Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial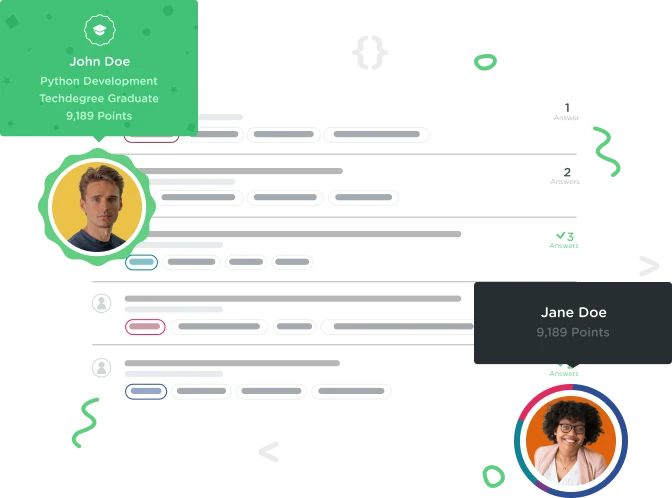

H Yang
2,066 PointsTrouble with mathematical shorthands
Hello,
I'm having trouble with math shorthands. My approach for generating a random number between two inputted number was to find the difference between the two numbers, multiply the difference by Math.random, and then take the Math.floor of the resulting number.
My trouble comes up when I try using mathematical shorthands. My code works when I don't use the -= shorthand and type out:
var output = input2 - input;
but doesn't work when I try using "-="
Can someone help me out? Here's my code.
alert("Hey! It's dangerous to go alone!");
var input = prompt("Let me see what I can do to help.2.0. Please give me a positive number.");
alert("Great!");
var input = parseInt(input);
var input2 = prompt ("One more number and I'll be able to forge something for you. Make this number greater than the first number.");
var input2 = parseInt(input2);
input2 -= input;
var weapon = Math.floor(Math.random() * input2) + 1;
var input += weapon;
alert (input);
2 Answers
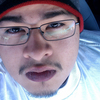
Chyno Deluxe
16,936 PointsI believe that the problem is that you are overwriting your variables input1 and input2 and that is causing your code not to work.
Try this. jsFiddle Demo
alert("Hey! It's dangerous to go alone!");
var input = prompt("Let me see what I can do to help.2.0. Please give me a positive number.");
alert("Great!");
input = parseInt(input); //removed var declaration
var input2 = prompt ("One more number and I'll be able to forge something for you. Make this number greater than the first number.");
input2 = parseInt(input2); //removed var declaration
input2 -= input;
var weapon = Math.floor(Math.random() * input2) + 1;
input += weapon; //removed var declaration
alert (input);
i hope this helps.

Jacques Retief
954 PointsHenry. the var command declares variables. the = sign changes or redefines the value inside the variable.
Chryno Deluxe, could Henry then use this instead to return a number?
var input = parseInt(prompt());
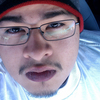
Chyno Deluxe
16,936 PointsYes, the code would turn any number response into an integer but you'll want to try and add a conditional statement to alert the user when they've provided an answer that does not include a number. Otherwise, your parseInt() will return a value of *NaN.
H Yang
2,066 PointsH Yang
2,066 PointsHey, that makes a lot of sense. Thank you for pointing out that var declaration is a problem.
When I'm doing var declaration here, what is actually happening? I understand that it redefines the variables. What is going in that space when I write
var input = parseInt(input)
? A null string?
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 PointsThe code above would return as NaN, meaning Not a Number.
It returns not a number because your input variable's value is undefined which is not a typeof string that can be parsed into an integer.