Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial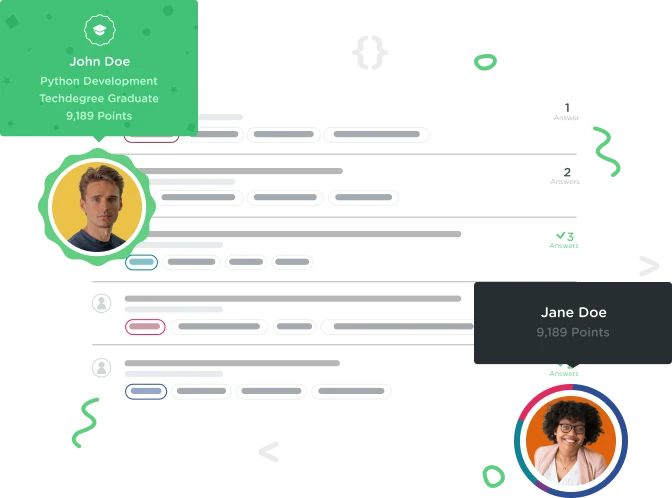

Ankit Biswas
280 Pointstry catch
Add input validation to the below program by printing "You must enter a whole number." if the user enters a decimal or something that isnβt a number.
Wrap all of the code contained in the Main method in a try/catch block The catch block should catch FormatException exceptions Inside of the catch block, output to the console the message "You must enter a whole number."?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
try
{
int count = int.Parse(input);
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
catch(Format Exception)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
}
}
}
2 Answers
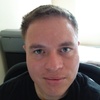
Pierre Gadea
4,542 PointsI think your problem is this line "catch(Format Exception)", should be catch(FormatException exception). This first word is the type of the exception and the second word is whatever name you want to give it. The int.Parse is the only place in your code that could throw a FormatException so you should not need the "continue" statement in there because if int.Parse() throws an exception it will never reach your while loop.
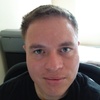
Pierre Gadea
4,542 Points try {
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
int count = int.Parse(input);
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
catch (FormatException exception)
{
Console.WriteLine("You must enter a whole number.");
}
Ankit Biswas
280 PointsAnkit Biswas
280 PointsIt doesn't work please tell me the full code.