Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial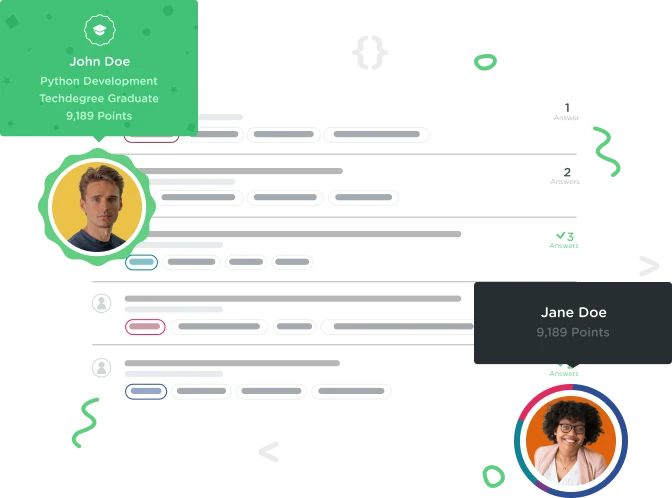
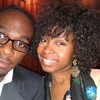
Jeremy Martin
Courses Plus Student 2,521 PointsTrouble with Intents using String[]
Code Challenge: The member variable 'mUrls' is filled with a few URLs. In the 'onListItemClick()' method, declare and start an Intent that causes the URL tapped on to be viewed in the browser. Hint: The String URL needs to be set as the data for the Intent as a Uri object.
The trouble is with the Strings and Intents at the bottom.
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.view.View;
import android.view.ListView;
import android.content.Intent;
import android.net.Uri;
public class CustomListActivity extends ListActivity {
protected String[] mUrls = { "http://www.teamtreehouse.com", "http://developer.android.com", "http://www.github.com" };
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_custom_list);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, mUrls);
setListAdapter(adapter);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
String URLs = mUrls.getString(position);
String URL = URLs.getString();
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(URL));
startActivity(intent);
}
}
10 Answers
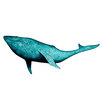
Deoward Kotze
14,604 PointsHey Jeremy, I had a look and the following seems to pass the challenge:
String URL = mUrls[position];
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(URL));
startActivity(intent);
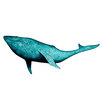
Deoward Kotze
14,604 PointsI don't see any problems with the way you are creating the Intent and setting the Uri, so I would think the problem lies in the way you are retrieving the String you want to pass as the uri from mUrls array.
String URL = URLs.getString();
Why are you calling getString() again without parameters?
You could just do the following:
String URL = mUrls.getString(position);
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(URL));
startActivity(intent);
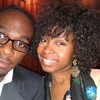
Jeremy Martin
Courses Plus Student 2,521 PointsThanks for the feedback! I put the extra getString() in there because I was desperate... lol. Tried a number of String combinations including what you have here. Been a this one for hours and I am really not sure what else to try.
Any suggestions?
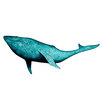
Deoward Kotze
14,604 PointsCould you give me some specifics about the errors or exceptions you are getting?
perhaps try the following:
String URL = (String) getListAdapter().getItem(position);
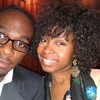
Jeremy Martin
Courses Plus Student 2,521 PointsThe error message from the original code posted:
Bummer! Compilation Error! Check your syntax and make sure you are correctly declaring your intent, setting its data, and then starting it using the 'startActivity' method.
I receive the same error message for both suggested solutions as well.
Forgive my ambiguity, I'm a newbie.
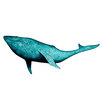
Deoward Kotze
14,604 PointsAgh apologies buddy, I didn't realise it was a code challenge...:)
perhaps they want you to create the intent and pass the uri as data in the same line.
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(URL));
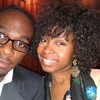
Jeremy Martin
Courses Plus Student 2,521 PointsOkay, I tried that as well and I received the same error message as before. I am so lost with this one. I feel like I'm being tricked. Lol
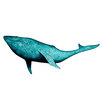
Deoward Kotze
14,604 Pointsyeah I know the feeling - but without proper debugging tools it can be very to hard to find out what you are doing wrong! What course is this code challenge from & what video is the challenge based on? Perhaps I can have a look at it and see if I can figure out exactly what they want you to do here....
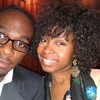
Jeremy Martin
Courses Plus Student 2,521 PointsI think Ben said they would be working on a debugging functionality for the Code Challenges this week...
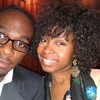
Jeremy Martin
Courses Plus Student 2,521 PointsTrack: Android Development
Project: Build a Blog Reader Android App
Stage 5: Using Intents to Display and Share Posts
Video: Opening a Webpage in the Browser
.... It's the first video and Code Challenge of the bunch... which is crazy because I was able to finish the other ones in this section.
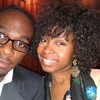
Jeremy Martin
Courses Plus Student 2,521 PointsWow... Thank you!!!
So, 'String URL = mUrls[position];' does not accomplish the same result as using 'String URL = mUrls.getString(position);'???

Lyubomyr Ostapiv
1,119 PointsHey. thanks for answer guys. I had no luck with intent.setData(Uri.parse(mUrls[position])); but two-line code worked String URL = mUrls.[position]; intent.setData(Uri.parse(URL));