Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial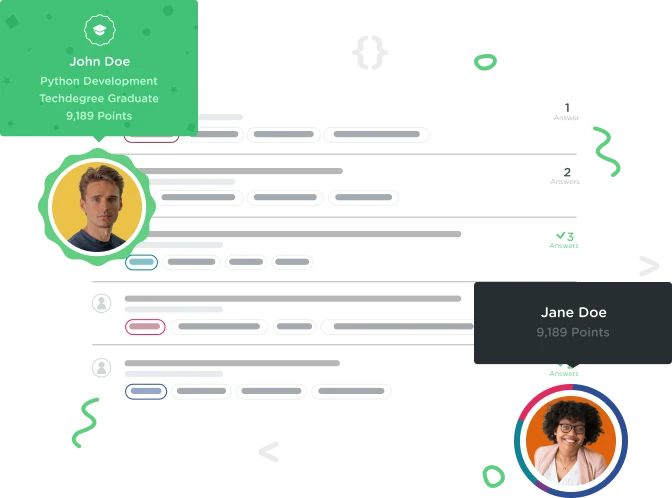
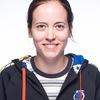
Jo Lingenfelter
Treehouse Project ReviewerTrouble with autolayout anchors. Does anybody have a solution to this coding challenge?
Hi!
I'm having some trouble with this coding challenge. First, when I run the given code, the debugger is telling me that it's breaking a constraint to create the blueView. Can we pin to both sides and then also have a width? I'm confused because that's the given view. Second problem is that I'm getting an error with my code saying that the view does not have a known member "layoutMarginsGuide", but only for the right one; the left one is working fine. I have a solution working in xCode, but the Treehouse compiler is telling me that there is a problem compiling. Any advice would be greatly appreciated.
Many thanks.
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activateConstraints([
NSLayoutConstraint(item: blueView, attribute: .Height, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .Width, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .Left, relatedBy: .Equal, toItem: view, attribute: .LeftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Top, relatedBy: .Equal, toItem: self.topLayoutGuide, attribute: .Bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .Right, relatedBy: .Equal, toItem: view, attribute: .RightMargin, multiplier: 1.0, constant: -8.0)
])
NSLayoutConstraint.activateConstraints([greenView.topAnchor.constraintEqualToAnchor(blueView.bottomAnchor, constant: 8.0),
greenView.rightAnchor.constraintEqualToAnchor(view.layoutMarginsGuide.rightAnchor, constant: -8.0),
greenView.leftAnchor.constraintEqualToAnchor(view.layoutMarginsGuide.leftAnchor, constant: 8.0),
greenView.heightAnchor.constraintEqualToConstant(75)])
// Add constraint code below
}
}
1 Answer
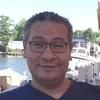
Safwat Shenouda
12,362 PointsHi Jo,
Here are the constraints you need to place for green view:
// Add constraint code below
NSLayoutConstraint.activateConstraints([
NSLayoutConstraint(item: greenView, attribute: .Top, relatedBy: .Equal, toItem: blueView, attribute: .Bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .Left, relatedBy: .Equal, toItem: view, attribute: .LeftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: greenView, attribute: .Right, relatedBy: .Equal, toItem: view, attribute: .RightMargin, multiplier: 1.0, constant: -8.0),
NSLayoutConstraint(item: greenView, attribute: .Height, relatedBy: .Equal, toItem: nil, attribute: .NotAnAttribute, multiplier: 1.0, constant: 75.0)
])
There are few points to take care of: 1) The challenge doesn't ask you to fix the width , you just need to pin right and left sides with 8 points space 2) You don't need to use anchors, you can just do it like the blue view, I dont think that tree-house pre-programmed answers will accept anchors for this challenge(I didnt try) 3)Anyway, if you want to use anchors, you can refer to view anchors directly like this: view.rightAnchor or view.leftAnchor
/// I hope my reply is helpful for you.
Good luck, Safwat
Edit: Code formatting Martin Wildfeuer
Jo Lingenfelter
Treehouse Project ReviewerJo Lingenfelter
Treehouse Project ReviewerThanks so much! It said we could use either, and I'm still confused about whether it's a mistake that the blueView's constraints are conflicted, but thanks for your speedy response. It is greatly appreciated.