Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial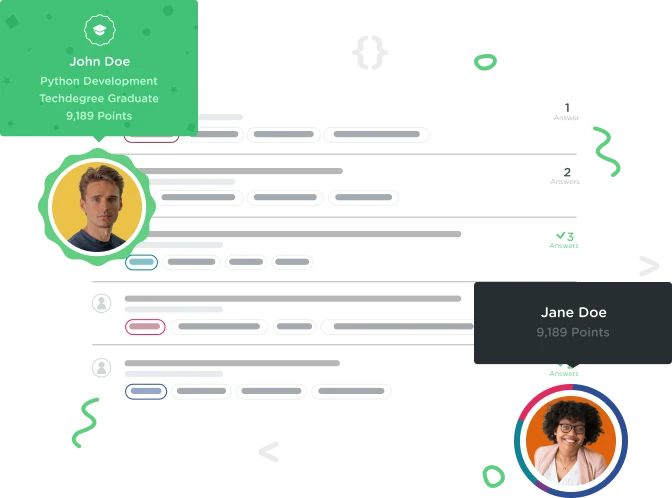

Pat Dudar
2,490 PointsTry and Exception coding problem
I'm trying to do a try, except, and else check on the parameters x and y in the following script, and am not sure how to move forward. This is from the very last question in Python Basics. Anyone have any tips? I don't know if I need to provide an input statement to test this out:
def add(x, y):
try:
x = int(input("Enter a number: "))
y = int(input("Enter a number: "))
except ValueError:
return None
else:
a = float(x)
b = float(y)
return (a + b)
def add(x, y):
try:
x = int(input("Enter a number: "))
y = int(input("Enter a number: "))
except ValueError:
return None
else:
a = float(x)
b = float(y)
return (a + b)
# def add(x, y):
#try:
# a = int(x)
# b = int(y)
# a = int(input("Enter a number: "))
# b = int(input("Enter a number: "))
# except ValueError:
# return None
# else:
# c = float(x)
# d = float(y)
#return (c + d)
2 Answers
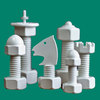
Steven Parker
231,269 PointsYou won't need input statements, the arguments are passed in to the function.
But you'll need to perform the float conversions in the "try" block for it to be able to catch the exceptions.

Pat Dudar
2,490 PointsAlright, well I already tried that and it didn't work out. I tested it out in a separate debugger and there didn't seem to be any errors..strange. Thanks for your help, man!
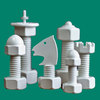
Steven Parker
231,269 PointsPerhaps there's more to it than just the placement of the float functions. Show the complete code again with the changes made.
Pat Dudar
2,490 PointsPat Dudar
2,490 PointsI see what you mean, Steven. However, the questions is asking to put a try: block before the float conversions are done. I give it a shot with the float conversions before and after the try: block and it didn't work out..maybe I'm missing something important in the try: block (I don't think I am though). Below is the question:
You're doing great! Just one more task but it's a bigger one. Right now, we turn everything into a float. That's great so long as we're getting numbers or numbers as a string. We should handle cases where we get a non-number, though. Add a try block before where you turn your arguments into floats. Then add an except to catch the possible ValueError. Inside the except block, return None. If you're following the structure from the videos, add an else: for your final return of the added floats.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsWhen they say "Add a try block before...", what they mean is "Add a try block that starts before...".
So the "try" will come before the float conversions, but the conversions themselves will be within the block established by the "try".