Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial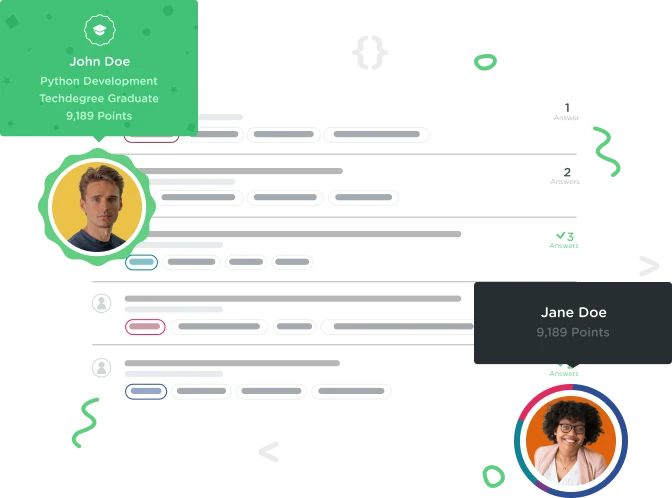

Katie Ferencik
1,775 PointsTruthiness of while tickets_remaining: never turns false - getting negative numbers
For fun, I wanted to play with the "truthiness" of tickets_remaining, as it was mentioned in the beginning, so I made my while statement as:
while tickets_remaining:
instead of
while tickets_remaining >= 1:
but when I ran it, I kept receiving negative numbers as the number of tickets left. For example, I would "buy" 90 tickets and then 100 tickets and I'd get "There are -90 tickets left".
Here's my complete code (ignore the different types of formatting - I was playing around with that as well):
while tickets_remaining:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("Please enter your name: ")
num_tickets = int(input("Hi {}, how many tickets would you like to buy? ".format(name)))
amount_due = num_tickets * TICKET_PRICE
print("The total for these tickets is ${}.".format(amount_due))
answer = input("Would you like to purchase the {} tickets for ${}, {}? (Y/N) ".format(num_tickets, amount_due, name))
if answer.lower() == "y":
print("SOLD!")
tickets_remaining -= num_tickets
else:
print(f"Thank you, {name}!")
print(f"Sorry, {name}, all the tickets are sold out!")
ULTIMATE QUESTION --->But when the truthiness of tickets_remaining hit 0, it didn't turn false.Why is that?
4 Answers

Jassim Alhatem
20,883 PointsHi, Katie Ferencik,
Before I tell you why your program is doing that. You need to know something. Every number is Truthy except for 0. Try this in your console:
> num = 5
> bool(num) # It will return True
> zero = 0
> bool(zero) # It will return False
So, while tickets_remaining >= 1:
is checking if the remaining tickets are greater or equal to 1. Therefore, it's going to keep looping until the condition is false. But, while tickets_remaining:
is basically saying: while True:
if the value isn't zero of course. That's why you're getting those results. Here's a simple example
ticket = 5
while ticket:
buy = input("Press ENTER to buy")
ticket -= 1
When the value of ticket
reaches 0 it'll end the loop, because it is no longer True. I hope that makes sense. If you have anymore questions, please ask :). Have a great day.

Jassim Alhatem
20,883 PointsSo you have 100 tickets, you buy 90, there are 10 left. Then you buy 100 again and it says that there are -90 tickets remaining. I'm guessing that's what's confusing you "Why is it -90".
Before I get to it when you buy 100 tickets when there are 10 remaining which is (10-100) the result is going to be -90. It hits zero when you subtract from it 10 (10-10). That's how mathematics works. That's what the while tickets_remaining >= 1
is for. It checks if the remaining tickets are more than or equal to 1, if it is true it's going to keep running the loop.
So your program is subtracting the number of tickets that the user wants num_tickets
from the number of tickets remaining tickets_remaining
. tickets_remaining -= num_tickets
.
Unless you enter '100' in the input, it will always be True. I hope I answered your question this time.

Katie Ferencik
1,775 PointsErrr - no not really. Okay, I understand how math works. I understand that 10 - 10 = 0.
I also understand that while tickets_remaining >=1
will make sure to break the loop once the tickets_remaining is 0, to prevent people from purchasing more than is available - this works and I understand that!
However, in the teaching video, it is mentioned that while tickets_remaining:
will also work, because once tickets_remaining is 0, then it will be false and exit the while loop. So, I expect both of these to work the same, and am playing around with the latter, not the former.
Maybe I'm not explaining myself clearly, and I apologize for that. Maybe I'm not understanding what you're saying but there's definitely a disconnect, so let me try again:
Let's say that tickets_remaining = 100.
Then, let's say the user enters 190. There's not enough tickets, because, as you said, math works like this: 100 - 190 = -90, and you can't (or shouldn't) sell negative 90 tickets.
But wait a minute! With that request, shouldn't while tickets_remaining:
hit 0 and break the while loop because it's no longer true? Didn't it hit zero at some point during the math equation and become false?
Why would the transaction be allowed to progress into negative numbers without becoming false once it hits and passes zero? Are you saying that the only way to make it break false is for tickets_remaining = 0 (and not any other negative number)?

Jassim Alhatem
20,883 PointsNo, it never hit zero during the match equation. I think he meant when you actually buy the amount that is remaining, not over that amount.
You can manually end the loop by using the break
statement.
if ticket_remaining < 0:
break
Also, you can check if num_tickets
is bigger than tickets_remaining
. If it is, return a message and use the continue
statement to start the loop back from the top.
if num_tickets > ticket_remaining:
print("write a message")
continue
I hope you got an answer this time xD. Sorry about the confusion.

Jassim Alhatem
20,883 PointsI just want to add:
Even if it hits zero at some point during the equation. It's not going to break the loop. Because after the equation, the condition runs tickets_remaining
. So basically, it checks if tickets_remaining is True after the equation.

Katie Ferencik
1,775 PointsIt's all good! Communicating through text isn't always ideal - thanks for sticking with it though. I appreciate it!

Jassim Alhatem
20,883 PointsNo problem :) have a great day.
Katie Ferencik
1,775 PointsKatie Ferencik
1,775 PointsJassim - thanks for the response, but it doesn't really answer my question, since the problem is the loop never ends when the value of ticket hits zero. Instead, it gives a negative number back. I understand conceptually that it should hit zero and then turn false, but it never does.
So, tickets_remaining has 100 tickets. So then I "buy" 90 tickets. Now, it has 10 tickets_remaining. So then I buy 100 tickets. Ideally it should hit zero and then be false and stop running the loop, but instead, I get: -90 tickets_remaining.
So then, what's happening when it hits zero? Why doesn't it turn false?
Thanks!