Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial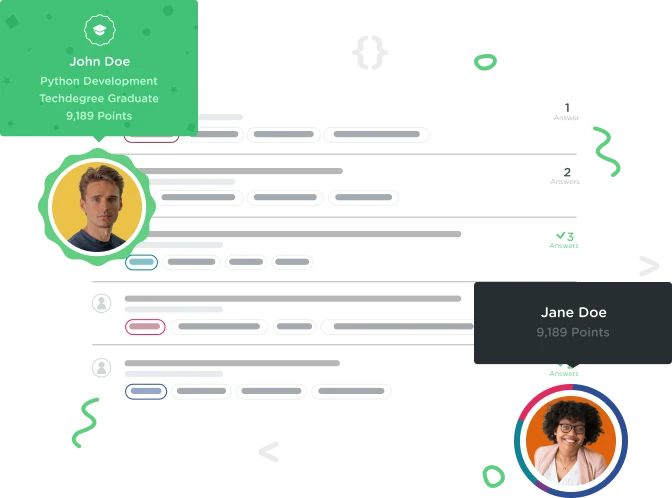

Travis Jewell
10,381 PointsTry Block - Continue with Index Input?
I want add the try block and if the exception occurs, have the program continue at the point where it asks the user to input the index. I've tried a bunch of different ways but I haven't been able to figure this out. The program always reverts back to the point where the user is asked to enter more shopping items. Is there a concise way to accomplish this?
shopping_list = []
def show_help():
print("\nSeparate each item with a comma.")
print("Type DONE to quit, SHOW to see the current list, and HELP to get this message.")
def show_list():
count = 1
for item in shopping_list:
print("{}: {}".format(count, item))
count += 1
print("Give me a list of things you wish to shop for.")
show_help()
while True:
new_stuff = input("> ")
if new_stuff == "DONE":
print("\nHere is your list:")
show_list()
break
elif new_stuff == "HELP":
show_help()
continue
elif new_stuff == "SHOW":
show_list()
continue
else:
new_list = new_stuff.split(",")
index = input("Currently {} items in the list. Add this at a certain spot? "
"Press enter for the end of the list, or give me a number: ".format(
len(shopping_list)))
if index:
while index:
try:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
break
except ValueError:
index = input("{} is not a number. Please try again. "
"\nEnter a valid number "
"or press enter for end of the list: ".format(index))
if index:
continue
else:
for item in new_list:
shopping_list.append(item.strip())
break
else:
for item in new_list:
shopping_list.append(item.strip())
4 Answers
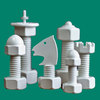
Steven Parker
231,275 PointsThis code seems incomplete. There are some break and continue statements in it, but no visible loop. It would help to include the complete code. Or better yet, make a snapshot of the workspace and provide the link to it.
But the basic idea is you need a loop that begins right before where the user inputs the index and ends after the except block. In the except block you will continue, but when the try succeeds you will break out of the loop.

Tessa Sullivan
1,517 PointsI wanted to repeatedly ask the user for a number if they entered in anything but an integer or nothing (i.e., just hit enter). Here's how I did it:
if index:
# Set the spot empty to trigger the while loop
# while spot is not an integer, ask them to enter a valid number (or enter)
spot = ''
while type(spot) is not int:
try:
spot = int(index) - 1
except ValueError:
index = input("{} is not a number. "
"Please re-enter the item number or press enter for end of list.".format(index))
# If user hits 'enter', then we set spot to the end of the list and break out of the while loop
if index == '':
spot = len(shopping_list)
break
# Now we insert the items at the entered (or calculated) spot
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
else: # if user did not enter a number, just append the new list to the end of the current list
for item in new_list:
shopping_list.append(item.strip())

Freddy Venegas
1,226 PointsTravis Jewell Could you share your solution?

Travis Jewell
10,381 PointsOk, I think I've got it all figured out now! If the user enters an invalid number, it asks for a valid number. If the user then enters a valid number, it will index accordingly, but if the user just presses enter, it will add the words to the end of the list.
If you notice any problems, let me know!

Timothy Recker
12,086 PointsI struggled for a while trying to accomplish the same thing by myself. Looking at this thread and following Steven Parker's advice helped me, and I thought I'd go ahead and post the code I ended up with in case it would be helpful to someone else (note: the code I started with is a copy of Kenneth's, with the exception of some additional printed text and lines I added to make the feedback more appealing to me):
else:
new_list = new_stuff.split(",")
while True:
index = input("""
Add this at a certain spot? Press enter for the end of the list,
or give me a number. Currently {} items in the list.
> """.format(len(shopping_list)))
if index:
try:
spot = int(index) - 1
for item in new_list:
shopping_list.insert(spot, item.strip())
spot += 1
print("")
print("Item(s) added!")
print("")
break
except ValueError:
print("")
print("{} is not a number. Please try again: ".format(index))
continue
else:
for item in new_list:
shopping_list.append(item.strip())
print("")
print("Item(s) added!")
print("")
break
Key additions include:
- a "while True:" loop in the line before "index = input(..."
- a "try:" in the line before "spot = int(index) - 1"
- an "except ValueError:" at the same indentations as the "try:" above that prints an error message
- "break" at the end of any successful input for index (i.e. an integer or an empty string)
- "continue" at the end of an invalid input for index (i.e. a non-integer)
Travis Jewell
10,381 PointsTravis Jewell
10,381 PointsI figured it out. Thank you so much for your help!