Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial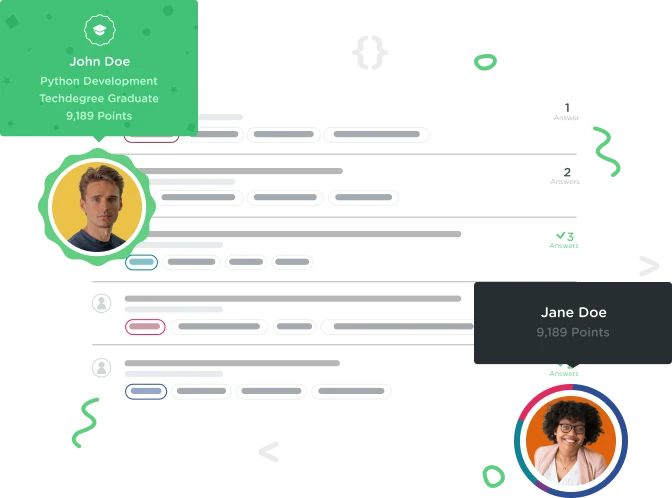

Abdulkadir Kollere
1,723 PointsTry again
I came up with the attached code. I am stuck and I dont know how to go about this challenge. Please help
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(word):
splitted_word = word.split( )
for word in splitted_word:
dic = dic.update({word : splitted_word.count(word)})
return dic
1 Answer
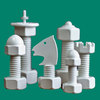
Steven Parker
231,269 PointsIt looks like you're close, but two things stand out for me:
- you should initialize dic as an empty dictionary before you reference it
- .update modifies the dictionary directly, it does not return anything (don't assign it to dic)
Abdulkadir Kollere
1,723 PointsAbdulkadir Kollere
1,723 PointsI tried initialising with dic = {:} and it returned an error also. will the kenneth[key] = "value" format work for the update?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsAnother approach might be directly assigning the dictionary using the key as an index. But remember that you want to accumulate a count, so you'd want to assign it to
1
only if it had not been added yet, and otherwise increase the value already stored.Abdulkadir Kollere
1,723 PointsAbdulkadir Kollere
1,723 PointsSteven can you please advise on how to initialize empty dictionaries?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou had it, but leave out the colon:
dic = {}
Abdulkadir Kollere
1,723 PointsAbdulkadir Kollere
1,723 PointsHi Steven. I am . struggling with this part of the challenges. I initialised the dic variable to be an empty dictionary. Now when I try the code in workspaces, it does not return anything and there is no error. Please look at the code below.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou nearly had it.
But you can't apply lower() to a list. You can, however, apply it to the string right before you split it:
splitted_word = word.lower().split()