Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial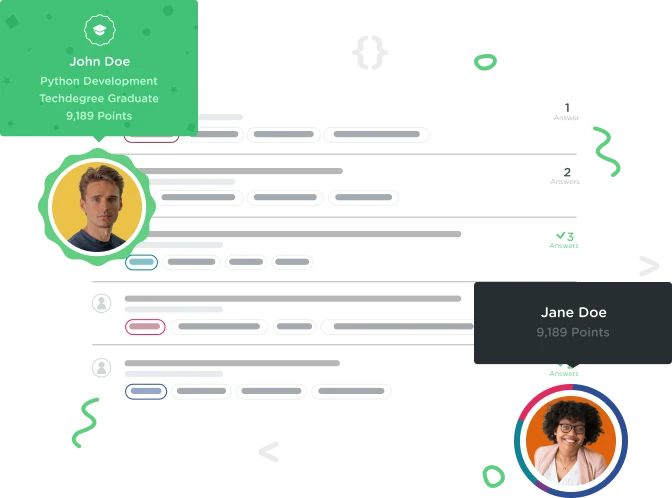
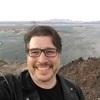
Craig Ossandon
974 PointsTrouble with raising exception challenge, unable to generate ValueError
I'm having some trouble with raising an exception when a user enters in a word that is less than three characters. I pretty sure I went a bit more than what the challenge asked for. I ran it in a file I created in workspaces, the script runs, but runs and does not kick back the exception when I type something with two characters. Checked the error messages when I ran the test, but having a hard time locating the trouble, seems it's probably something with the if statement. Here's what I did...
def suggest(product_idea):
if product_idea < 3:
raise ValueError("Please choose a longer name")
return product_idea + "inator"
try:
product_idea = input("Please suggest an idea! ")
except ValueError as err:
print("({})".format(err))
else:
print(product_idea + "inator", "\n" "Thank you for your ideas")```
treehouse:~/workspace$ python suggestinator.py
Please suggest an idea! B
Binator
Thank you for your ideas
3 Answers

Robin Goyal
4,582 PointsOhh. I see the challenge now and I misunderstood the situation. The code you had for the function in the beginning was correct for the challenge itself. I gave you advice on how to raise the error and catch the exception for it.
In your new code, the tests are not recognizing a ValueError exception being raised since you're actually catching the exception. By catching the exception, you suppress the ValueError which was raised and handle it yourself. In the case for this challenge, you just want the exception to occur which is what you had correct in your function in your initial question.
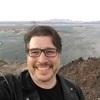
Craig Ossandon
974 PointsOhhhh ok, I was a bit confused and I wrote too much and I figured it was not exactly what the challenge was asking. Well, it works, just not showing the exception the way it's suppose to occur, like you said. Again, thanks for the help, much appreciated.

Robin Goyal
4,582 PointsNo problem Craig! It looks much better and easier to read. I'm still having a little trouble fully understanding your code. The suggest function is not being called within the try-except-else block so the ValueError exception won't be raised. You should check that the length of the product_idea is less than 3 also.
Here's one take on it. I had to make a few changes for your code.
def suggest():
try:
product_idea = input("Please suggest an idea! ")
if len(product_idea) < 3:
raise ValueError("Please choose a longer name")
except ValueError as err:
return ("({})".format(err))
else:
return (product_idea + "inator" + "\n" + "Thank you for your ideas")
The try block first prompts the user for input regarding the product idea and then it checks that the length of the idea is less than 3. If there are 3 or less characters, it'll raise the ValueError exception with your custom message. The Except clause will match the raised ValueError Exception and execute the block of code inside. Otherwise, if no exception is thrown, the thank you message will be returned.
The key here is that you want to raise your exception in the try block if you want to catch it!
I hope that helps! Please let me know if you have any more questions.
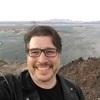
Craig Ossandon
974 PointsHey Robin, I see what you mean. So I changed the order under the try statement, made some changes and it works when I run it on my workspace, but when I reset the challenge, start clean and redo the code, i'm still getting the following error:
def suggest(product_idea):
return product_idea + "inator"
try:
product_idea = input("Please suggest an idea! ")
if len(product_idea) < 3:
raise ValueError("Please choose a longer name")
except ValueError as err:
print("({})".format(err))
else:
print(product_idea + "inator", "\n" "Thank you for your ideas") ```
This is the challenge error
----------------------------------------------------------------------
Ran 1 test in 0.000s
OK
.F
======================================================================
FAIL: test_exception_raised (__main__.TestRaiseExecution)
----------------------------------------------------------------------
Traceback (most recent call last):
File "", line 32, in test_exception_raised
AssertionError: ValueError not raised : I passed in 'a' and expected a ValueError to be raised
----------------------------------------------------------------------
Ran 2 tests in 0.000s
FAILED (failures=1)
This is what I get when I run it on my workspace, I get the exception
treehouse:~/workspace$ python suggestinator.py
Please suggest an idea! Fly
Flyinator
Thank you for your ideas
treehouse:~/workspace$ python suggestinator.py
Please suggest an idea! Pi
(Please choose a longer name)
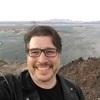
Craig Ossandon
974 PointsThank you Robin, I was trying to get the formatting correct, I was missing the word python, that looks much better, thanks for the help :)
Robin Goyal
4,582 PointsRobin Goyal
4,582 PointsQuick comment! You may want to format your python code according to the Markdown guidelines. It's easier for people to see what your issue is. Use the three backticks(`) followed by python, your code and then three closing backticks (`).