanted > tickets_remaining:
raise ValueError("There are only {} tickets remaining.".format(tickets_remaining))
except ValueError as err:
print("Oh no, we ran into an issue. {}. Please try again".format(err))
else:
total_price = tickets_wanted * TICKET_PRICE
print("The total is ${}".format(total_price))
purchase_confirmation = input("Do you want to purchase? Y/N ")
tickets_remaining -= tickets_wanted
if purchase_confirmation.lower() == "y":
print("SOLD! {} tickets left.".format(tickets_remaining))
else:
print("Okay, goodbye {}. ".format(name))
print("Sorry the tickets are all sold out!!")
3 Answers
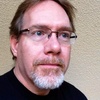
Chris Freeman
Treehouse Moderator 68,460 PointsHey Tsvetelin Buhlev, unfortunately, that text message is created by the Python when "blue"
is presented to the int()
function:
$ python
Python 3.8.5 (default, Jul 28 2020, 12:59:40)
[GCC 9.3.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> int('blue')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: invalid literal for int() with base 10: 'blue'
That is one of the drawbacks to using as err
, you get what the system implementation gives you.
Post back if you have more questions. Good luck!!
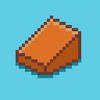
cheeseslope
3,303 PointsThis was bugging me, too. I did some research and discovered that the isnumeric()
method can be used here. The method returns True
if a string contains only numbers. (It’ll return False
if there are letters, a decimal, or any non-numbers in the string.)
I added a second if-else statement, and now, my try-except can print both errors in a friendly way.
Here’s more information → Python String isnumeric() Method
# ==================================================
# Raise ValueError if input is not an integer.
if tickets_requested.isnumeric():
# Input is an integer, OK to convert
tickets_requested = int(tickets_requested)
# Input is not an integer, so raise error.
else:
raise ValueError("Please use number keys to enter ticket quantity.")
# ==================================================
# Raise ValueError if too many tickets are requested.
if tickets_requested > tickets_remaining:
raise ValueError("Sorry {}, we only have {} tickets left.".format(name, tickets_remaining))
# ==================================================
# Print error
except ValueError as err:
print(err)

freddie
2,762 PointsSo my code:
SERVICE_CHARGE = 2
TICKET_PRICE = 10
tickets_remaining = 100
def price_calculation(num_tickets):
return num_tickets * TICKET_PRICE + SERVICE_CHARGE
while tickets_remaining >= 1:
print("Hi, there are {} tickets remaining.".format(tickets_remaining))
name = input("What's your name? ")
num_tickets = input("Hi {}, how many tickets would you like to buy? ".format(name))
#ValueError
try:
num_tickets = int(num_tickets)
if num_tickets > tickets_remaining:
raise ValueError("Sorry {}, we only have {} tickets left.".format(name, tickets_remaining))
except ValueError as err:
print("We are having a problem here. {}".format(err))
else:
amount_due = price_calculation(num_tickets)
order_confirmation = input("Would you like to buy {} tickets for $ {} (y/n)".format(num_tickets, amount_due))
order_confirmation = order_confirmation.lower()
#Gather credit card information
if order_confirmation == 'y':
print("Thank you for your purchase")
tickets_remaining -= num_tickets
else:
print("Thank you {} for your visit".format(name))
else:
print("Sorry, we're out of tickets")
provides this failure message "We are having a problem here. invalid literal for int() with base 10: 'blue' " when confronted with the invalid "number". According to my taste "We are having a problem here." would be sufficient :)
freddie
2,762 Pointsfreddie
2,762 PointsDoes this mean that by implementing the as err scenario, we made the former ValueError structure obsolete?
Chris Freeman
Treehouse Moderator 68,460 PointsChris Freeman
Treehouse Moderator 68,460 PointsFrederic Stein, the same raised
ValueError
instance still exists, except now it has an additional label defined as “err” that can be used to reference it.Aaron Elkind
1,393 PointsAaron Elkind
1,393 PointsChris Freeman Then this answer using the "as err:" breaks the error handling and messaging if a string is input for the number of tickets. So this isn't a complete solution and very confusing because it doesn't make sense to break something to fix another problem. What would be an actual working solution that accounts for both situations?