Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial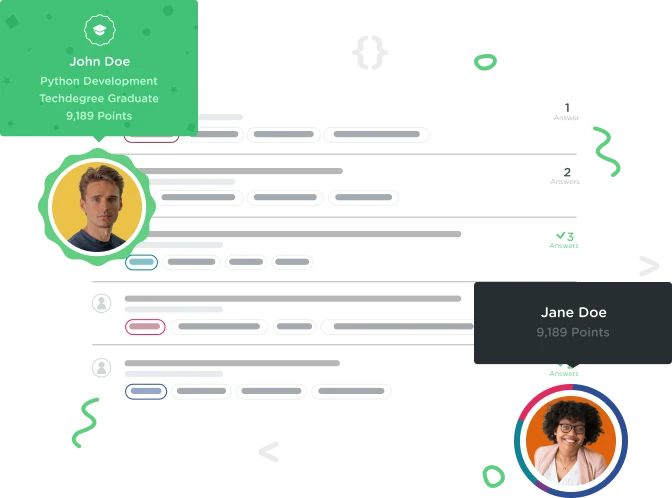

henry jefferson
2,136 PointsTry and except problem. I'm trying to write a function that outputs only a letter; an alphabetic letter.
Try and except problem. I'm trying to write a function that outputs only a letter; an alphabetic letter. It should also not complete until the user has inputted a letter (hence while True..) Please look at the code and let me know where things are going wrong.
def guess(): while True: try: guess = ( 'choose a sinlge letter: ') guess.isaplha() except TypeError: print ('must be a single letter') except False: print ('must be a single letter') else: break
print (guess) return guess
guess()
10 Answers
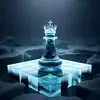
crosscheckking
25,155 PointsIs this about what you wanted it to do? As Gavin Ralston said, there is a typo for isalpha() and you will need to include the input function to allow users to input data.
def guess():
while True:
user_guess = input('Choose a single letter: ')
if user_guess.isalpha():
print(user_guess)
break
else:
print('You must enter a single letter')
return user_guess # I'm assuming this is for later when you want to use their guess
guess()

Gavin Ralston
28,770 PointsTwo things that stand out:
guess.isaplha() # is misspelled: you want .isalpha()
and the more obvious issue:
guess = ( 'choose a sinlge letter: ')
Won't work the way I think you want it to. You want to capture the input from a user, so use this:
guess = input( 'choose a sinlge letter: ')
There's more to work on, but that should help you with the syntax so you can focus on the logic and try/except blocks.

henry jefferson
2,136 PointsThankyou

Kevin Li
2,634 PointsYou said something about inputting a letter... did you want to use input()?

henry jefferson
2,136 Points'''def guess(): while True: try: guess = ( 'choose a sinlge letter: ') guess.isaplha() except TypeError: print ('must be a single letter') except False: print ('must be a single letter') else: break
print (guess) return guess
guess() '''

henry jefferson
2,136 Pointssorry guys! how do I copy in the code so that the formatting isn't altered?? Thanks

Gavin Ralston
28,770 PointsClick on the markdown cheatsheet link below the editor window for details. :)
You want to use three backticks and the word "python" on the first line, then three backticks on the last line to format your code.

henry jefferson
2,136 Pointsthanks Gavin.
''' python def guess(): while True: try: guess = ( 'choose a sinlge letter: ') guess.isaplha() except TypeError: print ('must be a single letter') except False: print ('must be a single letter') else: break
print (guess) return guess
guess() '''

henry jefferson
2,136 Pointsdef guess():
while True:
try:
guess = ( 'choose a sinlge letter: ')
guess.isaplha()
except TypeError:
print ('must be a single letter')
except False:
print ('must be a single letter')
else:
break
print (guess)
return guess
guess() ```

henry jefferson
2,136 Pointsok wooo, managed to post the code. Let me know what is going wrong here if you can. I'm trying to create a function that will only return a letter and not break until a letter is inputted.
Thanks

henry jefferson
2,136 PointsThanks, guys. I think I was trying to work in a 'try/ except' block where it's not necessarily required.

Gavin Ralston
28,770 PointsJust keep in mind that any exception handling (try/except in python, try/catch in many other languages) is something you should typically use when there are factors outside of your control (file i/o, network connections, etc)
In the case of collecting user input from the console, which is always going to be a string, and all you really have to do is determine if it's a single character and whether or not it's a letter, you've got no real reason to worry that you'd get an exception anyway.
In fact, in a case like that, you'd probably almost want it to throw an unhandled exception and draw attention to it right away, as it probably indicates a bigger problem you'd want to fix in your code.
I think later on in the course you'll run into a try/except situation where you're converting strings to integers or some other data type. There, you'll want handle an exception because it's likely a user could enter the wrong information, or information that (like a string that should represent a number, but might contain a typo or letter, that wouldn't get cast to an int) In cases like that, you'd want to use try/except because it's very possible to get that ValueError or TypeError or whatever, and you'll want to handle that smoothly.

henry jefferson
2,136 PointsAgain, thank you Gavin and John for taking the time to look at this, much appreciated.
henry jefferson
2,136 Pointshenry jefferson
2,136 PointsAppreciated, thanks John. That's what I'm after. Would you happen to know how I could write this using 'Try and except?' Or would that really not make sense here? I know if I was checking for an integer as an output from the input() function I could include an 'except ValueError' to catch none int.'s. In other words, can I use try /except to catch none alphabetic values coming from input()?
Thanks
crosscheckking
25,155 Pointscrosscheckking
25,155 PointsYou're welcome. I'm not sure how to add try/except in this case to be used to test if the input is a single letter. Like you said you would need it if you were converting the input to a number, but input returns a string and we are just testing to see if they gave us a single letter (it says a letter in the original question so I'm assuming it is one letter you are wanting from the user). isalpha() returns True or False depending on if the contents of the variable are letters or not, so this will not crash the program and activate the except block if someone inputs a number (just False will be returned instead). I tried a few things and couldn't think of a way to make it crash without the try/except blocks. If you want the extra exception handling though you could do the following. Instead of crashing (if someone found a way), it would gather the description of the Exception and print it to the terminal/console and then rerun the function. I also added an additional test for the input to make sure that it is one character, but you can remove this if that's not the case. There probably is a way to implement the try/except the closer to the way you want, but I'm not sure (and this should do just fine).
Sachin Kanchan
564 PointsSachin Kanchan
564 PointsHi John, Good day to you. In your 2nd code with TRY / EXCEPT, why did you call the guess() function twice? Regards