Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial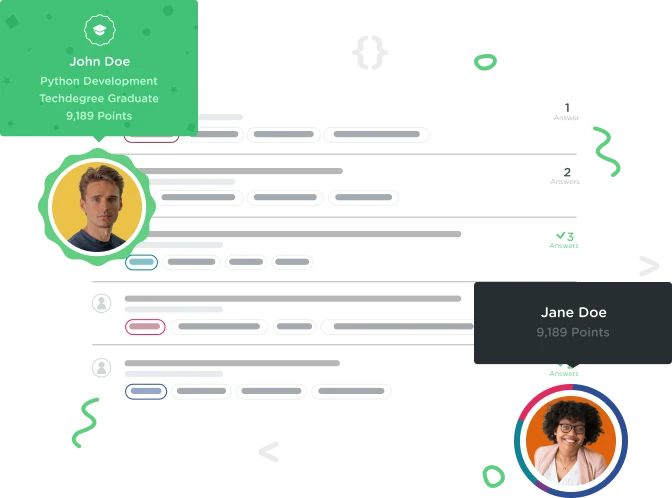

Matthew Earlywine
3,508 PointsTrouble with Task 2
Great now that you have created your new square method, let's put it to use.
Under the function definition, call your new function and pass it the argument 3.
Since your square function returns a value, create a new variable named result to store the value from the function call.
def square(argument):
try:
return int(argument) ** 2
except ValueError:
return str(argument) * len(argument)
7 Answers

Craig Dennis
Treehouse TeacherI am asking you to use the function you just created.
The function is defined correctly, and I want you to call it with the value of 3. Since it returns a value, store it in a variable named result.
That make sense?

Keith McDonald
816 PointsCraig, Ive written out the task and performed it correctly but when I go to run the square function it just spits out a syntax error near unexpected token. What am I doing wrong with this?

Craig Dennis
Treehouse TeacherCan you share your code?

Keith McDonald
816 Pointsdef square(number):
return((number)**2)
result = square(3)

Craig Dennis
Treehouse TeacherHmm...wonder if it's those parentheses around that return statement. Try removing those. Return is a statement, not a function so you don't need to call it. Also the parents around number are not needed.
If you use the preview pane it'll tell you were the error is.
I'm away from a computer right now, but let me know if you are still stuck.

Keith McDonald
816 Pointsyeah so ive changed the code to
def square(number):
return number**2
Result = square(3)
I ran it in treehouse:~/workspace$ python number.py and it returns back to treehouse:~/workspace$
I put it into the REPL as well and it just says invalid syntax line 1 python number.py ^ Im not sure what Im missing. Im guessing its something simple.

Craig Dennis
Treehouse TeacherIf you lowercase that Result
to result
it passes for me.
Is it not working for you on the challenge?

Keith McDonald
816 PointsThank you sir that seems to have done the trick.
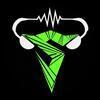
Suryansh Sisodiya
Python Development Techdegree Student 382 PointsThank you sir, you're helping me a lot with this :D

Mohammed Anam
829 PointsHi Craig,
I am struggling a bit in deciphering the directions for this specific exercise. The exercise says, "Call your new function and pass it the argument 3. What does it mean when it says "pass it the argument 3?" I have no idea what that means. I saw people put (3) but I don't know why. I know that you need to commas to separate arguments, but I'm really confused on the verbiage. Apologies if my questions are vague.
Respectfully,
Mohammed Anam

Antonio De Rose
20,885 Pointsit is asking to call the function, that you created and pass the argument 3, and then store the value in a variable called result.
3 thins you will have to know.
1) how do you call the function you created ? square
2)how to pass an argument into the call function ? square(3)
3) how to store the value from the result you get ? result = square(3)

Matthew Earlywine
3,508 PointsThis is what I came up with but it's not passing. def square(num): try: int(num) return(num * num) except ValueError: return(num * len(num))
square(num = num3) num3 = result

penpen
618 PointsI was able to figure out task 1 but struggled with task 2. I don't comprehend why pass it to argument 3 equates square(3).

Antonio De Rose
20,885 Points@Pierre ILYAMUKURU
I ran your code, and it did say, success, I am sure, that you have an issue to do with the indentation. next time, when you post your code, please the code formatting option, under markdown sheet.
def square(number):
value=(number*number)
return(value)
result=square(3)
print(result)
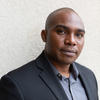
pierreilyamukuru
9,831 PointsThank you so much, Antonio is working perfectly right now.
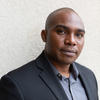
pierreilyamukuru
9,831 PointsHello, I did my code and pass the first task and the second task failed. Can you please help me to figure out where I am doing the mistake. Here are my code
def square(number):
value=(number*number)
return(value)
result=square(3)
print(result)

Howard Kutiwa
5,648 Pointsdef square(number): return number*number
result = square(3) print(result)

Matthew Green
446 PointsHello everyone, this is my first post in the community and really struggled with this challenge but i eventually passed it, how ever looking back at the results others have been getting my code seems a little basic, but i still managed to pass it.
def square(number):
return number * number
square(3)
result = square(3)
print(result)
Am i on the right track or was i way off here?

jon nikolakakis
1,639 Points@antonioderose
def square(number):
return number * number
### square(3) This part isn't needed.
result = square(3)
print(result)
Should get 9

r j weiner
2,741 PointsI think you don't need the
print(result)
Matthew Earlywine
3,508 PointsMatthew Earlywine
3,508 PointsI am not sure what to do for this one.