Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial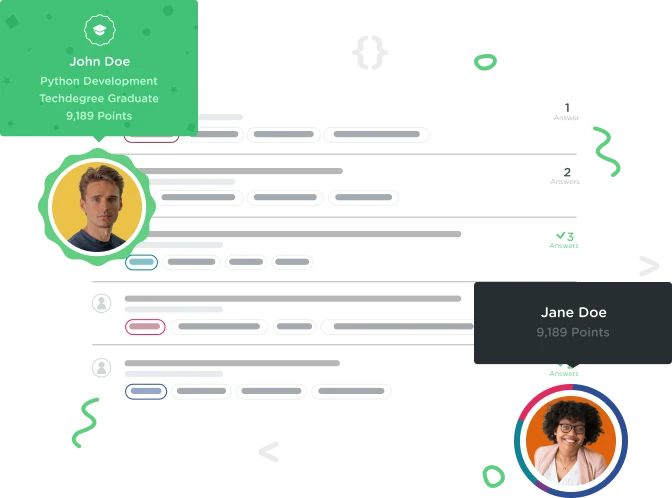

Michael Fitzgerald
7,006 PointsTrouble with foreach loops
I'm trying to complete this challenge and I'm coming up short. I took a look at what a few other people did in their questions and I thought I was on the right track, but I'm getting an error that says: "An object reference is required to access non-static member `Treehouse.CodeChallenges.Frog.TongueLength' "
Could someone point out what I've done wrong or give me a hint so I can figure this out? Thanks
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double sum;
int count;
foreach(Frog frog in frogs) {
sum += Frog.TongueLength;
count ++;
}
return sum / count;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
4 Answers

Oisin M
5,463 PointsYou are calculating the average before the foreach loop (At this point sum is 0 so average will also equal 0). This is the value you are returning at the end of the function.
Instead you want to put "double average = sum / count;" after the loop

Oisin M
5,463 Pointssum += Frog.TongueLength;
Frog (with a capital 'F') refers to the class, whereas frog (small 'f') refers to the current instance of the class in the foreach loop.
You can easily see errors like this if you copy the code into Visual Studio.

Michael Fitzgerald
7,006 PointsThat helped with that particular problem, thank you. But while my code compiles I'm getting an error from Treehouse saying my code isn't returning the average tongue length.
public static double GetAverageTongueLength(Frog[] frogs)
{
int count = frogs.Length;
double sum = 0;
double average = sum / count;
foreach (Frog frog in frogs){
sum += frog.TongueLength;
}
return average;
}
I tried it like how it was originally, so I changed the count variable to use the length of the array and it still isn't doing much.

Karen Ho
10,190 PointsThis works - public static double GetAverageTongueLength(Frog[] frogs) { double total = 0.0; int count = frogs.Length; for (int i=0; i < frogs.Length; i++) { total = total + frogs[i].TongueLength; } return total / (frogs.Length); }