Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial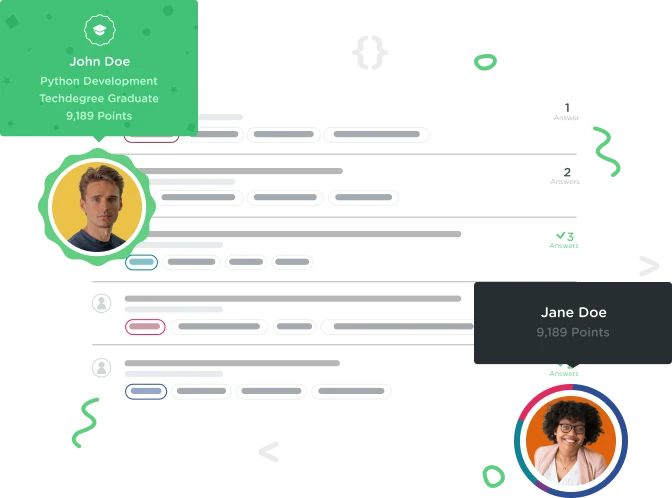

Ankit Biswas
280 PointsTry Catch and if else
Add more input validation to the program by printing "You must enter a positive number." if the user enters a negative number.
Add an if/else conditional statement just after parsing the user's provided input to an integer Write a conditional expression to ensure that the remainder of the code in the try block is only executed if the user provides a non-negative number If the user enters a negative number, output to the console the message "You must enter a positive number." I do the program but hot this error I entered 2.5. I expected "You must enter a whole number.", but got "You must enter a positive number." instead. I have to do both so please help me with that.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
try
{
int count = int.Parse(input);
if (count > 0)
{
int i = 0;
while (i < count)
{
i += 1;
Console.WriteLine("Yay!");
Console.ReadKey();
}
}
}
catch (FormatException)
{
Console.WriteLine("You must enter a positive number.");
}
}
}
}
1 Answer
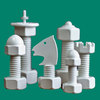
Steven Parker
231,269 PointsThe "try" and "catch" are for detecting if something other than a whole number is entered (causing the "Parse" to fail). That code should be left as it was after the first task.
The detection of a negative number can be done with an "if" (or "if/else") conditional.