Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial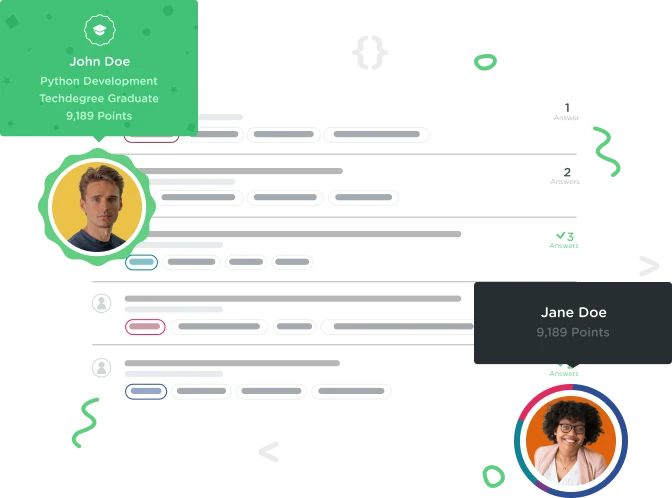

LeJacque Gillespie
3,990 PointsTrouble with the sign up
I have typed everything like in the video, but I keep throwing an error when the app tries to run. Now, instead of using activity login I was forced to used fragment login (guess it's just a newer version). This is my code from Login Activity:
package com.lejacque.ribbit2;
import android.app.Activity; import android.app.Fragment; import android.content.Intent; import android.os.Bundle; import android.view.LayoutInflater; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.ViewGroup; import android.widget.TextView;
public class LoginActivity extends Activity {
protected TextView mSignUpTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mSignUpTextView = (TextView)findViewById(R.id.signUpText);
mSignUpTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Intent intent = new Intent(LoginActivity.this,
SignUpActivity.class);
startActivity(intent);
}
});
if (savedInstanceState == null) {
getFragmentManager().beginTransaction()
.add(R.id.container, new PlaceholderFragment()).commit();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.login, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_login,
container, false);
return rootView;
}
}
}
AND THIS IS MY CODE FROM FRAGMENT SIGHUP.XML
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.lejacque.ribbit2.SignUpActivity$PlaceholderFragment" >
<EditText
android:id="@+id/usernameField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:ems="10"
android:hint="@string/username_hint" >
<requestFocus />
</EditText>
<EditText
android:id="@+id/passwordField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/usernameField"
android:layout_below="@+id/usernameField"
android:ems="10"
android:inputType="textPassword"
android:hint="@string/password_hint"/>
<EditText
android:id="@+id/emailField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/passwordField"
android:layout_below="@+id/passwordField"
android:ems="10"
android:inputType="textEmailAddress"
android:hint="@string/email_hint"/>
<Button
android:id="@+id/signupButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/emailField"
android:layout_below="@+id/emailField"
android:text="@string/sign_up_button_label" />
</RelativeLayout>
THIS IS WHERE THE ERROR SHOWS:
mSignUpTextView = (TextView)findViewById(R.id.signUpText); mSignUpTextView.setOnClickListener(new View.OnClickListener()
Any help will be appreciated to get this code to run.
1 Answer

James Simshaw
28,738 PointsHello,
The answer to your question really comes down to if you really want to be using fragments or if you want to have something simpler(and still correct) that is very close to what the videos show. Since it would be a lot easier to following along with the simpler version, I'll show you how to remove the fragment in this case. You want to remove the whole PlaceholderFragment inner class as well as the lines
if (savedInstanceState == null) {
getFragmentManager().beginTransaction()
.add(R.id.container, new PlaceholderFragment()).commit();
}
in your Activity's onCreate method. Which should make your java code look close to
package com.lejacque.ribbit2;
import android.app.Activity;
import android.app.Fragment;
import android.content.Intent;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
public class LoginActivity extends Activity {
protected TextView mSignUpTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
mSignUpTextView = (TextView)findViewById(R.id.signUpText);
mSignUpTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Intent intent = new Intent(LoginActivity.this,
SignUpActivity.class);
startActivity(intent);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.login, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
Then you want to copy
<EditText
android:id="@+id/usernameField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:ems="10"
android:hint="@string/username_hint" >
<requestFocus />
</EditText>
<EditText
android:id="@+id/passwordField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/usernameField"
android:layout_below="@+id/usernameField"
android:ems="10"
android:inputType="textPassword"
android:hint="@string/password_hint"/>
<EditText
android:id="@+id/emailField"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/passwordField"
android:layout_below="@+id/passwordField"
android:ems="10"
android:inputType="textEmailAddress"
android:hint="@string/email_hint"/>
<Button
android:id="@+id/signupButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/emailField"
android:layout_below="@+id/emailField"
android:text="@string/sign_up_button_label" />
into your activity_login.xml file. This should get your code in line more with what the videos show and get rid of your NullPointerException(at least I'm guessing your error was a NullPointerException from the segment of code you showed the error happening at). Let me know if you have any further questions and if this fixes your issue.
LeJacque Gillespie
3,990 PointsLeJacque Gillespie
3,990 PointsThanks, but it didn't help. I even deleted the entire file and started over again and received the same errors. I downloaded eclipse because I thought it wold be easier to follow along but maybe I need to try it on android studio.
James Simshaw
28,738 PointsJames Simshaw
28,738 PointsCould you give the full error message that you are getting? That would help with figuring out what is actually going on. Also, an updated version of your code would likely help at this point as well.