Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial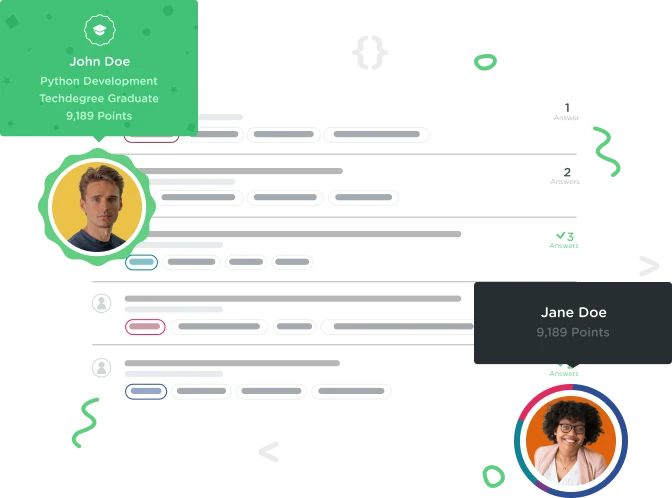

Timothy James-Hammond
1,641 PointsTry catch block does not seem to be functioning - still getting the "hard quit" and exception error message from class.
I dont seen the message in the try/catch block. (try/catch does not seem to fire) Cant seem to figure it.... and help would be ace!
Class code
public void load(int pezAmount){ int newAmount = mPezCount + pezAmount; if (newAmount > MAX_PEZ){ throw new IllegalArgumentException("Too many PEZ"); } mPezCount = newAmount; }
Example code
try{ dispenser.load(400); System.out.println("this will never happen!"); }catch (IllegalArgumentException iae){ System.out.println("Whoa there!"); System.out.printf("There was a %s", iae.getMessage()); }
Output
java.lang.IllegalArgumentException: Too many PEZ
Strange? or am i making a schoolboy error? thanks
4 Answers

Gavin Ralston
28,770 PointsThe snippets you're showing look okay. Maybe posting the full code would help?
Before you do that, though, check and make sure the Example.java file was saved. It looks like the try/catch block is added right at the end of the video, so while everything else appears to be going okay, that last little bit, if the file wasn't saved yet, means that you're still compiling the code that doesn't catch the error and handle it.

Timothy James-Hammond
1,641 PointsHi there, I have done some more delving into my code bug and the exception and hard quit is being triggered by the load() method where it defaults back to and calls load(MAX_PEZ).
here is the full code. I cant work out why it is trowing an error (but if you comment out the line in the load() method "load(MAX_PEZ);" then the try catch works and it does not hard quit.
Perhaps I should mention I have changed the name to PezDispenser4 and I am running and compiling in BlueJ (but surely this will not make a difference).
Any help would me awesome.
PezDispenser4 (class)
public class PezDispenser4 {
//m = member variable
private String mCharacterName;
private int mPezCount;
public static final int MAX_PEZ = 12;
//caps means constant value
//static means variable accesible anywhere
//final mean value of variable cant me changed
//constructor
public PezDispenser4(String selectedName){
mCharacterName = selectedName;
mPezCount = MAX_PEZ;
}
public boolean dispense(){
//set default value if there is nothing to dispense = false
boolean wasDispensed = false;
if(!isEmpty()){
mPezCount--;
wasDispensed = true;
}
return wasDispensed;
}
//helper method to check to see if pez machine is empty
public boolean isEmpty(){
return mPezCount == 0;
//is the pez count equal to 0? method returns a true/false
}
//method to load pez
public void load(){
load(MAX_PEZ);
//use other method and load it with constant to give it max
}
//method for loading chosen number of pez
public void load(int pezAmount){
int newAmount = mPezCount + pezAmount;
if (newAmount > MAX_PEZ){
throw new IllegalArgumentException("Too many PEZ");
}
//so this is safe to raise the amount by what was added as
//this will never happen if the amount loaded exceeds the max_pez
mPezCount = newAmount;
}
//properties
public String getCharacterName(){
return mCharacterName;
}
}
Exceptions (instance)
public class Exceptions {
public static void main(String[] args){
// Your amazing code goes here...
System.out.println("We are a making a new Pez Dispenser.");
PezDispenser4 dispenser = new PezDispenser4("yoda");
System.out.printf("The dispenser character is %s\n",
dispenser.getCharacterName());
//true
if(dispenser.isEmpty()){
System.out.println("The pez machine is empty");
}
//call load method to load more pezes
System.out.println("Loading more pezes...");
dispenser.load();
//check to see if not empty (! means not)
if(!dispenser.isEmpty()){
System.out.println("Now fully loaded");
}
while(dispenser.dispense()){
System.out.println("Chomp!");
}
if (dispenser.isEmpty()){
System.out.println("You ate all the Pez");
}
dispenser.load(4);
dispenser.load(2);
while(dispenser.dispense()){
System.out.println("Chomp!");
}
//dispenser.load(400);
//System.out.println("this will never happen!");
//try and catch block
try{
dispenser.load(400);
System.out.println("this will never happen!");
}catch (IllegalArgumentException iae){
System.out.println("Whoa there!");
System.out.printf("There was a %s", iae.getMessage());
}
}
}
Output (error log)
We are a making a new Pez Dispenser. The dispenser character is yoda Loading more pezes...```
Error log
java.lang.IllegalArgumentException: Too many PEZ at PezDispenser4.load(PezDispenser4.java:54) at PezDispenser4.load(PezDispenser4.java:45) at Exceptions.main(Exceptions.java:16)

Timothy James-Hammond
1,641 PointsOK in the spirit of helpfulness - I think I have found the answer to my logic error myself. By calling load(); when the PezDispenser was not empty I was overfilling the dispenser and thus getting the 'hard quit' as the code was never executing down to try and catch block. So maybe (in theory) the load(); would need to be in a try and catch block? and/or code in the class (load()) to deal with overfilling. I think I complicated this way too much - thats overthinking something for you! Cheers

Zeljko Porobija
11,491 PointsI see the problem here, in your constructor function
public PezDispenser4(String selectedName){
mCharacterName = selectedName;
mPezCount = MAX_PEZ;
}
Actually, the last line should go mPezCount = 0;